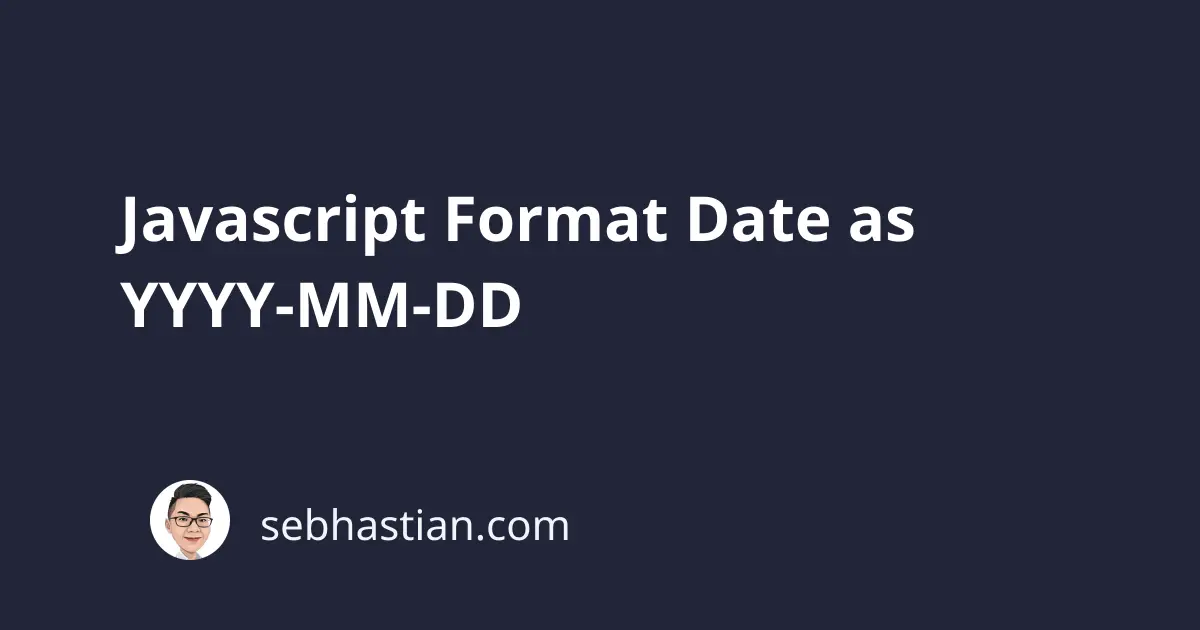
To format a date as a YYYY-MM-DD string, you need to use the toISOString()
method which converts a Date object to the ISO 8601 format.
The following example shows how to convert ‘August 30, 2023’ to ‘2023-08-30’:
let myDate = new Date('August 30, 2023');
console.log(myDate); // Wed Aug 30 2023 00:00:00 GMT+0400
let isoString = myDate.toISOString();
console.log(isoString); // 2023-08-29T20:00:00.000Z
Here, you can see that the toISOString()
method converts the Date object into a string in YYYY-MM-DD format.
But since the toISOString()
considers the time zone difference and my current time zone is GMT+4, the returned string goes back one day to 29th August to get the UTC time zone.
To avoid going back or forward one day because of time zone difference, you need to use the getTime()
and getTimezoneOffset()
method to add the time zone difference to your date:
let myDate = new Date('August 30, 2023');
console.log(myDate); // Wed Aug 30 2023 00:00:00 GMT+0400
let offset = myDate.getTimezoneOffset() * 60 * 1000;
// Create a new date without the offset
let dateWithOffset = new Date(myDate.getTime() - offset);
console.log(dateWithOffset); // Wed Aug 30 2023 04:00:00 GMT+0400
// Create the ISO string
let isoString = dateWithOffset.toISOString();
console.log(isoString); // 2023-08-30T00:00:00.000Z
The getTimezoneOffset()
counts the time zone difference in minutes, and it returns a negative number for positive GMT time zone difference, and vice versa.
This means GMT+4 returns -240, and we multiply it by 60 * 1000 to convert it to milliseconds.
Next, we create a new Date object, using the myDate
object which is converted to milliseconds with the getTime()
method. The offset
is added to the calculation.
When calling the toISOString()
method, JavaScript adds or subtracts time from the date to get the UTC time, returning the same time as the original myDate
object.
Now that you have the string in YYYY-MM-DD format, the last thing you need to do is remove the time part from the string.
You can easily do this using the split()
method as follows:
let myDate = new Date('August 30, 2023');
console.log(myDate); // Wed Aug 30 2023 00:00:00 GMT+0400
let offset = myDate.getTimezoneOffset() * 60 * 1000;
// Create a new date without the offset
let dateWithOffset = new Date(myDate.getTime() - offset);
console.log(dateWithOffset); // Wed Aug 30 2023 04:00:00 GMT+0400
// Create the ISO string
let isoString = dateWithOffset.toISOString();
console.log(isoString); // 2023-08-30T00:00:00.000Z
// Split the ISO string to remove time format
isoString = isoString.split('T')[0];
console.log(isoString); // 2023-08-30
And now you get the ISO string from the Date object. Here are other articles related to JavaScript date manipulation that you might find useful:
- Javascript Convert ISO String to a Date object
- Javascript Convert Milliseconds to a Date
- and Javascript Convert ISO Date to a Timestamp
These tutorials should come in handy when you work with JavaScript Date objects. Make sure to save it for later.
Happy coding!