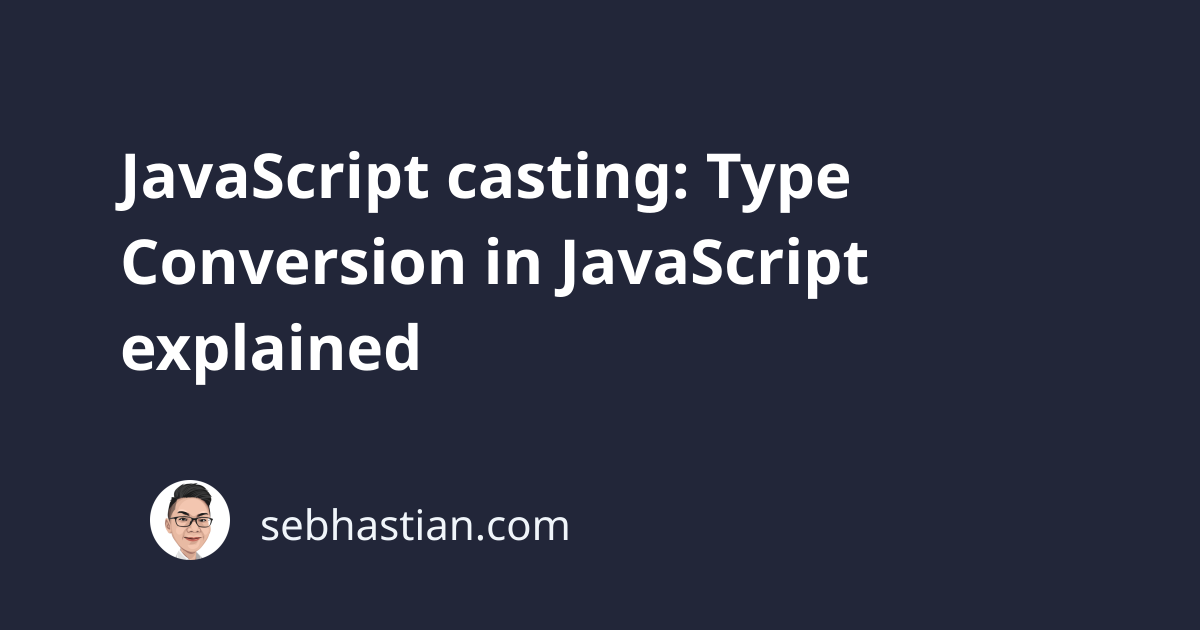
In programming language, casting is a way of telling the compiler to change an expression or value from one type to another. At times, you may want to convert your JavaScript expressions or values from one type to another.
For example, you may want to convert a value of string
type to number
so that you can perform mathematical operations on the value. You may also want to convert a data of boolean
type into a string
type. JavaScript provides you with built-in global functions and methods to convert data from one type to another
In this tutorial, we’ll look at the following ways of casting type in JavaScript:
- Casting data to
string
type - Casting data to
number
type - Casting data to
boolean
type
Let’s start with learning how to cast other types into string
type first.
Casting data to string type
Number type to string type
To convert any type of data to string
type, you need to use the built-in global function String()
. For example, here’s how you convert a number
type to a string
type. Notice the log from the typeof
operator call:
let str = String(101);
console.log(str); // "101"
console.log(typeof str); // "string"
Alternatively, you can also use the Number.toString()
method to convert a number to string. It works just the same as the global String()
function:
let str = (101).toString();
console.log(str); // "101"
console.log(typeof str); // "string"
Boolean type to string type
Next, you can also convert boolean
type into string
by using the global String()
function or the Boolean.toString()
method as follows:
let strOne = String(true);
console.log(strOne, typeof strOne); // "true" "string"
let strTwo = (true).toString();
console.log(strTwo, typeof strTwo); // "true" "string"
Date type to string type
You can even convert a value of Date
object, which holds date values into string with the same global function String()
and the Date.toString()
method:
let strOne = String(new Date("2021-04-06"));
console.log(strOne, typeof strOne);
// "Tue Apr 06 2021 00:00:00 GMT+0000 (Greenwich Mean Time)" "string"
let strTwo = (new Date("2021-03-05")).toString();
console.log(strTwo, typeof strTwo);
// "Tue Apr 06 2021 00:00:00 GMT+0000 (Greenwich Mean Time)" "string"
Special falsy types to string type
There are also special falsy type that you can convert to string only by using the String()
global function, such as null
, undefined
, and NaN
:
String(null); // "null"
String(undefined); // "undefined"
String(NaN); // "NaN"
Let’s look at converting other data to number
type next.
Casting data to number type
To cast a string into number type, you can use either the global function Number()
:
let num = Number("202");
console.log(num, typeof num); // 202 "number"
You can also convert other types like boolean
with the Number()
function. true
value will be converted as 1
and false
value will be converted as 0
:
let numTrue = Number(true);
console.log(numTrue); // 1
let numFalse = Number(false);
console.log(numFalse); // 0
Finally, you can also convert a string
that represents a floating number into number
type using Number()
function:
let num = Number("7.6");
console.log(num); // 7.6
And that’s all about casting other types to number
type in JavaScript. Let’s take a look at casting to boolean
type next.
Casting other types to boolean type
The global function Boolean()
allows you to convert any other data type into boolean
type. Here are some examples of converting a string
and a number
into boolean
:
let boolStr = Boolean("string");
console.log(boolStr); // true
let boolNum = Boolean(9);
console.log(boolNum); // true
Any truthy values will be converted to true
while falsy values will be converted into false
.
See also: JavaScript truthy / falsy values explained
Conclusion
And now you’ve learned how JavaScript type casting works. The global function String()
, Number()
, and Boolean()
enable you to convert your data from one type to another easily.
Also, since JavaScript is a weakly-typed language, so it also has a type coercion feature that automatically converts data from one type to another when the situation requires it.