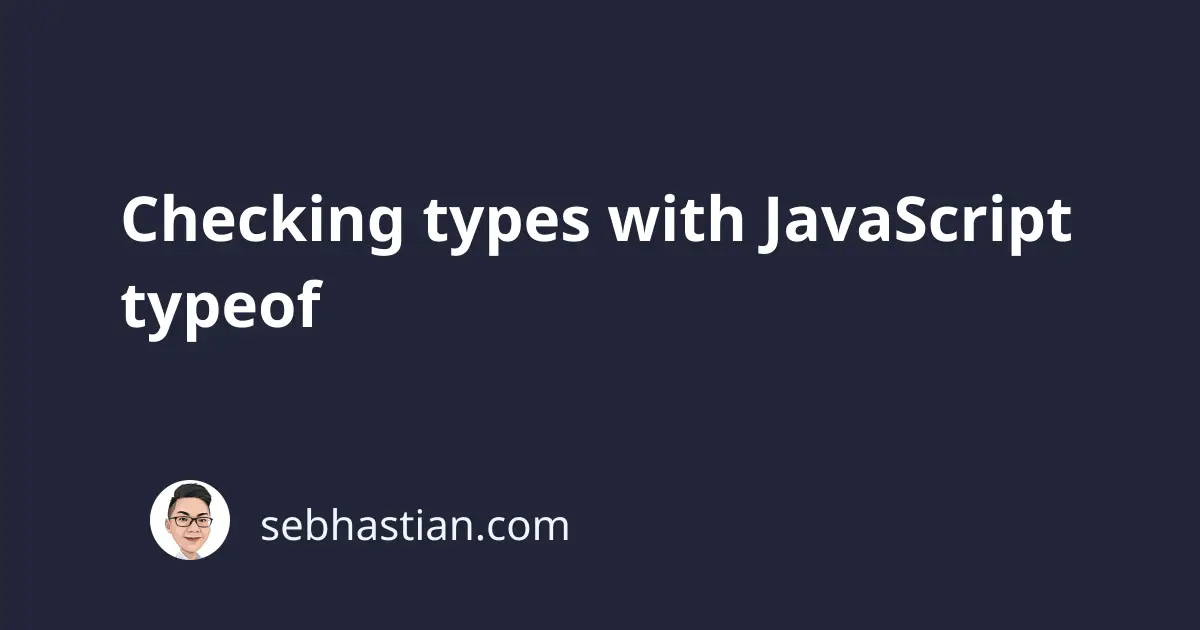
typeof
is a JavaScript operator that returns the data type of the value you passed as the argument. The data type returned will be of string
type.
let x = "Hello";
console.log(typeof x); // "string"
JavaScript is a weakly-typed language that allows you to change the data type of your variable after it has been declared:
let x = "Hello";
x = 12; // string is changed to number
In a strongly-typed language like Java, the code above will crash the program and outputs an error. But not so with JavaScript.
This is why the typeof
operator is very useful to help you check your variables type as you code your application.
For example, suppose you want to perform an addition between two number variables, but one of them is mistakenly written as a string
:
let x = 10;
let y = "10";
console.log(x + y); // "1010"
When you add the variables above, it will return “1010” of string
type. This is because JavaScript doesn’t understand how to add a number
into a string
, but instead of crashing your program, it silently converts the x
variable value into a string
and concatenate both variables.
To handle this kind of bug, you can use a combination of a try..catch
block and an if
statement to check on the type of the variables and throw
an error when they are not a number
.
let x = 10;
let y = "10";
try {
if (typeof x !== "number") {
throw "Error: The variable x is not a number";
}
if (typeof y !== "number") {
throw "Error: The variable y is not a number";
}
} catch (err) {
console.error(err);
return;
}
console.log(x + y);
However, keep in mind that the value NaN
in JavaScript also returns a number
, so you also need to check if the value is NaN with isNaN()
function:
let y = NaN;
try {
if (typeof x !== "number" || isNaN(x)) {
throw "Error: The variable x is not a number";
}
if (typeof y !== "number" || isNaN(y)) {
throw "Error: The variable y is not a number";
}
} catch (err) {
console.error(err);
return;
}
console.log(x + y);
Finally, here’s the return value of typeof
for your reference:
argument value | typeof return |
---|---|
"ABC" | string |
123 | number |
true | boolean |
false | boolean |
function myFn(){} | function |
[1,2,3] | object |
{age: 8} | object |
null | object |
undefined | undefined |