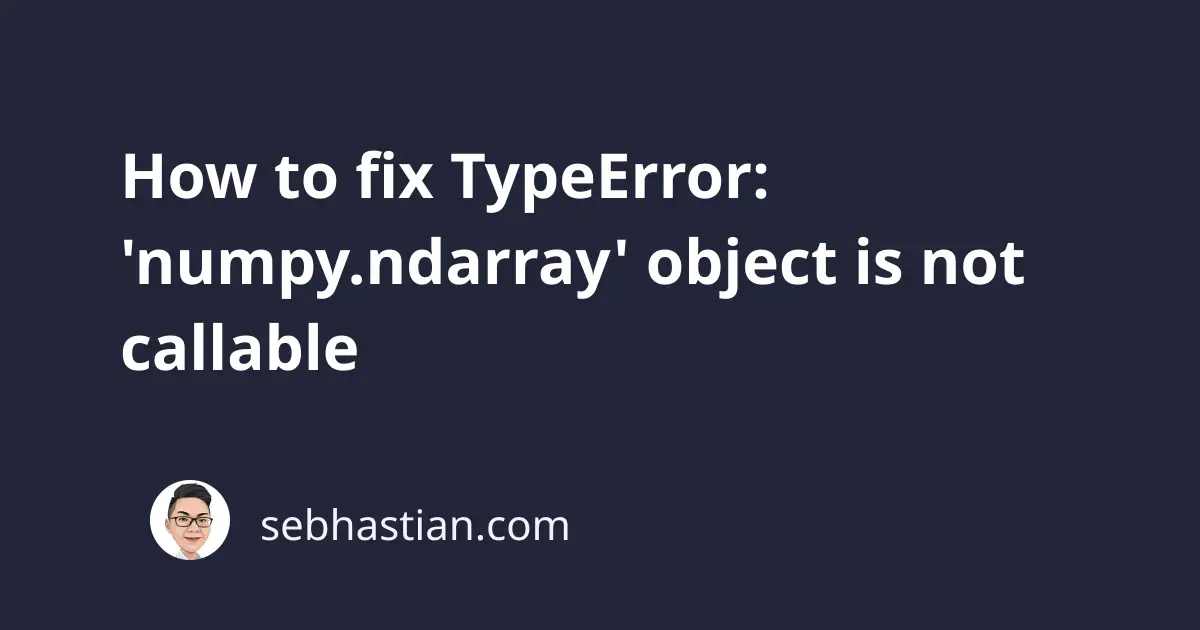
While working with NumPy in Python, you might get the following error:
TypeError: 'numpy.ndarray' object is not callable
This error usually occurs when you try to call a NumPy array like a function by using round brackets.
The following tutorial shows examples that cause this error and how to fix it.
How you get this error
Suppose you defined a NumPy array in your source code as follows:
import numpy as np
res = np.array([4, 5, 2, 3, 5, 4, 4, 9])
Next, you try to access the first element of the array at index 0
like this:
x = res(0)
You get the following error:
Traceback (most recent call last):
File "main.py", line 4, in <module>
x = res(0)
TypeError: 'numpy.ndarray' object is not callable
In the code, you used round brackets (0)
to access the first element in the array, which causes Python to think you’re trying to call the res
variable like a function.
Since res
is an array and not a function, you receive this error.
How to fix this error
To resolve this error, you need to replace the round brackets with square brackets []
so that Python knows you’re trying to access an array element:
import numpy as np
res = np.array([4, 5, 2, 3, 5, 4, 4, 9])
x = res[0]
print(x) # 4
In the example above, we assigned the first element of the res
array to the x
variable without raising the error.
Using square brackets, you can access any valid element in the array:
# Sum the first and last element in the array
x = res[0] + res[-1]
print(x) # 13
Now you’ve learned how to fix TypeError: 'numpy.ndarray' object is not callable
that occurs when using NumPy in your source code.
Alternative cause: You have a function and an array with the same name
This error can also occur when you have a function and an array with the same name.
For example, suppose you declare a function and a variable named greet
as follows:
import numpy as np
def greet():
print("Hello World!")
greet = np.array(['John, Lisa'])
greet()
The greet
variable overwrites the greet()
function, causing you to call a NumPy array instead of a function.
To fix this error, you need to change the variable name:
import numpy as np
def greet():
print("Hello World!")
friends = np.array(['John, Lisa'])
greet()
The function greet
can now be called and the error is resolved.
More resources
The following tutorials explain how you can fix other common errors when using the NumPy library:
How to fix NameError: name ’np’ is not defined
Fix Python ’numpy.float64’ object is not iterable error
I hope the tutorials are helpful. See you in other articles! 👍