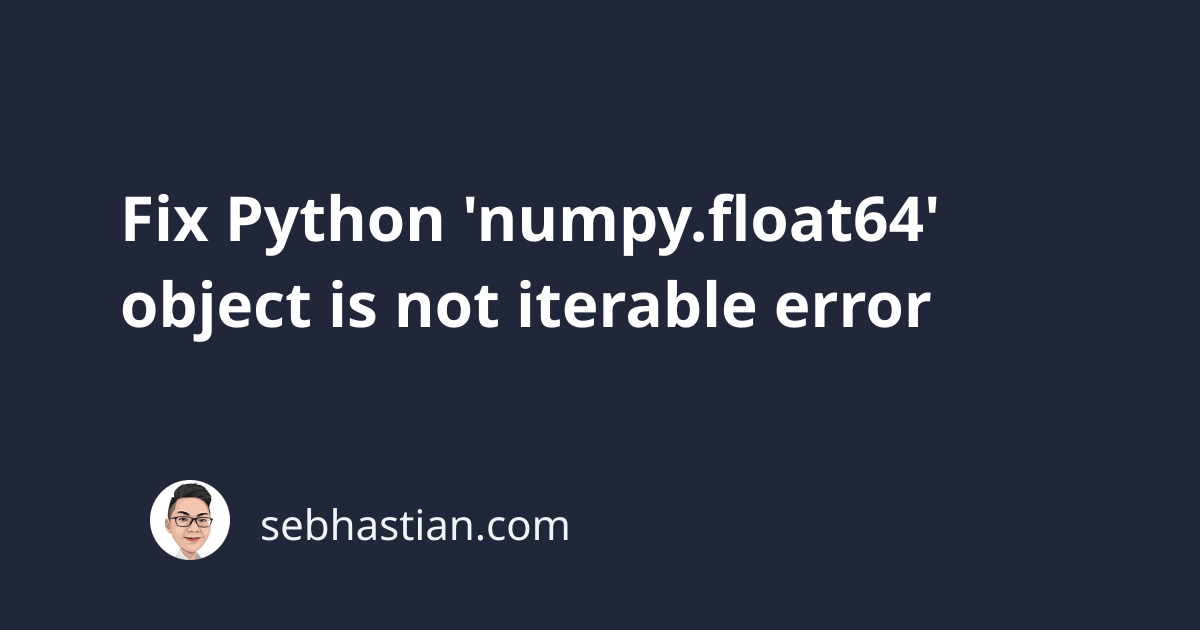
Python shows the TypeError: 'numpy.float64' object is not iterable
message when you try to iterate over a numpy.float64
object, which is not a sequence and therefore not iterable.
To solve this error, you need to make sure you’re not passing a float object where an iterable is expected. Continue reading to learn how.
Solution # 1: Make sure you are passing an iterable object
One common cause of this error is attempting to use the in
operator on a float value, as in the example below:
import numpy as np
score = np.float64(5.0)
# ❌ numpy.float64 object is not iterable
for i in score:
print(i)
The for
loop statement in the code above will cause the following error:
To fix the error, make sure you are passing an iterable to the for
loop.
For example, you can pass a NumPy array as shown below:
import numpy as np
scores = np.array([1.2, 3.4, 5.6, 7.8])
for i in scores: # ✅
print(i)
The Python code above works because you are passing a NumPy ndarray
object to the for
statement.
Solution #2: Do not pass a float to a function that requires an iterable
Another cause of this error is when you pass a numpy.float64
object to a function that requires an iterable.
For example, the Python min()
function requires that you pass an iterable so that it can determine the smallest value from a set of values.
If you pass a float to the function, Python responds with a TypeError
as follows:
import numpy as np
score = np.float64(5.0)
# ❌ TypeError: 'numpy.float64' object is not iterable
res = min(score)
You can’t pass a single numpy.float64
object to the min()
function, but you can pass an ndarray
object like this:
import numpy as np
scores = np.array([5.0])
res = min(scores) # ✅
print(res) # 5.0
As you can see, you can create an array with only a single value and pass it to a function that requires an iterable, such as min()
, max()
, sum()
, and list()
.
These functions can also be called if you have a multi-dimensional array as shown below:
import numpy as np
scores = np.array([[5.0, 6.0], [1.2, 9.1]])
for i in scores: # ✅
print(min(i)) # ✅
The for
statement works because you are passing an array object, and the min()
function also works because you are passing the nested arrays to the function.
The output will be:
5.0
1.2
The min()
function calculates the smallest value from the array you passed as its argument.
Conclusion
The numpy.float64 object is not iterable
error occurs when you attempt to iterate over a numpy.float64
object, which is not iterable.
The solution is to use a NumPy array to hold a sequence of float values, then pass that array to the function or construct that requires an iterable object.
Even when you have only a single float value, you need to pass it as an array as shown in this article.