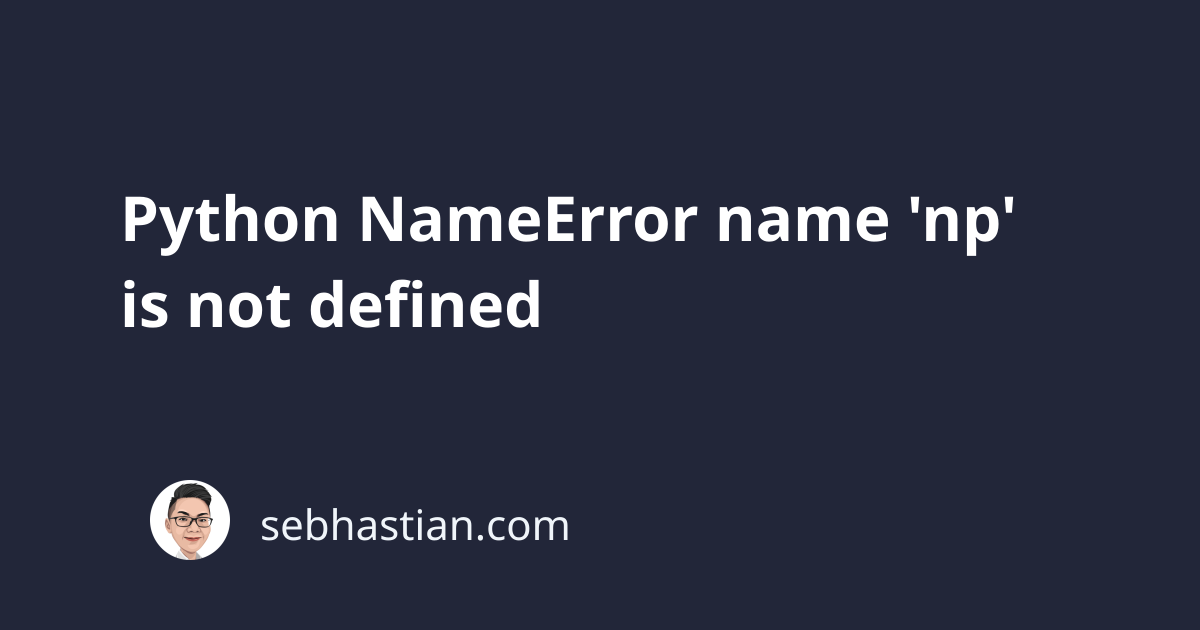
The NameError: name 'np' is not defined
is a common error that occurs when using the NumPy module in your code.
The stack trace is as follows:
Traceback (most recent call last):
File ...
data = np.array([1, 2, 3])
NameError: name 'np' is not defined
If you see a similar error, it means you didn’t alias the numpy
module as np
in the import
statement.
This article shows you the most common scenarios when this error may happen.
1. Using import numpy syntax
The most common import statement for numpy
is as follows:
import numpy as np
data = np.array([1, 2, 3])
The alias as np
isn’t required, but it’s nice to have as you don’t need to type numpy
when using NumPy’s functions and classes.
The NameError
is triggered when you import numpy
without an alias, but still type np
when you use its functions:
import numpy
data = np.array([1, 2, 3]) # ❌
data = numpy.array([1, 2, 3]) # ✅
The solution for this error depends on whether you want to use np
or numpy
in your code.
If you want to use np
, add as np
to the import numpy
statement.
Otherwise, replace all mentions of np
with numpy
in your code.
2. Using import * syntax
Sometimes, you may see starred import when importing the numpy
library as follows:
from numpy import *
The import *
syntax means you are importing the whole numpy
module into your code.
If you try to use the numpy module with np
name, you also get a NameError
:
from numpy import *
data = np.array([1, 2, 3]) # ❌
To fix this error, type the function directly as follows:
from numpy import *
data = array([1, 2, 3]) # ✅
Please note that it’s discouraged to use starred imports in Python because it can cause confusion.
Using regular or named imports makes it easy to identify which module is used because the module name is invoked.
Since you want to use the np
name, then the best fix is to use a named import as follows:
import numpy as np
data = np.array([1, 2, 3]) # ✅
Most developers do like to alias numpy as np
because it’s shorter and easier to type.
Now you’ve learned how to fix NameError: name 'np' is not defined
.
I hope this article was helpful. See you in other tutorials! 👋