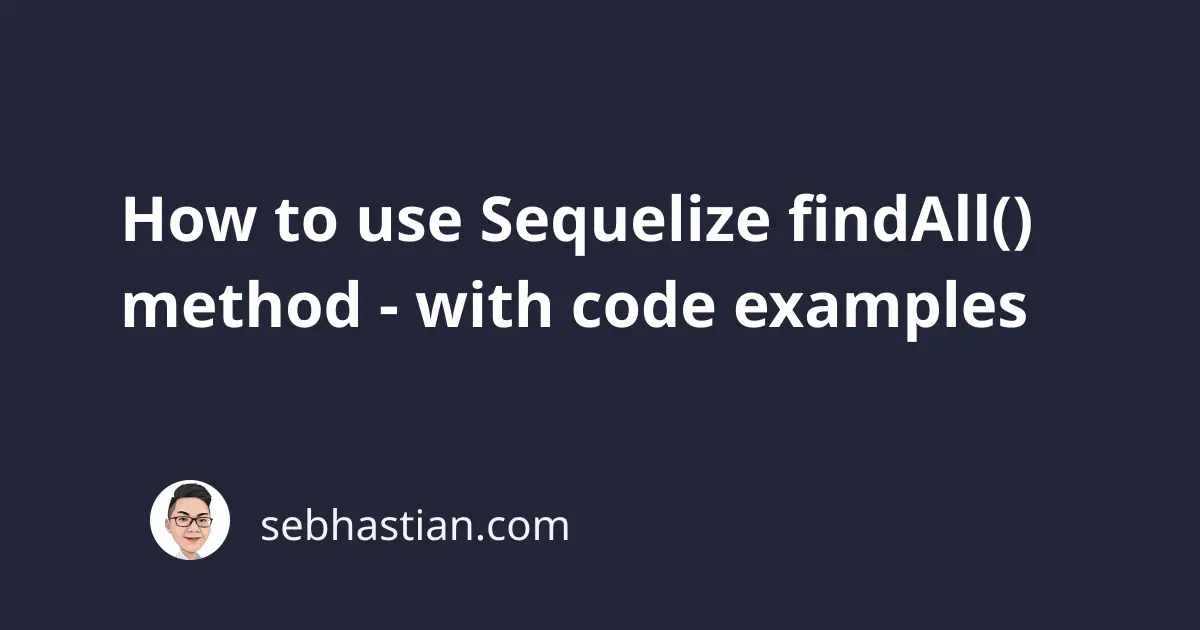
The Sequelize findAll()
method is used to query data from your SQL table to your JavaScript application. The method will return your table rows as an array of objects.
The findAll()
method can be called from a Model
that represents the table in your database.
Let’s see an example of the findAll()
method in action.
Suppose you have a database named Users
with the following data:
+----+-----------+----------+
| id | firstName | lastName |
+----+-----------+----------+
| 1 | Nathan | Doe |
| 2 | Joe | Doe |
| 3 | Jane | Doe |
+----+-----------+----------+
Before you query the table, you need to first create a Sequelize model that represents the table as follows:
const User = sequelize.define(
"User",
{
firstName: Sequelize.STRING,
lastName: Sequelize.STRING,
},
{
timestamps: false,
}
);
After that, you can use the User
model to query the table using the findAll()
method.
Here’s an example:
const user = await User.findAll();
console.log(JSON.stringify(user, null, 2));
The call to User.findAll()
method above will cause Sequelize to generate and execute the following statement:
SELECT
`id`,
`firstName`,
`lastName`
FROM
`Users` AS `User`;
You’ll see the following output from the console:
[
{
"id": 1,
"firstName": "Nathan",
"lastName": "Doe"
},
{
"id": 2,
"firstName": "Joe",
"lastName": "Doe"
},
{
"id": 3,
"firstName": "Jane",
"lastName": "Doe"
}
]
When you need to improve the query, you can add one or more options available in the findAll()
method.
For example, you can add the where
option to add the WHERE
clause to the query:
const user = await User.findAll({
where: { firstName: "Jane" }
});
You can also pass the limit
option to add the LIMIT
clause to the query:
const user = await User.findAll({
limit: 2,
where: { firstName: "Jane" },
});
Or you can change the sorting order by adding the order
option:
const user = await User.findAll({
order: [['id', 'DESC']]
});
// ORDER BY `User`.`id` DESC;
You can see the full options available for findAll()
method in Sequelize findAll() documentation
And that’s how you can use the findAll()
method in Sequelize. Besides the findAll()
method, Sequelize also provides:
- The
findOne()
method to retrieve exactly one row from your table - The
findByPk()
method to retrieve a row by its primary key value - The
findOrCreate()
method to retrieve matching rows or create one when there’s no matching row
You can use the method that fits your query requirements the best.