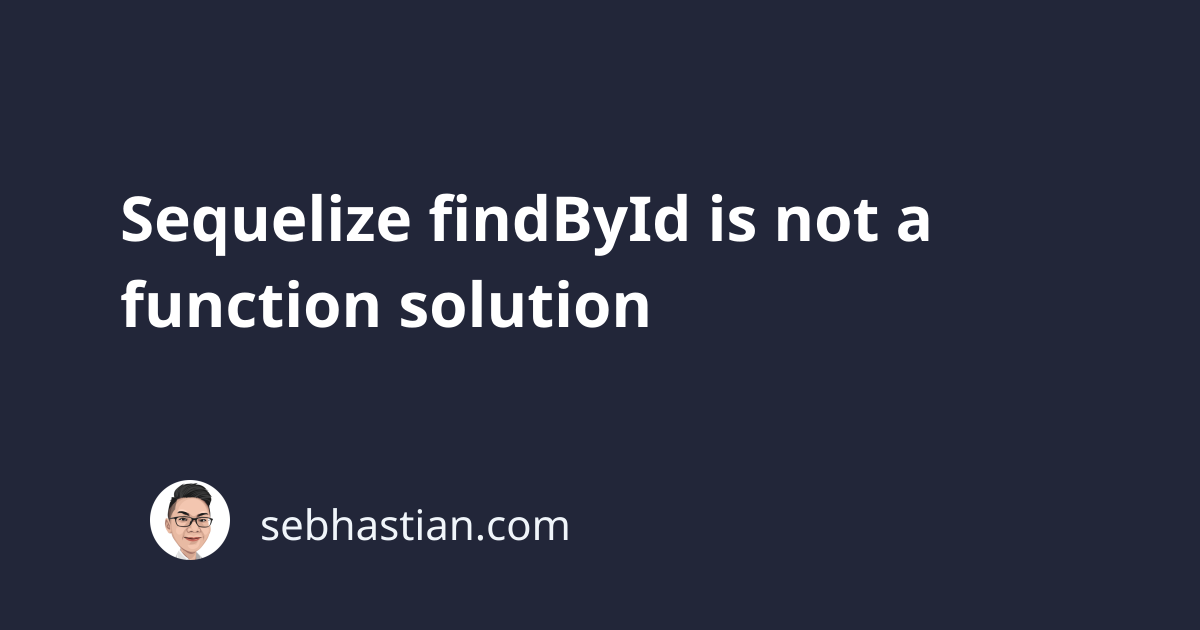
When you run a Sequelize findById()
method, JavaScript may throw an error saying findById
is not a function.
The following code searches for a user
data with an id
value of 2
:
const user = await User.findByPk(2);
But JavaScript throws an error when the code above is run:
const user = await User.findById(2)
^
TypeError: User.findById is not a function
This is because the findById()
method has been deprecated since Sequelize version 5.
As an alternative, Sequelize provides the findByPk()
method, which finds a data row that has the same primary key value as the one you passed as its argument.
Just replace findById
with findByPk
in your code and everything should work.
const user = await User.findByPk(2);
if (user === null) {
console.log("Not found!");
} else {
console.log(user);
}
Now you know how to fix the findById
is not a function error. Nice work! 👍