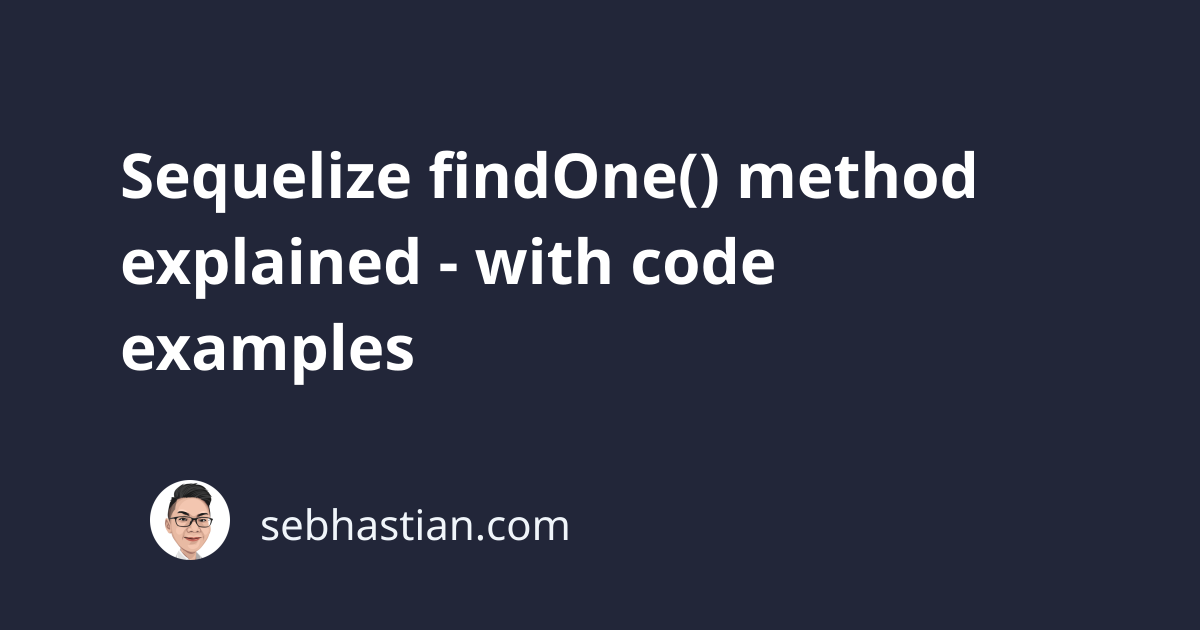
The Sequelize findOne()
method is used to retrieve exactly ONE row from all rows that match your SQL query.
The method is similar to the findAll()
method except that it adds the LIMIT 1
clause to the generated SQL query.
The method is a property of the Model
object. You need to create a Model
that represents your table first and then call the method from it.
For example, suppose you have a table of Users
with the following data:
+----+-----------+----------+
| id | firstName | lastName |
+----+-----------+----------+
| 1 | Nathan | Doe |
| 2 | Joe | Doe |
| 3 | Jane | Doe |
+----+-----------+----------+
First, you create a Sequelize model of the table using sequelize.define()
method:
const User = sequelize.define(
"User",
{
firstName: Sequelize.STRING,
lastName: Sequelize.STRING,
},
{
timestamps: false,
}
);
Next, call the findOne()
method and pass a where
condition as a filter:
const user = await User.findOne({
where: { lastName: "Doe" },
});
console.log(user.toJSON());
The findOne()
method will generate and execute the following SQL query. Note how the LIMIT 1
clause is added by the end of the statement:
SELECT
`id`,
`firstName`,
`lastName`
FROM
`Users` AS `User`
WHERE
`User`.`lastName` = 'Doe'
LIMIT 1;
The output of the user
log will be as follows:
{
id: 1, firstName: 'Nathan', lastName: 'Doe'
}
While the findAll()
method returns an array even when you have only one matching row, the findOne()
method always returns an Object
or null
when there’s no matching row.
And that’s how the findOne()
method works in Sequelize. You can see the list of valid findOne()
method options here.