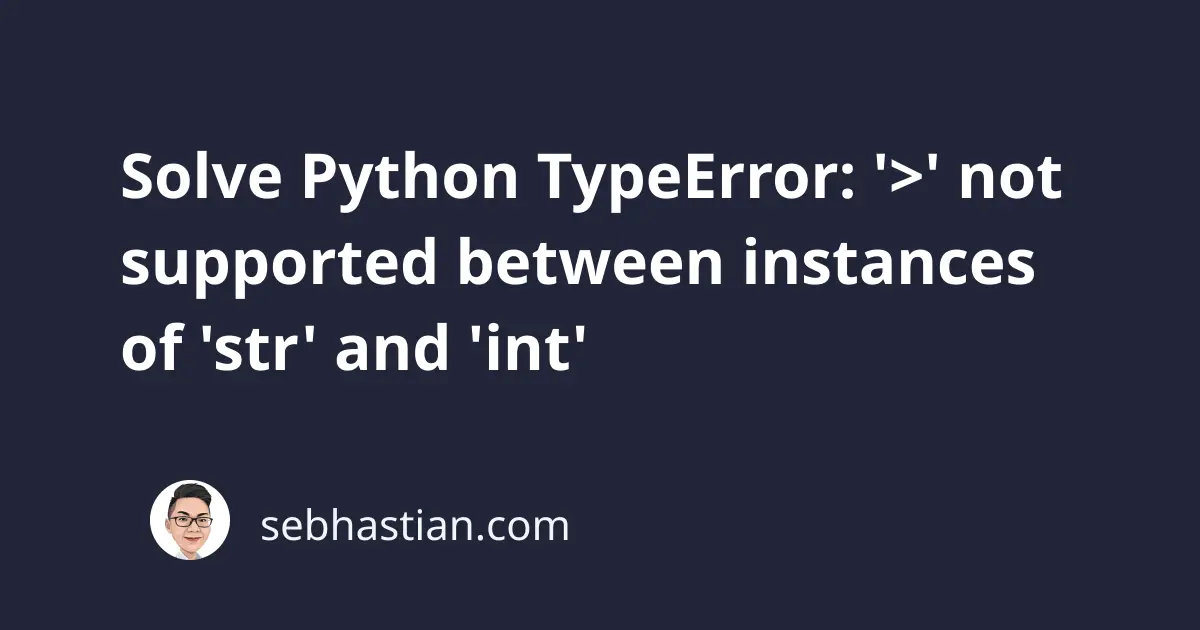
The Python TypeError: ‘>’ not supported between instances of ‘str’ and ‘int’ happens when you try to compare a string to an integer using the greater than operator (>
).
To resolve this error, you need to make sure the data being compared is of the same type.
The following code example shows this error:
result = "100" > 50 # ❗️TypeError
This is because Python doesn’t automatically convert values from one type to another.
When you compare a string with an integer as in the code above, Python will throw an error instead of trying to make the code work for you:
Traceback (most recent call last):
File "/script.py", line 1, in <module>
result = "100" > 50
^^^^^^^^^^
TypeError: '>' not supported between instances of 'str' and 'int'
This is different from other programming languages like JavaScript, where the implicit conversion allows you to compare values of different types.
To resolve this error, you must do one of the following before performing the comparison:
- Convert the string to an integer using the
int()
function - Convert the integer value to a string using the
str()
function.
See the example below:
result = int("100") > 50 # This will work
result = "100" >= str(50) # This will also work
You can compare the values above as integers or as strings.
When you compare them as strings, the values will be compared in lexicographic order, meaning they are compared based on alphabetical order.
Also, this error may occur when you call the max()
function on a list that has both integer and string data:
result = max([50, "100"]) # ❗️TypeError
The code above will produce the following output:
Traceback (most recent call last):
File "/script.py", line 1, in <module>
result = max([50, "100"])
^^^^^^^^^^^^^^^^
TypeError: '>' not supported between instances of 'str' and 'int'
To fix this error, you need to make sure all values passed to the max()
function are of the same type.
Conclusion
To conclude, the greater than operator >
can only be used to compare values of the same type.
If you try to compare values of different types, Python will raise a TypeError:
x = 100 # integer
y = "50" # string
if x > y: # ❗️TypeError
print("x is greater than y")
To resolve this error, you need to make sure the data being compared is of the same type.
There are two ways you can do this:
- Modify the values so they all have the same type
- Convert the values to have the same type before performing the comparison.
In addition to the greater than operator, this error can also occur when using other comparison operators such as the less than operator (<
) or the equal to operator (==
).
To avoid the same error with these operators, you need to pay attention to the type of data you are comparing.
Now you’ve learned how to fix Python TypeError: ‘>’ not supported between instances of ‘str’ and ‘int’ like a professional. Good work!