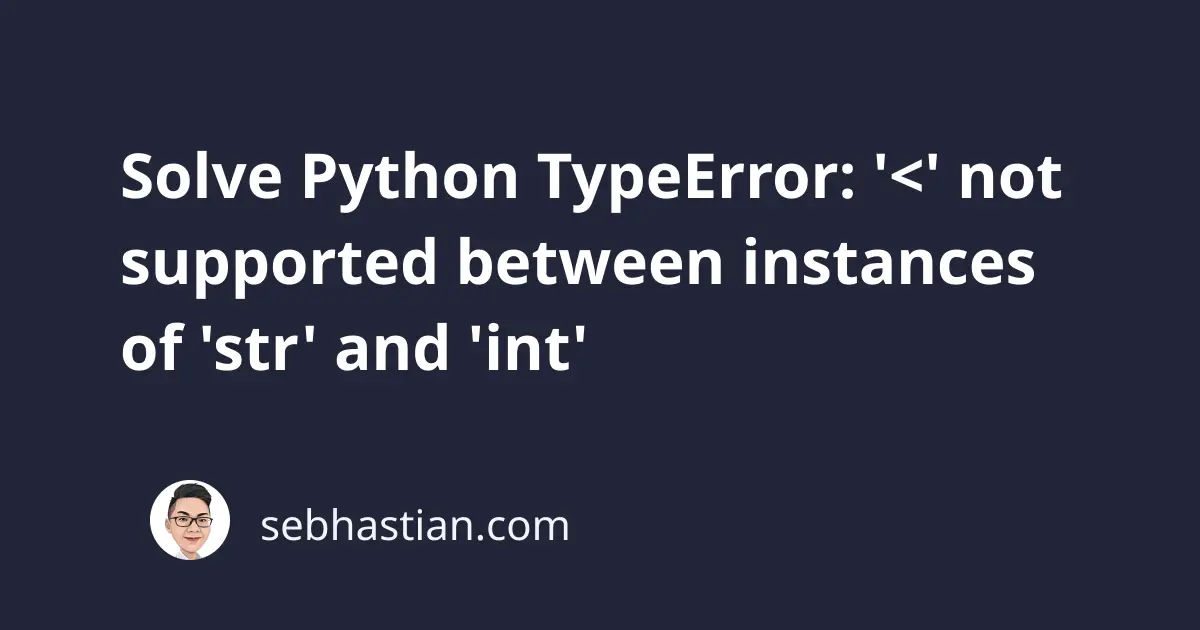
The TypeError: ‘<’ not supported between instances of ‘str’ and ‘int’ occurs when you try to compare a string and an integer using the less than operator (<
).
To resolve this error, you need to convert the string type data to integer before you compare them.
Here’s an example of how this TypeError occurs:
print("99" < 20) # ❗️TypeError
The number "99"
in the code above is actually a string because it’s enclosed in quotation marks.
Running the code will produce the following output:
Traceback (most recent call last):
File "/script.py", line 1, in <module>
print("99" < 20)
^^^^^^^^^
TypeError: '<' not supported between instances of 'str' and 'int'
In Python, strings and integers can’t be compared using comparison operators, such as the less than operator.
To compare the data above successfully, you need to convert the string type to int using the int()
function:
print(int("99") < 20) # False
The TypeError: ‘<’ not supported between instances of ‘str’ and ‘int’ error can occur in any situation where you try to compare a string and an integer using the less than operator.
Here are some scenarios when the error may be raised:
- When you try to compare the elements of a list or tuple that contain both strings and integers:
my_list = ["10", 5, "20", 10]
print(my_list[0] < my_list[1]) # ❗️TypeError
- When you call the
min()
function on a list that has both integer and string data:
list = [23, "999"]
result = min(list) # ❗️TypeError
- When you try to compare an integer input from a user with another integer:
age = input("Please enter your age: ")
if age < 18: # ❗️TypeError
print("You are not old enough to vote.")
The input()
function always returns a string even when you enter a number.
You need to convert the string type data to integer first before comparing it like this:
age = input("Please enter your age: ")
# 👇 Convert age to int to avoid TypeError
if int(age) < 18:
print("You are not old enough to vote.")
The above code will properly compare the age
input as an integer, avoiding the TypeError.
Conclusion
To conclude, the Python TypeError: ‘<’ not supported between instances of ‘str’ and ‘int’ occurs when you try to compare a string and an integer using the less than <
operator.
To resolve this error, you need to make sure that you are comparing values of the same type.
When you have values of different types, use the appropriate type conversion functions to convert the values to the same type before performing the comparison.
Now you’ve learned how to solve this error like a professional Python developer. Awesome!