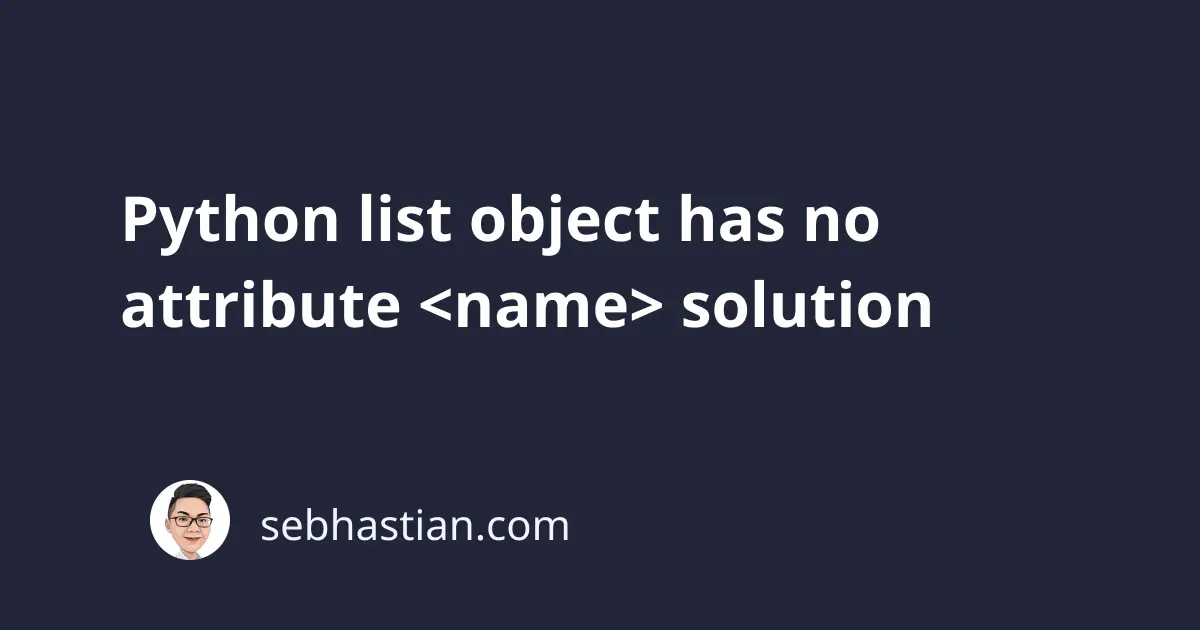
The Python list object has no attribute
error occurs whenever you try to access an attribute that’s not defined on the list object.
To fix this error, you need to make sure you are calling an attribute that exists on a list object.
This article will show you an example that causes this error, as well as how to avoid it in the future.
Fix Python AttributeError: ’list’ object has no attribute
Suppose you try to call the lower()
method on a list object as follows:
list = ["A", "C", "Z"]
list.lower()
You may expect that the lower()
method will transform all string data in the above list to lowercase.
But since the list object doesn’t have the lower()
method, Python will show the following error:
Traceback (most recent call last):
File ...
list.lower()
AttributeError: 'list' object has no attribute 'lower'
To see all attributes defined in a list object, you can call the dir()
function and print the result.
The following code:
list = ["A", "C", "Z"]
print(dir(list))
Prints the following result:
['__add__', '__class__', '__class_getitem__', '__contains__',
'__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__',
'__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__',
'__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__',
'__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__',
'__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__',
'__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__',
'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop',
'remove', 'reverse', 'sort']
The names that are not surrounded with underscores (__
) are methods that you can call from the list object.
As you can see it doesn’t show the lower
method because that method is defined in a string object.
To transform the string characters into lowercase, you can use a list comprehension to return a new list as follows:
list = ["A", "C", "Z"]
new_list = [item.lower() for item in list]
print(new_list) # ['a', 'c', 'z']
Conclusion
Python AttributeError: 'list' object has no attribute <name>
happens when you try to access an attribute that’s not defined in a list.
This article shows an example of the error with the lower()
method, but any method you call that doesn’t exist will also produce this error.
For example, calling the split()
or upper()
method also gives the same error.
To fix this error, you need to call the attributes that exist on a list. You can use the dir()
function to get all attributes of a list and print it out.
Now you know how to fix AttributeError: 'list' object has no attribute <name>
. Very nice! 👍