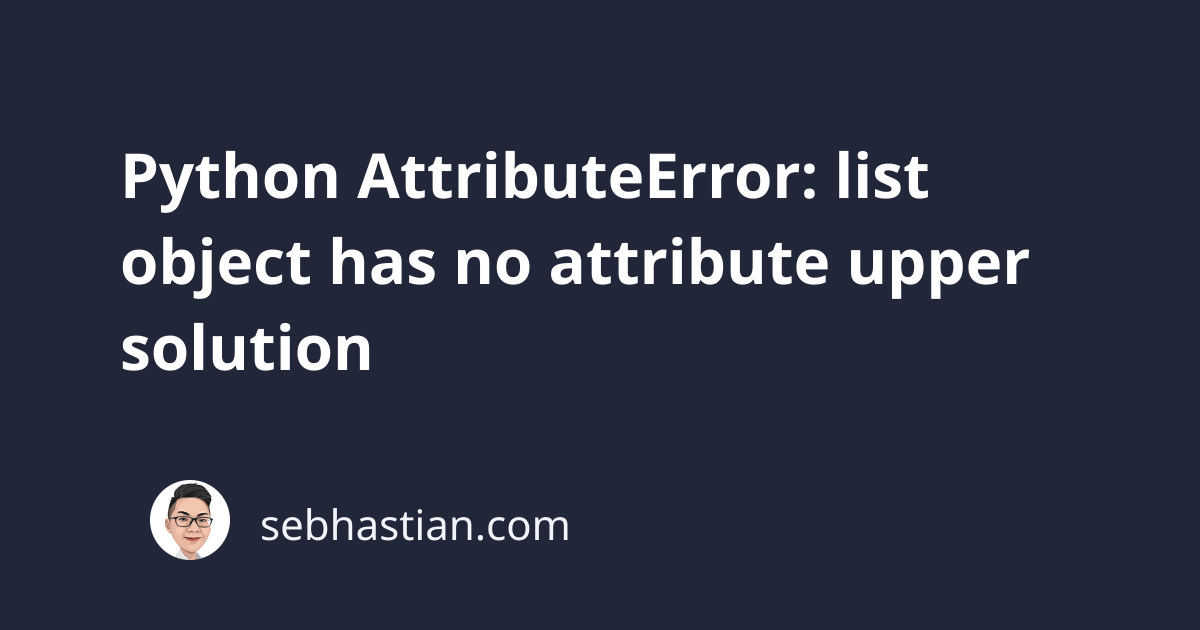
Python error AttributeError: list object has no attribute upper
occurs when you call the upper()
method on a list object.
To fix this error, you need to call the upper()
method from a string object.
Fix Python AttributeError: list object has no attribute upper
The list object doesn’t have the upper()
method because a list can contain a mixed type of data, such as a string, an integer, or a boolean.
Suppose you try to call the upper()
method on a list as follows:
words = ["hello", "there", "son"]
words.upper()
Python will respond with the following error message:
Traceback (most recent call last):
File ...
words.upper()
AttributeError: 'list' object has no attribute 'upper'
To fix this error, you need to call the upper()
method on the string object. This means you have to access the elements of your list.
You can use a list comprehension to do so:
words = ["hello", "there", "son"]
new_words = [item.upper() for item in words]
print(new_words) # ['HELLO', 'THERE', 'SON']
Alternatively, you can also use a for
loop to iterate over the list as follows:
words = ["hello", "there", "son"]
for i in range(len(words)):
words[i] = words[i].upper()
print(words) # ['HELLO', 'THERE', 'SON']
But I recommend you use the list comprehension syntax because it’s more concise.
Calling upper() on a list with non-string elements
Finally, when you have a list of mixed types, you need to check the type of each element before calling the str.upper()
method on it.
Consider the following example:
words = ["hello", 1, "there", True, "son"]
new_words = [
word.upper() if isinstance(word, str) else word for word in words
]
print(new_words)
# Output: ['HELLO', 1, 'THERE', True, 'SON']
The code above used a list comprehension to convert the string elements to uppercase.
This is done by checking if the element is an instance of str
using the isinstance()
function.
Conclusion
The Python AttributeError: list object has no attribute upper
is triggered when you call the upper()
method on a list object.
To fix this error, make sure you are calling the upper()
method only on string objects.
To access strings inside a list, you can use a list comprehension or a for
loop as shown in this tutorial.
To learn more about list object has no attribute
error, you can check my article Understanding Python list object has no attribute error.
Thanks for reading. Hope this tutorial helps! 👍