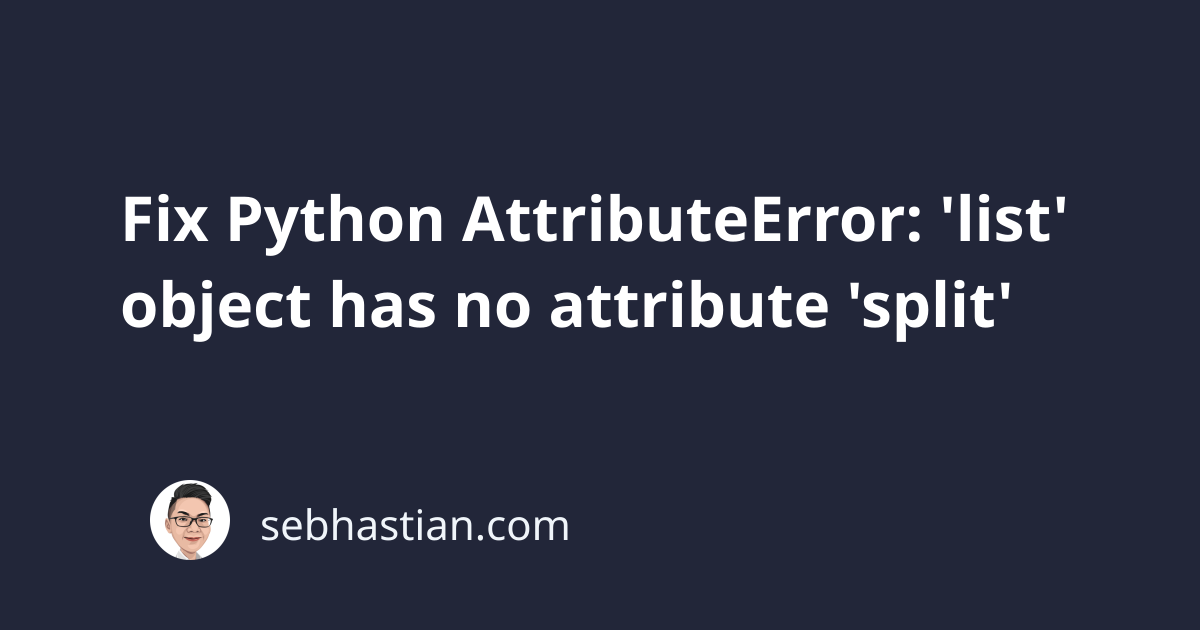
Python shows AttributeError: ’list’ object has no attribute ‘split’ when you try to use the split()
method on a list object instead of a string.
To fix this error, you need to make sure you are calling the split()
method on a string object and not a list. Read this article to learn more.
The split()
method is a string method that is used to divide a string into a list of substrings based on a specified delimiter.
When you call this method on a list object as shown below:
words = ['hello', 'world']
words.split()
You’ll receive the following error response from Python:
To resolve this error, you need to make sure you are calling the split()
method on a string.
You can use an if
statement to check if your variable contains a string type value like this:
words = "hello world"
if type(words) is str:
new_list = words.split()
print(new_list)
else:
print("Variable is not a string")
In the example above, the type()
function is called to check on the type of the words
variable.
When it’s a string, then the split()
method is called and the new_list
variable is printed. Otherwise, print “Variable is not a string” as the output.
If you have a list of string values that you want to split into a new list, you have two options:
- Access the list element at a specific index using square brackets
[]
notation - Use a
for
loop to iterate over the values of your list
Let’s see how both options above can be done.
Access a list element with [] notation
Suppose you have a list of names and numbers as shown below:
users = ["Nathan:92", "Jane:42", "Leo:29"]
Suppose you want to split the values of the users
list above as their own list.
One way to do it is to access the individual string values and split them like this:
users = ["Nathan:92", "Jane:42", "Leo:29"]
new_list = users[0].split(":")
print(new_list) # ['Nathan', '92']
As you can see, calling the split()
method on a string element contained in a list works fine.
Note that the split()
method has an optional parameter called delimiter
, which specifies the character or string that is used to split the string.
By default, the delimiter is any whitespace character (such as a space, tab, or newline).
The code above uses the colon :
as the delimiter, so you need to pass it as an argument to split()
Using a for loop to iterate over a list and split the elements
Next, you can use a for
loop to iterate over the list and split each value.
You can print the result as follows:
users = ["Nathan:92", "Jane:42", "Leo:29"]
for user in users:
result = user.split(":")
print(result)
The code above produces the following output:
['Nathan', '92']
['Jane', '42']
['Leo', '29']
Printing the content of a file with split()
Finally, this error commonly occurs when you try to print the content of a file you opened in Python.
Suppose you have a users.txt
file with the following content:
EMPLOYEE_ID,FIRST_NAME,LAST_NAME,EMAIL
198,Donald,OConnell,[email protected]
199,Douglas,Grant,[email protected]
200,Jennifer,Whalen,[email protected]
201,Michael,Hartl,[email protected]
Next, you try to read each line of the file using the open()
and readlines()
functions like this:
readfile = open("users.txt", "r")
readlines = readfile.readlines()
result = readlines.split(",")
The readlines()
method returns a list containing each line in the file as a string.
When you try to split a list, Python responds with the AttributeError message:
Traceback (most recent call last):
File "script.py", line 10, in <module>
result = readlines.split(",")
^^^^^^^^^^^^^^^
AttributeError: 'list' object has no attribute 'split'
To resolve this error, you need to call split()
on each line in the readlines
object.
Use the for
loop to help you as shown below:
readfile = open("users.txt", "r")
readlines = readfile.readlines()
for line in readlines:
result = line.split(',')
print(result)
The output of the code above will be:
['EMPLOYEE_ID', 'FIRST_NAME', 'LAST_NAME', 'EMAIL\n']
['198', 'Donald', 'OConnell', '[email protected]\n']
['199', 'Douglas', 'Grant', '[email protected]\n']
['200', 'Jennifer', 'Whalen', '[email protected]\n']
['201', 'Michael', 'Hartl', '[email protected]']
You can manipulate the file content using Python as needed.
For example, you can print only the FIRST_NAME
detail from the file using print(result[1])
:
for line in readlines:
result = line.split(',')
print(result[1])
Output:
FIRST_NAME
Donald
Douglas
Jennifer
Michael
Conclusion
To conclude, the Python “AttributeError: ’list’ object has no attribute ‘split’” occurs when you try to call the split()
method on a list.
The split()
method is available only for strings, so you need to make sure you are actually calling the method on a string.
When you need to split elements inside a list, you can use the for
loop to iterate over the list and call the split()
method on the string values inside it.