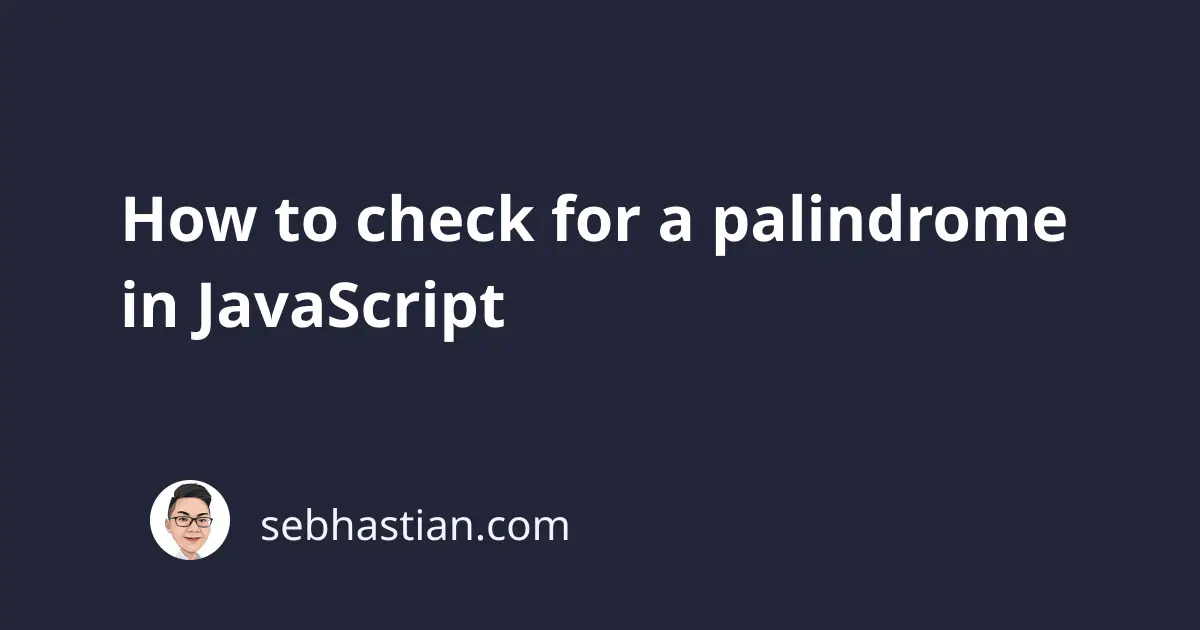
Palindrome is a string that’s spelled the same when read forward and backward. Some palindrome strings are as follows:
- “nope” spelled backwards is “epon”, so it’s not a palindrome
- “civic” spelled backwards is also “civic”, so it’s a palindrome
- “kayak” spelled backwards is also “kayak”, so it’s a palindrome
- “even” spelled backwards is “neve”, so it’s not a palindrome
This tutorial will help you to write a script that enables you to check for palindrome strings. These are the two best ways you can find palindrome strings in JavaScript.
Find a palindrome using Array methods
The easiest way to check for a palindrome string is by using the built-in Array methods called split()
, reverse()
, and join()
. Here’s an example of how you can do so:
let str = "civic";
let strReverse = str.split("").reverse().join("");
if (str === strReverse) {
console.log("The string is a palindrome");
} else {
console.log("NOT a palindrome");
}
The String.split()
method allows you to split a string into an array, while the Array.reverse()
method will reverse the array. Finally, the Array.join()
method will convert your array back into a string. An empty string (''
) is passed both on split()
and join()
methods:
- On
split()
method so that each character in the string will be converted to an array element - On
join()
method so that the array elements will be joined without any separator
And just like that, you can find if a string is a palindrome or not. But finding palindrome is a common interview question, and most likely you won’t be allowed to use this technique. Let’s see how you can also find a palindrome using for
loop.
Finding palindrome using for loop
To find a palindrome string using the for
loop, you need to loop over half of the string and check if the first character of the string matches the last character, and so on. When the characters do not match, that means the string is not a palindrome:
function checkPalindrome(str) {
// find the length of the string
const len = str.length;
// loop through half of the string
for (let i = 0; i < len / 2; i++) {
// check each position
// between the first and the last character
if (str[i] !== str[len - 1 - i]) {
console.log("NOT a palindrome");
}
}
console.log("The string is a palindrome");
}
And with that, you can check for a palindrome word using a for
loop.
JavaScript will consider two same letters with different cases to be different, so you may also need to use .tolowercase()
method before checking for a palindrome so that the string characters will have the same letter cases:
let word = "CivIc";
let str = word.toLowerCase();
function checkPalindrome(str) {
// find the length of the string
const len = str.length;
// loop through half of the string
for (let i = 0; i < len / 2; i++) {
// check each position
// between the first and the last character
if (str[i] !== str[len - 1 - i]) {
console.log("NOT a palindrome");
}
}
console.log("The string is a palindrome");
}
Those are the two best ways you can find a palindrome. Using JavaScript built-in array methods is the easiest way to find a palindrome, but if you are not allowed to use them, you can use a for
loop to finish the job.