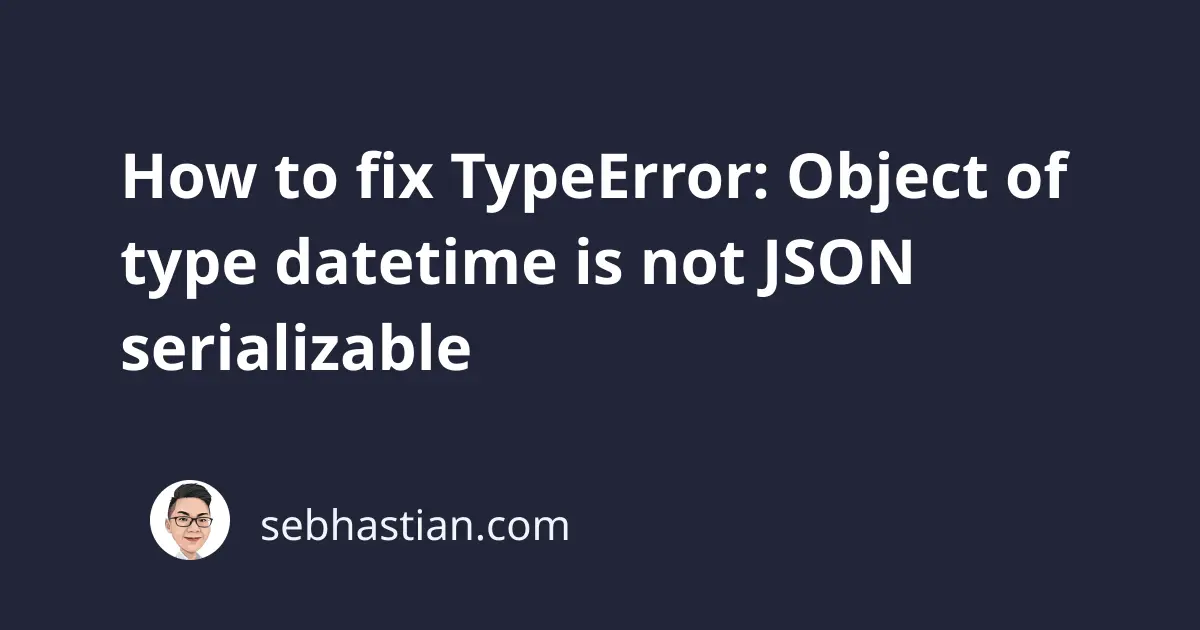
One error that you might encounter in Python is:
TypeError: Object of type datetime is not JSON serializable
This error occurs when you try to serialize a datetime
object into a JSON string.
This tutorial will show you an example that causes this error and how I fix it in practice.
How to reproduce this error
Suppose you created a datetime
object using the datetime()
constructor as shown below:
from datetime import datetime
now = datetime.now()
Next, you include the now
object into a dictionary object and try to serialize that dictionary object using the json.dumps()
method:
import json
from datetime import datetime
now = datetime.now()
data = json.dumps({'created_at': now, 'user': 'Nathan'})
print(data)
But because json.dumps()
doesn’t support serializing a datetime
object, you get this error:
Traceback (most recent call last):
File "main.py", line 5, in <module>
data = json.dumps({'created_at': now, 'user': 'Nathan'})
...
TypeError: Object of type datetime is not JSON serializable
The Python JSON encoder class doesn’t know what to do when you pass a datetime
object in the data.
How to fix this error
To resolve this error, you need to specify the default
argument when passing a data with a datetime
object.
You probably want a string that represents the datetime
object, so put the default=str
argument in the json.dumps()
method as follows:
import json
from datetime import datetime
now = datetime.now()
json_obj = {'created_at': now, 'user': 'Nathan'}
data = json.dumps(json_obj, default=str)
print(data)
The default
argument accepts a function that will be applied to the data in case a TypeError
exception occurs when serializing the object.
As an alternative, you can also call the str()
function for the now
object when including it in the JSON object:
import json
from datetime import datetime
now = datetime.now()
json_obj = {'created_at': str(now), 'user': 'Nathan'}
data = json.dumps(json_obj)
print(data)
With the datetime
object converted into a string, the json.dumps()
method will have no problem serializing the data.
If you want to format the datetime
object, you can also use the strftime()
method, which returns a string from a datetime object in a specific format.
For example, to create a string of yyyy-mm-dd
format:
import json
from datetime import datetime
now = datetime.now()
dt_str = now.strftime("%Y-%m-%d")
json_obj = {'created_at': dt_str, 'user': 'Nathan'}
data = json.dumps(json_obj)
print(data)
Output:
{"created_at": "2023-05-09", "user": "Nathan"}
As you can see, the result string only contains the year, month, and date from the datetime
object as a string.
Conclusion
The Python TypeError: Object of type datetime is not JSON serializable
occurs when you try to serialize a datetime
object into a JSON string.
To resolve this error, you need to convert the datetime
object into a string yourself before passing it to the json.dumps()
method.
You can add a default=str
argument to the method, or you can use the datetime.strftime()
method to convert the datetime object into a string using a specific format.
Other similar errors when creating a JSON serializable include:
- TypeError: Object of type ndarray is not JSON serializable
- TypeError: Object of type function is not JSON serializable
You might want to read these tutorials to help you fix the object is not JSON serializable errors.
I hope this tutorial is helpful. See you again and happy coding! 👋