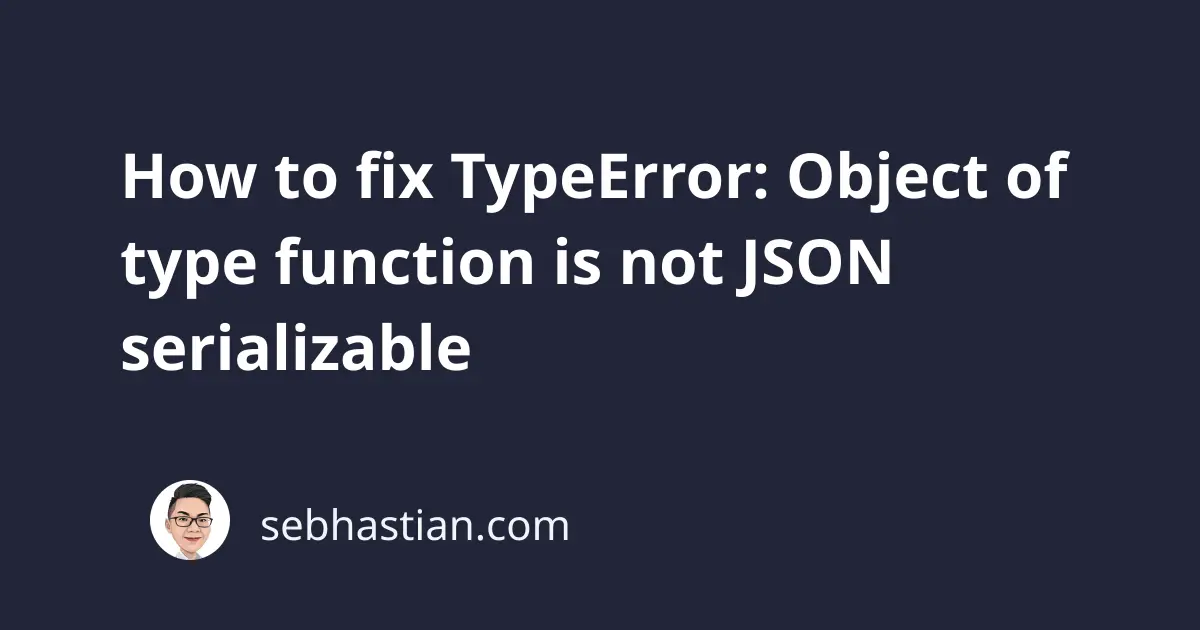
When using the json
module in Python, you might get the following error:
TypeError: Object of type function is not JSON serializable
This error usually occurs when you try to serialize a Python function into a JSON string.
Most likely, you passed the function name without adding parentheses, so the function didn’t get called.
The following tutorial shows an example that causes this error and how to fix it.
How to reproduce this error
Suppose you have a Python function that returns a list
object as shown below:
def get_data():
return [12, 25, 34]
Next, let’s say you wanted to encode the list
object returned by the function into a JSON string.
You called the json.dumps()
method on the function as follows:
import json
def get_data():
return [12, 25, 34]
json_str = json.dumps(get_data)
Python will respond with the following error:
Traceback (most recent call last):
File "main.py", line 6, in <module>
json_str = json.dumps(get_data)
...
TypeError: Object of type function is not JSON serializable
The error occurs because the JSON encoder that’s used in the dumps()
method doesn’t support converting a function into a JSON string.
How to fix this error
If you want to convert the value returned by the function, then you need to call the function by adding parentheses ()
after the function name.
This will fix the error:
import json
def get_data():
return [12, 25, 34]
json_str = json.dumps(get_data())
print(json_str) # [12, 25, 34]
Notice that we didn’t receive any error this time, and the list
object gets converted to a JSON string.
Without the parentheses, you’re passing a reference to the function instead of calling it, which causes the error.
I hope this tutorial is helpful. Happy coding! 🙌