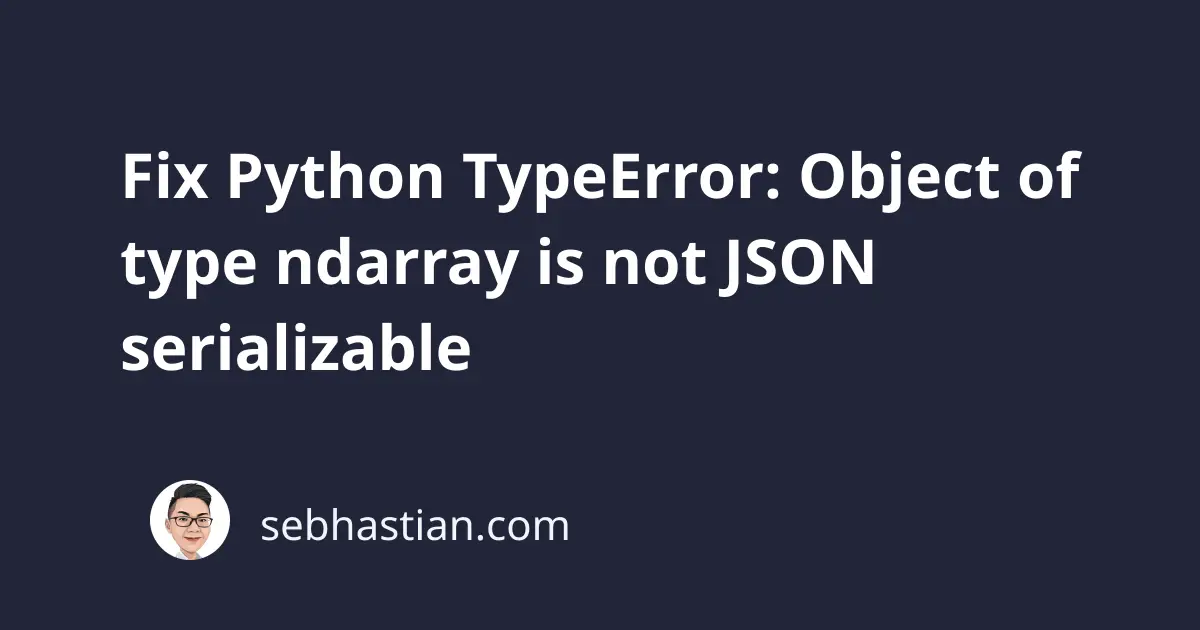
Python shows TypeError: Object of type ndarray is not JSON serializable
when you try to convert a NumPy array to a JSON string.
To solve this error, you need to call the tolist()
method from the NumPy array and turn it into a list object.
A NumPy array can’t be converted into a JSON string because Python doesn’t know how to handle it.
When you run the following code:
import json
import numpy as np
# create a numpy array
data = np.array([1, 2, 3])
# ❌ TypeError: Object of type ndarray is not JSON serializable
json_data = json.dumps(data)
Python responds with an error as follows:
Traceback (most recent call last):
...
TypeError: Object of type ndarray is not JSON serializable
The json
library supports native Python objects and types like dict
, list
, tuple
, str
, .etc.
Fortunately, the NumPy array class has the tolist()
method, which allows you to convert an array to a list.
To fix this error, call the tolist()
method from your array data as shown below:
import json
import numpy as np
data = np.array([1, 2, 3])
json_data = json.dumps(data.tolist()) # ✅
Once you convert the array to a list, the dumps()
method is able to process that list to a JSON string.
Another option for solving this error is to extend the JSONEncoder
class and add custom handler for NumPy arrays.
Consider the code below:
import json
import numpy as np
# Extend the JSONEncoder class
class NumpyEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, np.ndarray):
return obj.tolist()
if isinstance(obj, np.integer):
return int(obj)
if isinstance(obj, np.floating):
return float(obj)
return json.JSONEncoder.default(self, obj)
# create a numpy array
data = np.array([1, 2, 3])
# serialize numpy array as JSON
json_data = json.dumps(data, cls=NumpyEncoder)
print(json_data) # "[1, 2, 3]"
The above code defines the NumpyEncoder
class, which extends the built-in JSONEncoder
class and adds a default()
method for handling numpy arrays.
The default()
method is called by the JSONEncoder
class whenever it encounters an object that it does not know how to serialize. You can override this method to provide custom serialization for specific types of objects.
Three if
statements are defined inside the default()
method to handle NumPy types:
- If the object is an instance of
np.ndarray
, return it as a list - If the object is an instance of
np.integer
, return it as an int - If the object is an instance of
np.floating
, return it as a float
This way, you can handle different data types from NumPy using json.dumps()
method.
To use the extended class, pass a cls
argument when calling json.dumps()
:
json_data = json.dumps(data, cls=NumpyEncoder)
Extending the JSONEncoder
class is also useful when you have a complex type that you want to convert to JSON.
For more information and code examples, you can visit Python json documentation.
Conclusion
The Python json
module can only serialize JSON-serializable data types, such as dictionaries, lists, and primitive data types (strings, numbers, booleans).
When you serialize a NumPy array with the module, Python will respond with a TypeError: Object of type ndarray is not JSON serializable
.
The solution is to convert the array to a native Python list first so that json
module can process it.
Alternatively, you can extend the JSONEncoder
class and let json
knows how to handle complex data types you have in your project.