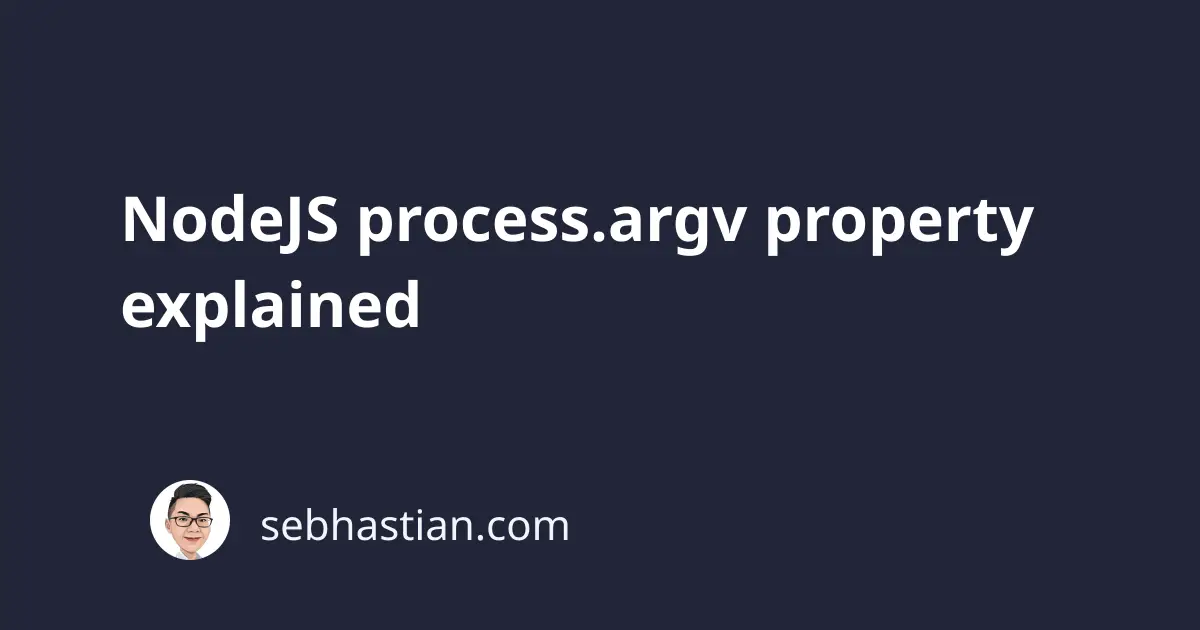
NodeJS has a built-in module called process
that provides you with the information related to your currently running NodeJS process.
The process
module is always available by default, so you don’t need to import it into your code using require()
function.
The process
module has a property named argv
that stores all the command-line arguments passed when you issue the command to start the NodeJS process. The command-line arguments are stored as an array inside the property.
For example, suppose you run an index.js
file using NodeJS command as follows:
node index.js Nathan
Then, inside the index.js
file, you can log the process.argv
value as follows:
console.log(process.argv);
The printed value in the console would be an array similar as follows:
[
'/Users/nsebhastian/.nvm/versions/node/v16.3.0/bin/node',
'/Users/nsebhastian/Desktop/test/index.js',
'Nathan'
]
As you can see from the log result above, the argv
property contains a single array.
The index 0
stores the path of your runnable Node program, while index 1
stores the path to the index.js
script. The arguments you passed when you start the NodeJS process will be stored from index 2
onward.
This is why you can see the argument Nathan
from the command-line above at index 2
. The more arguments you passed to the process, the longer the array length will be.
Here’s another example with more than one argument passed to the node
command:
node index.js Nathan 18 true
The output will be as follows. Note how the number and boolean value are stored as strings inside the array. The argv
property stores all data types as a string:
[
'/Users/nsebhastian/.nvm/versions/node/v16.3.0/bin/node',
'/Users/nsebhastian/Desktop/test/index.js',
'Nathan',
'18',
'true'
]
Since the argv
property is a regular JavaScript array type, you can use Array
object methods like forEeach
with the property as follows:
const { argv } = process;
argv.forEach((val, index) => {
console.log(`${index}: ${val}`);
});
The console log result will be similar as follows:
0: /Users/nsebhastian/.nvm/versions/node/v16.3.0/bin/node
1: /Users/nsebhastian/Desktop/test/index.js
2: Nathan
3: 18
4: true
You can also use array destructuring assignment syntax to grab the arguments from the argv
property as follows:
const { argv } = process;
const [node, script, username, age, isMember] = argv;
console.log(node); // /Users/nsebhastian/.nvm/versions/node/v16.3.0/bin/node
console.log(script); // /Users/nsebhastian/Desktop/test/index.js
console.log(username); // "Nathan"
console.log(age); // "18"
console.log(isMember); // "true"
To skip the first two indices, you can omit them from the destructuring syntax as follows:
const { argv } = process;
const [, , username, age, isMember] = argv;
console.log(username); // "Nathan"
console.log(age); // "18"
console.log(isMember); // "true"
And that’s all you need to know about the process.argv
property. The property is also useful as a point of reference when you want to set up conditionals inside your JavaScript code.
For example, you can write an if..else
block to execute a piece of code only when the username
argument is defined:
const username = process.argv[2];
if(username){
console.log(`Greetings, ${username}!`);
} else {
console.log("Hello, world!");
}
Then when you run the script without the argument, JavaScript will execute the code inside the else
block.
Keep in mind that NodeJS doesn’t store the passed arguments as a key/value pair, so you need to pass the arguments in the right order, just like when you pass arguments to a function.