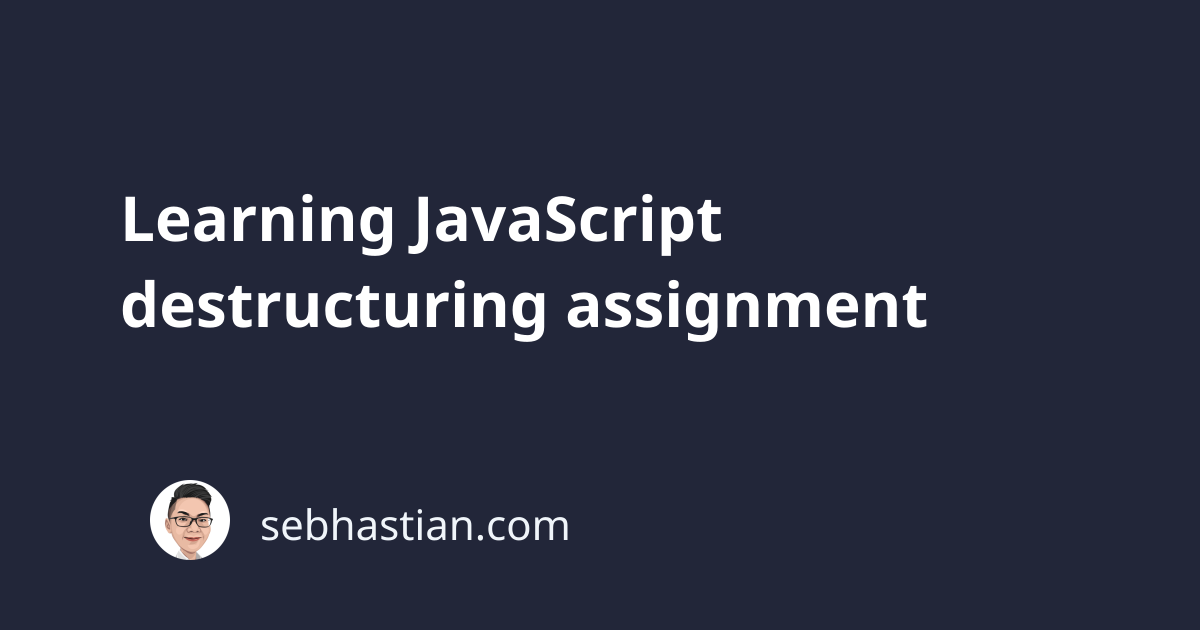
The destructuring assignment was added into JavaScript with the release of ES2015 (also know as ES6) and it has become one of the most useful features of JavaScript language because of two reasons:
- It helps you to avoid code repetition.
- It keeps your code clean and easy to understand.
This post will help you learn about the destructuring assignment with practical examples.
So what is the destructuring assignment?
It’s a special syntax that allows you to “unpack” or “extract” the values of JavaScript arrays and objects.
Destructuring Arrays
Here’s how you normally assign an array values to variables:
const sampleArray= ['Jane', 'John']
const firstIndex = sampleArray[0]
const secondIndex = sampleArray[1]
And here’s how you do it with destructuring assignment:
const sampleArray= ['Jane', 'John']
const [firstIndex, secondIndex] = sampleArray
The above code will return the same value for firstIndex
and secondIndex
variable. No matter how many elements you have, the destructuring will start from the zero index. You can also use the rest operator ...
to copy the rest of the values after your assignment. Take a look at the following example:
const sampleArray= ['Jane', 'John', 'Jack', 'Aston']
const [one, two, ...rest] = sampleArray
The rest
variable will contain an array with values of ['Jack','Aston']
.
You can also put default values for these variables when the extracted value is undefined:
const [a = 'Martin', b = 10] = [true]
// a will return true
// b will return 10
You can also immediately assign the return of a function into assignments. This is frequently used in libraries like React:
const [ a, b ] = myFn()
function myFn() {
return ['John', 'Jack']
}
The variable a
will return “John” and b
will return “Jack”.
Finally, you can also ignore some variables by skipping the assignment for that index:
const [ a, ,b ] = [ 1, 2, 3 ]
// a will return 1
// b will return 3
Destructuring assignment makes unpacking array values easier and shorter, with less repetition.
Object destructuring
Just like arrays, you can destructure objects the same way, but instead of using the square brackets ([]
) you need to use the curly brackets ({}
):
const user = {
firstName: "Jack",
lastName: "Smith"
}
const { firstName, lastName } = user
You can use the colon delimiter (:
) to rename the property into different name. Let’s rename firstName
into just name
:
const user = {
firstName: "Jack",
lastName: "Smith"
}
const { firstName: name, lastName } = user
Please note that you still only create two variables: name
and lastName
. The firstName
is assigned to name
, so it won’t create a separate variable.
Just like arrays, you can destructure an object returned by a function immediately:
const { firstName, lastName } = myFn()
function myFn(){
return { firstName: "Jack", lastName: "Austin" }
}
Also, you can destructure an object from the function parameters, exactly when you define the function:
function myFn( { firstName, lastName } ){
return firstName + ' ' + lastName
}
const user = {
firstName: "Jack",
lastName: "Austin"
}
const name = myFn(user)
Some real use cases for destructuring assingment is very beneficial, particularly when you use a library like React.