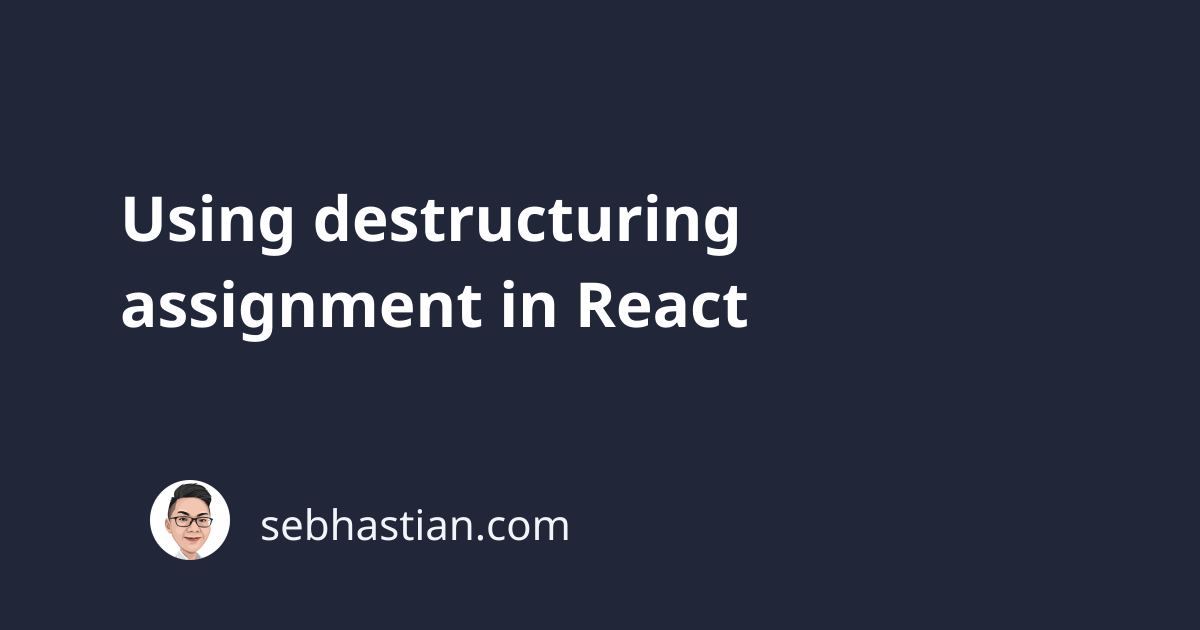
Destructuring assignment is a feature of JavaScript introduced by ES6 (or ES 2015) that’s available for both object and array data types. It allows you to assign the values of an array or the properties of an object without needing to reference the variable directly in the assignment.
Here’s the traditional way of assigning a value to a variable in JavaScript:
const sampleObject = {
name: "John",
age: 25
}
const name = sampleObject.name
const age = sampleObject.age
With destructuring assignment, your code will look like this:
const sampleObject = {
name: "John",
age: 25
}
const { name, age } = sampleObject
You just turned two lines of assignment into one. Awesome, right? The same is also possible with an array, but instead of using curly brackets({}
) you need to use square brackets ([]
) on the assignment:
const myArray = [1,2]
const [firstIndex, SecondIndex] = myArray
In fact, the useState
hook from React returns an array with two values that we destructure immediately. Here’s an illustration of how it works:
const [name, setName] = useState('name')
function useState(defaultValue){
// executing React state mechanism..
return [value, updateFunction]
}
The benefits of using destructuring assignment is especially visible when using React because you would deal with state
and props
frequently. For example, a functional component may receive a single prop object with many properties like this:
const userData = {
auth: {
loggedIn: true,
serial: "yfueqh129slx9283929",
},
user: {
firstName: "John",
lastName: "Doe",
age: 27,
email: "[email protected]",
friendsCount: 29,
education: "Bachelor"
}
}
<Profile auth={auth} user={user} />
When you need to display the props in your interface, you’d probably pass the prop into the component and use the value with props.
syntax:
function Profile(props){
if(props.userData.auth.loggedIn){
return (
<>
<h1> Profile </h1>
<h2> Name: {props.userData.user.firstName} {props.userData.user.lastName}</h2>
<h3> email: {props.userData.user.email}</h3>
</>
)
}
return <h1>You need to log in first</h1>
}
Yikes! Look at how long the syntax just for displaying a name. When using destructuring assignment, you can reduce that long syntax and make it better. First, destructure the userData
props before passing it to the component:
const { auth, user} = userData
Then pass it into the component as two props:
<Profile auth={auth} user={user}>
Or you can also use spread operator so you don’t need to destructure it:
<Profile {...userData} />
Then immediately destructure the props
object when you define the parameters in the function, like this:
function Profile( {auth, user} ) {
And now you can use the arguments inside your props with less repetition:
function Profile( {auth, user} ) {
if(auth.loggedIn){
return (
<>
<h1> Profile </h1>
<h2> Name: {user.firstName} {user.lastName}</h2>
<h3> email: {user.email}</h3>
</>
)
}
return <h1>You need to log in first</h1>
}
Want even less repetition? You can even destructure nested properties with JavaScript!
function Profile({
auth: { loggedIn },
user: { firstName, lastName, email }
}) {
if (loggedIn) {
return (
<>
<h1> Profile </h1>
<h2> Name: {firstName} {lastName}</h2>
<h3> email: {email}</h3>
</>
);
}
return <h1>You need to log in first</h1>;
}
Here’s a Code Sandbox example that you can tweak with.
The syntax looks a bit complicated at first, so here’s how to make destructuring easy in two steps process:
First, you need to find the name of the properties you want to extract first. In the example, the goal is to extract loggedIn
, firstName
, lastName
, and email
properties:
After that, you simply find the first-level properties that hold the second-level properties. Write the property name:
{
auth: {},
user: {}
}
Then write the desired properties inside the curly brackets:
{
auth: { loggedIn },
user: { firstName, lastName, email }
}
The same could also be applied in class-based components. You simply destructure the props in your render()
function:
class Profile extends React.Component {
render(){
const {
auth: { loggedIn },
user: { firstName, lastName, email }
} = this.props
return (
// ...
)
}
}
And that’s it. Destructuring assignment is a wonderful feature from ES6 that really helps you to avoid repetition and keep your code clean. You can extract nested properties of an object and simply use them inside your render function.