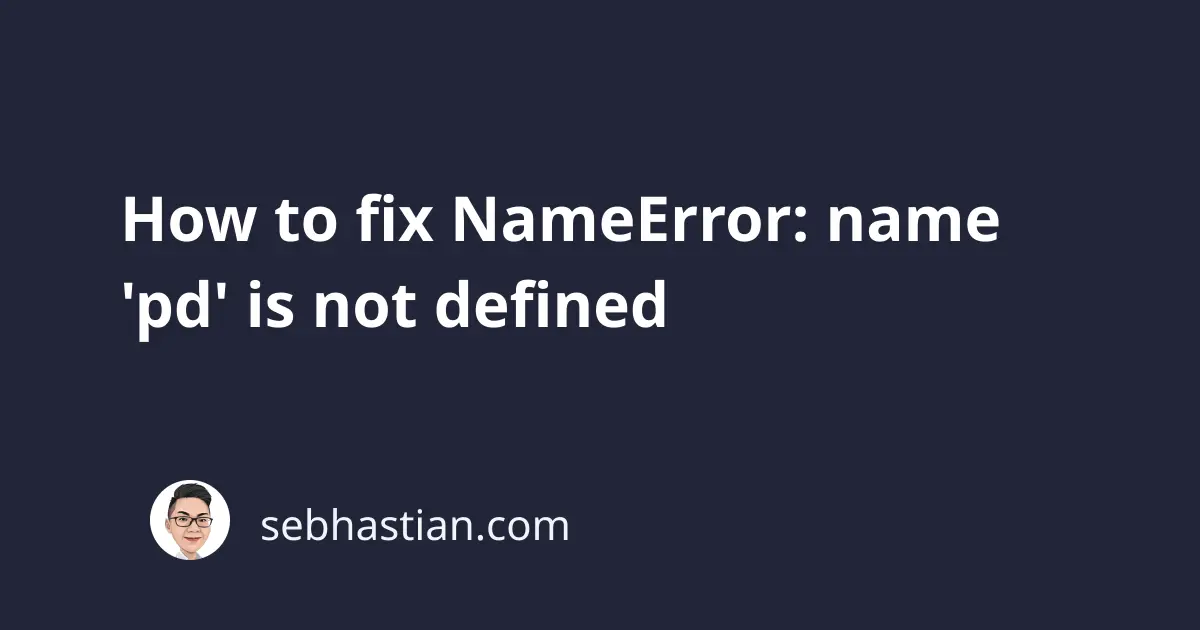
When working with the pandas library, you might get the following error:
NameError: name 'pd' is not defined
This error commonly occurs when you import the pandas
library without giving it an alias as pd
at the top of your file.
The following tutorial shows an example that causes this error and how to fix it.
How to reproduce this error
Suppose you import the pandas
library into your project as follows:
import pandas
Next, you create a DataFrame
object using the pandas library as follows:
df = pd.DataFrame({"distance": [3.6, 18.3, 21.5, 25.2]})
You get the following error when running the code:
Traceback (most recent call last):
File "main.py", line 3, in <module>
df = pd.DataFrame({"distance": [3.6, 18.3, 21.5, 25.2]})
NameError: name 'pd' is not defined. Did you mean: 'id'?
This error occurs because Python doesn’t understand the pd
object from where you call the DataFrame()
attribute.
How to fix this error
To resolve this error, you need to alias the pandas
library as pd
when importing it as follows:
import pandas as pd
df = pd.DataFrame({"distance": [3.6, 18.3, 21.5, 25.2]})
By adding the pd
alias, you can write pd
instead of pandas
when using attributes and functions from the module.
Alternatively, you can also change pd
to pandas
to fix this error without adding an alias as follows:
import pandas
df = pandas.DataFrame({"distance": [3.6, 18.3, 21.5, 25.2]})
But this means you need to change all occurrences of pd
to pandas
in your code.
If you’re using a Jupyter Notebook, make sure that you modify and run the code block that imports the pandas
library as follows:
Once you run the first block as shown above, you won’t receive any error when running the pd.DataFrame()
line.
More resources
The following tutorials explain how you can fix other common NameErrors in Python:
How to Fix Python NameError: name ‘sys’ is not defined
How to Fix Python NameError: name ’nltk’ is not defined
I hope these tutorials are helpful. Happy coding! 🙌