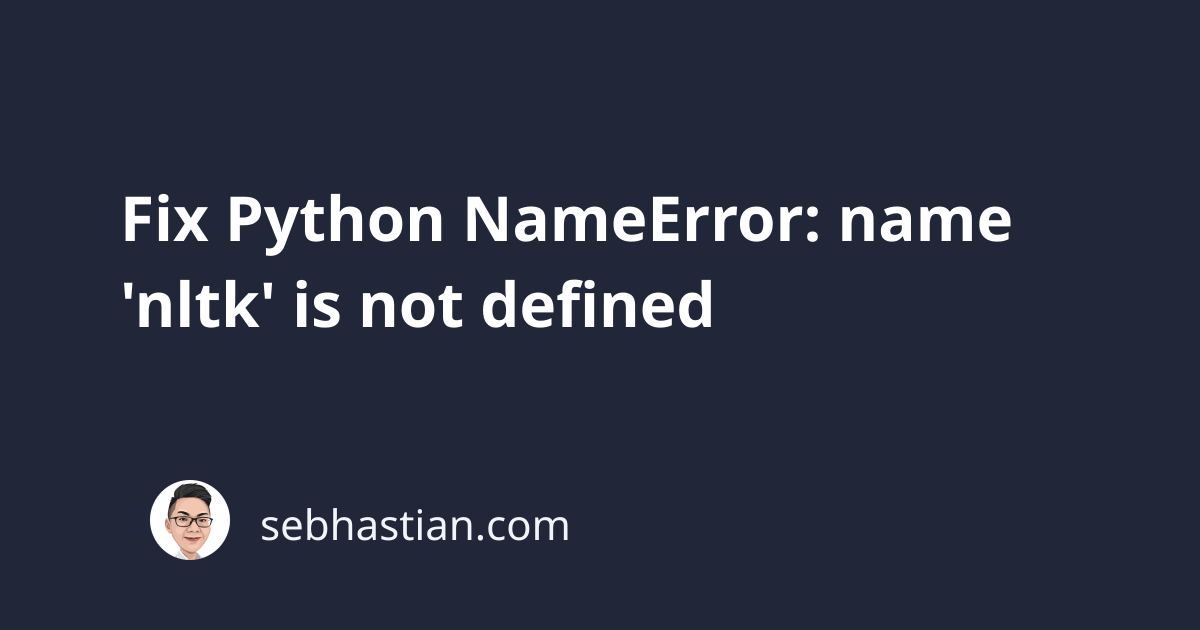
When you use the Natural Language Toolkit (NLTK) in your Python code, you might get the following error:
NameError: name 'nltk' is not defined
This error occurs because Python can’t find the nltk
module in your current environment.
The following tutorial shows examples that cause this error and how to fix them.
1. You forget to install the nltk module
Before you try anything else, make sure that nltk is installed by running one of the following commands:
pip install nltk
# If you have pip3:
pip3 install nltk
# If no pip in PATH:
python -m pip install nltk
python3 -m pip install nltk
# Windows:
py -m pip install nltk
# From Jupyter Notebook:
!pip install nltk
#or
!pip3 install nltk
Once the package is installed, try importing the module as follows:
import nltk
print(nltk.__version__)
You should see the version number of the installed nltk
module printed on the console. This means you installed and imported the nltk package successfully.
2. Check if you have multiple Python versions installed
If you still see the error, then you may have multiple versions of Python installed on your system.
You can check this by running the which -a python
and which -a python3
commands from the terminal:
$ which -a python
/usr/local/bin/python
$ which -a python3
/usr/local/bin/python3
/usr/bin/python3
In the example above, there are multiple versions of Python installed on the system:
- Python 2 installed on
/usr/local/bin/python
- Python 3 installed on
/usr/local/bin/python3
and/usr/bin/python3
Each Python distribution is bundled with a specific pip
version. If you want to install a module for Python 2, use pip
. Otherwise, use pip3
for Python 3.
The problem arise when you install nltk for Python 2 but runs the code using Python 3, or vice versa:
# Install nltk for Python 2
$ pip install nltk
# Run the code with Python 3
$ python3 main.py
# Or Install nltk for Python 3
$ pip3 install nltk
# Run the code with Python 2
$ python main.py
If you install nltk for Python 2, then you need to run the code using Python 2 as well:
$ pip install nltk
$ python main.py
Having multiple Python versions can be confusing. You must carefully inspect how many Python versions you have on your computer, then find out which pip
and python
versions you are calling from the terminal.
Once you use the right pip
and python
versions, you should be able to import nltk successfully.
3. No module named nltk in Visual Studio Code (VSCode)
If you use VSCode integrated terminal to run your code, you might get this error even when nltk is already installed.
This means the python
and pip
versions used by VSCode differ from the one where you install nltk.
You can check this by running the following code:
import sys
print(sys.executable)
The print
output will show you the absolute path to the Python used by VSCode. For example:
/path/to/python3
Copy the path shown in the terminal and add -m pip install nltk
as follows:
/path/to/python3 -m pip install nltk
The command will install nltk for the Python interpreter used by VSCode. Once finished, try running your code again. It should work this time.
Conclusion
Most likely, you see the NameError: name 'nltk' is not defined
error because you didn’t install the nltk
package from pip
, or you installed the package on a different version of Python.
The steps shown in this article should help you fix this error.
I hope this article is helpful. Happy coding! 👋