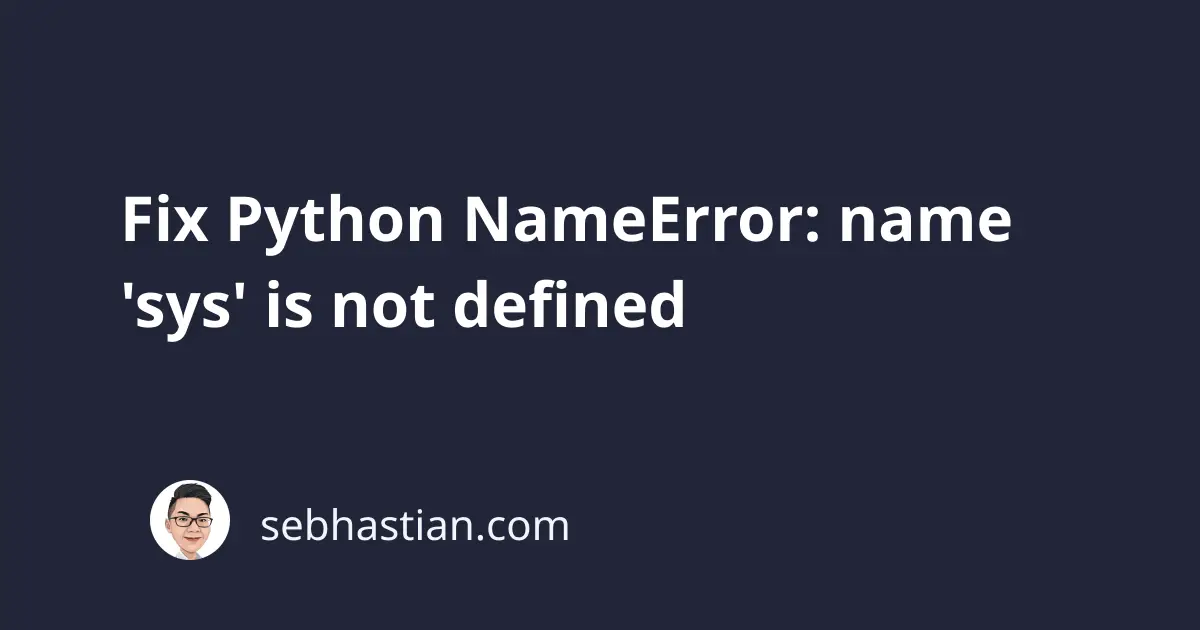
Python shows the NameError: name 'sys' is not defined
error message when you call the sys
module without importing it.
To fix this error, you need to import the sys
module at the beginning of your script.
The sys
module is a built-in Python module that provides access to system-related information, such as the version of your Python interpreter, the OS platform, or the command-line arguments added.
When you try to access the module as shown below:
print(sys.version)
print(sys.exit("System exit"))
If you didn’t add an import
statement at the top of the file, Python will respond with the following error:
Traceback (most recent call last):
File ...
print(sys.version)
NameError: name 'sys' is not defined
To fix this error, add an import
statement at the top of your Python script file as shown below:
import sys
print(sys.version)
print(sys.exit("Sys module exit"))
Also, please make sure that you are not importing the sys
module in a nested scope as shown below:
def print_sys_info():
import sys
print("Python version:", sys.version)
print("Command line arguments:", sys.argv)
# ❌ Call sys outside the module
print("Current platform:", sys.platform)
The example above imports the sys
module inside the print_sys_info()
function.
When accessing the sys.platform
constant outside the function, the error occurs.
This is because importing a module inside a function only makes it available within that function scope. The module won’t be available globally.
If you need the module outside of the function, then place the import
statement at the top-level as shown below:
import sys
def print_sys_info():
print("Python version:", sys.version)
print("Command line arguments:", sys.argv)
print_sys_info()
print("Current platform:", sys.platform)
Alternatively, you can import only specific functions and constants you need into your code:
from sys import version, platform, exit
print("Accessing sys module")
print("Python version:")
print(version)
print("OS platform version:")
print(platform)
print(exit("Sys module exit"))
Named imports as shown above give you more clarity as to which part of the module you used in the code.
You also optimize your Python code by importing only specific functions and constants that you need.
You can read all of the functions and constants defined in the sys
module on the Python sys documentation page.
Conclusion
To summarize, the Python NameError: name 'sys' is not defined
error occurs when the sys
module is not imported.
To solve this error, make sure to import the sys
module at the beginning of your script and use its functions and variables as you need.
Good work solving the error! 👍