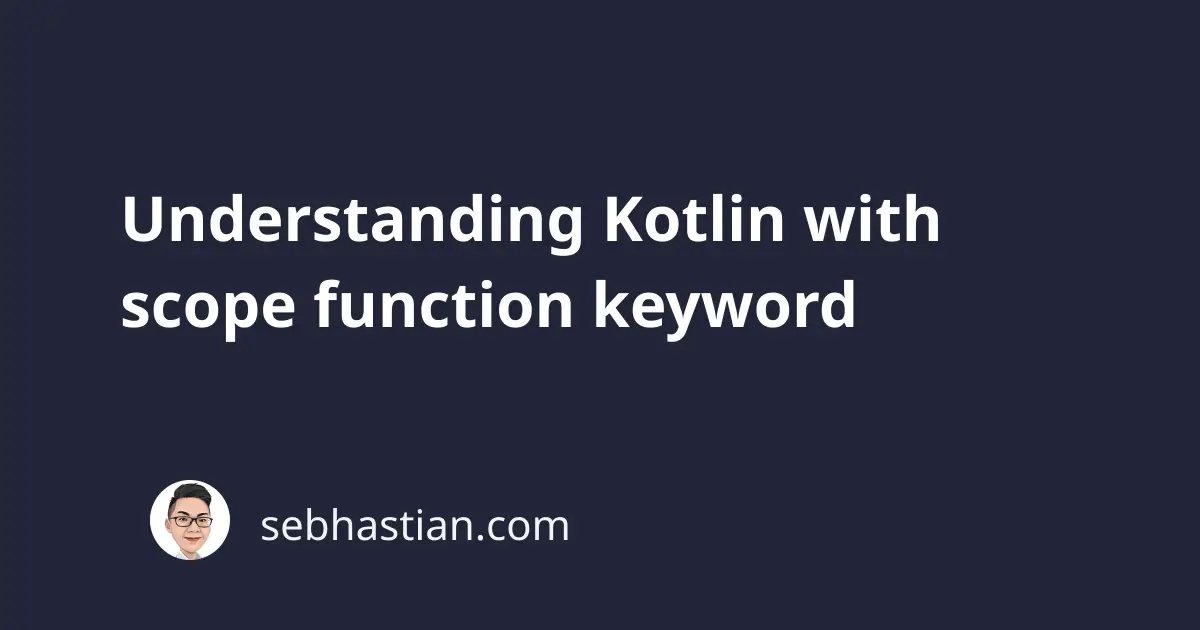
In Kotlin, the with
keyword is used to define a scoped function.
A Kotlin scoped function is a function that gets executed under the context of an object.
The object that you use to call the scoped function will be available inside the function under the it
or this
keyword, depending on the keyword you use to create the function.
There are other keywords that you can use to create a scoped function in Kotlin:
This tutorial will focus on understanding the with
keyword in action.
Suppose you have a Kotlin class
as shown below:
class User(var username: String, isActive: boolean) {
fun hello() {
println("Hello! I'm $username")
}
}
Next, you create an object instance of the class
above, then change the initialized property as follows:
val user = User("Nathan", true)
user.username = "Jane"
user.isActive = false
While there’s nothing wrong with the above code, you can actually make the code less redundant by NOT calling the user.propertyName
twice.
You can do this using the with
keyword, which allows you to access the object without having to refer to it as follows:
val user = User("Nathan", true)
with(user) {
username = "Jane"
isActive = false
}
Inside the with
keyword function body, you can refer to the user
object using the this
keyword.
You can also omit referring to it explicitly because Kotlin is smart enough to know that the assignment for username
and isActive
above refers to the object property.
This is how a scoped function works in Kotlin. It allows you to write more concise code when doing something with your object.
Because everything is an Object
in Kotlin, you can even write scoped functions for built-in data types.
The following example shows how to use the with
function on a String
variable:
val str = "Good Morning!"
with(str){
println("The string length is $length")
println("The character at index 3 is ${get(3)}")
}
The println
output above will be as follows:
The string length is 13
The character at index 3 is d
The with
keyword is a bit different from the rest of the scoped function keywords.
Instead of calling the function as a property of the object, you pass the object as an argument to the function.
Consider the following example:
val str = "Good Morning!"
with(str) {
println("The string length is $length")
}
str.run {
println("The string length is $length")
}
Both with
and run
function above generates the same result.
And that’s how the with
keyword works in Kotlin 😉