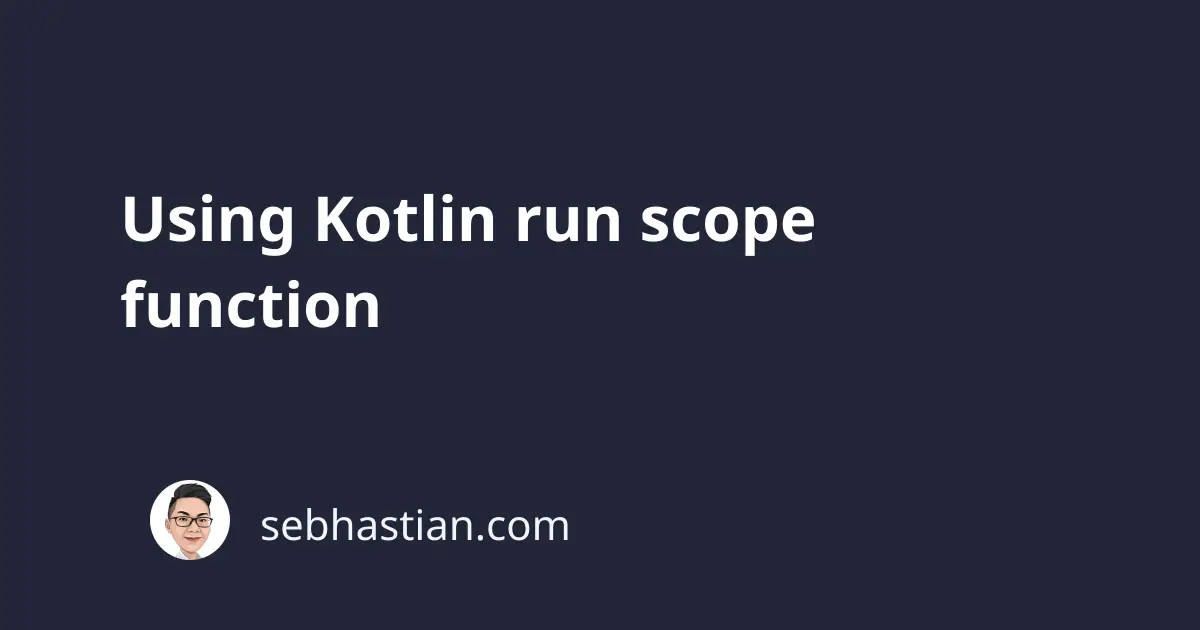
The run
keyword is used to create a scoped function in Kotlin.
A scoped function is a function that’s called under the context of an object.
While a scoped function doesn’t add any new capabilities to your source code, it helps in writing a more concise code.
Let’s learn how to use the run
function with code examples.
Suppose you have a class named Car
with the following definitions:
class Car(var name: String, var year: Int) {
fun hello() {
println("Hello! I'm a $name")
}
}
Next, you create an instance of the Car
class:
val car = Car("Tesla S", 2021)
When you want to change the properties of the car
instance above, you might do it like this:
car.name = "Ford Mustang Mach-E"
car.year = 2022
Using the run
method, you can do the same changes with the following syntax:
car.run {
name = "Ford Mustang Mach-E"
year = 2022
}
println(car.name) // Ford Mustang Mach-E
println(car.year) // 2022
Instead of the usual function call with parentheses ()
before the opening bracket, the run
method above is called with a lambda expression as its body block.
Inside the body of the function, the car
object is available for you to access using the this
keyword.
But you can also omit the this
keyword as you can see in the example above.
The run keyword also returns the result of the lambda expression that you can save in a variable if you need it.
For example, the following run
method returns a string Hello World
:
val result = car.run {
car.name = "Ford Mustang Mach-E"
car.year = 2022
"Hello World"
}
println(result) // Hello World
When there’s no value returned from the function body, then an empty Unit
will be returned:
val result = car.run {
car.name = "Ford Mustang Mach-E"
car.year = 2022
}
println(result) // kotlin.Unit
And that’s how the run
scope function works in Kotlin.
It can be called from any valid object in Kotlin, so you can also call it from an instance of Kotlin built-in object.
The example below calls the run
function from a String
instance:
val str = "Hello World"
val char = str.run {
println(length) // 11
get(1)
}
println(char) // e
Great work learning about the run
scope function in Kotlin. 👍