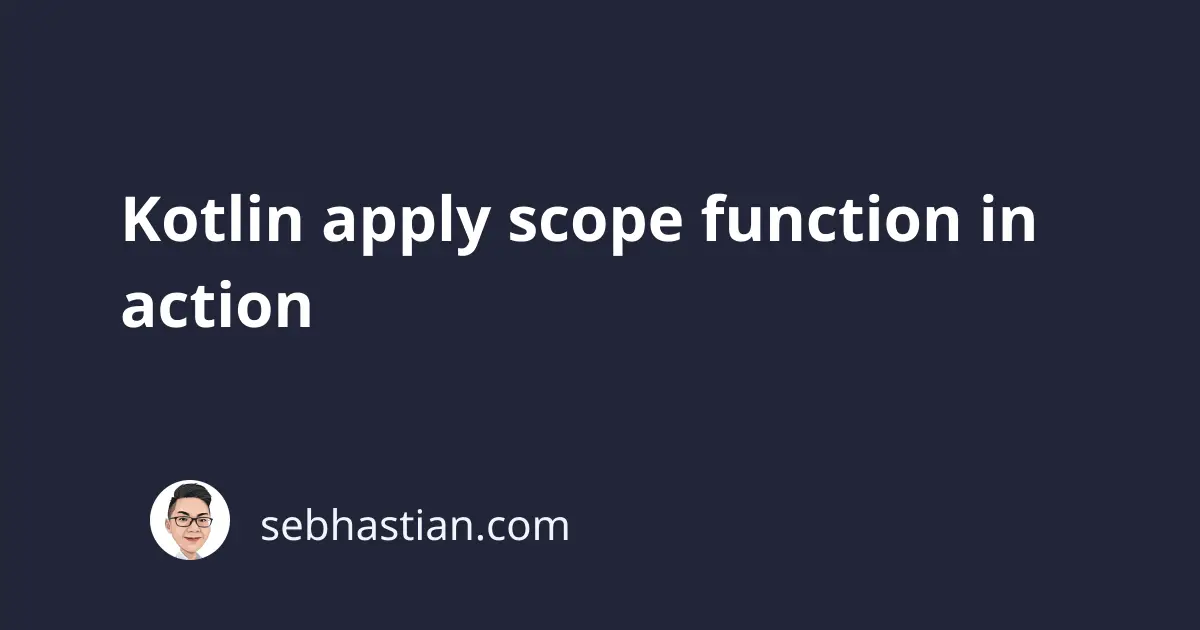
The apply
keyword is one of the keywords used to create a scope function in Kotlin.
A scope function is a block of code that’s executed under the context of an object where you call the function.
With the apply
function keyword, you’ll be able to modify or call the methods of your object in one scoped block.
Let’s see the apply
function in action. Suppose you have a User
class with the following definitions:
class User(var username: String, var isActive: Boolean) {
fun hello() {
println("Hello! I'm $username")
}
}
Next, you create an instance of the class with the following code:
val user = User("Nathan", true)
You can change the object properties and call its hello()
method as follows:
val user = User("Nathan", true)
user.username = "Jane"
user.isActive = false
user.hello()
Alternatively, you can use the apply
keyword and create a lambda expression that operates on your object.
Take a look at the following code:
user.apply {
username = "Jane" // or this.username = "Jane"
isActive = false
hello()
}
Inside the apply
lambda expression body scope, you can access the properties and methods of the context object using the this
keyword.
But Kotlin is also smart enough that you can omit the this
keyword when accessing the members of the object, as shown above.
The apply
method returns the context object as its return value. While you can call the methods defined in your class
from inside it, any value returned by your method will be ignored.
For example, let’s add a function named getNum()
that returns a number value in the User
class:
class User(var username: String, var isActive: Boolean) {
fun hello() {
println("Hello! I'm $username")
}
fun getNum(): Int {
return 7
}
}
Next, call it inside the apply
block as follows:
val user = User("Nathan", true)
val num = user.apply {
getNum()
}
println(num) // User@51016012
Instead of returning the number 7
to the num
variable, the apply
method returns the object itself.
The apply
method can be read as an instruction to “apply the following configuration changes to the object”, so calling methods from inside the method is not recommended.
Because the object is the return value, you can add a function call chain immediately after the apply
block like this:
user.apply {
username = "Jane"
isActive = false
}.hello() // Hello! I'm Jane
If you need a different return value from the scoped function, you need to use the let
function instead of the apply
function.
Learn more: Kotlin let function keyword explained
Now you’ve learned how the apply
function works in Kotlin. Good work! 👍