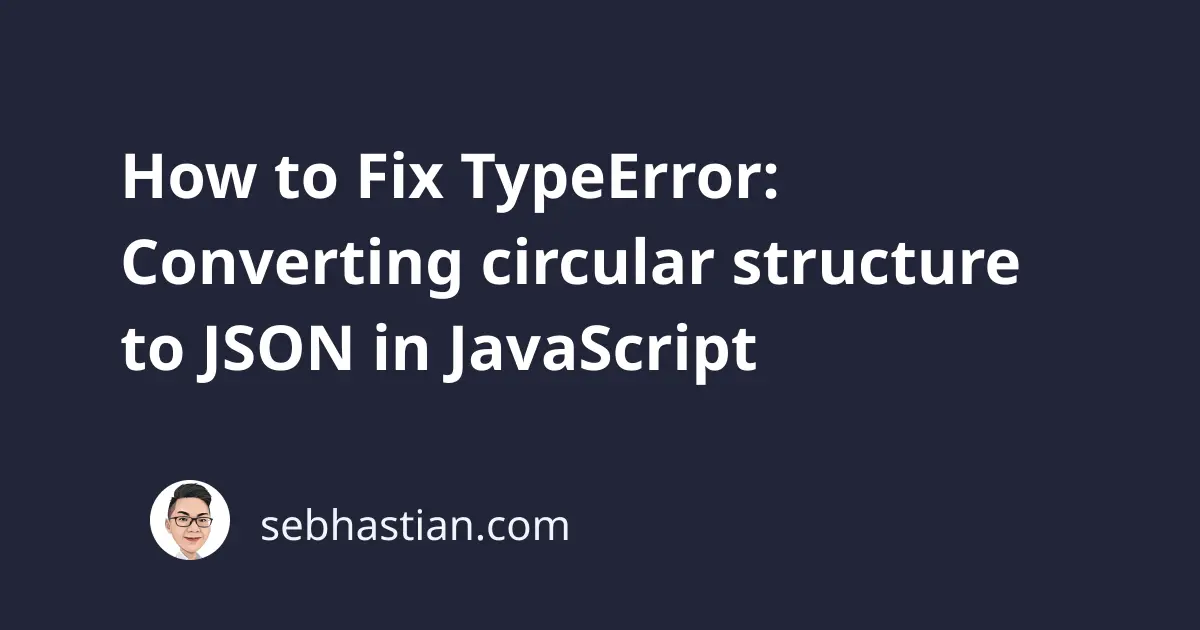
The TypeError: Converting circular structure to JSON occurs when you try to convert an object that has a reference to itself into a JSON string.
The example code that causes the error looks like this:
let user = { name: 'Nathan' };
user.comment = user;
JSON.stringify(user);
Here, you can see that the user
object has a property called comment
that got assigned the user
object itself. This is what JavaScript meant with a circular reference.
When you pass an object with a circular reference to the JSON.stringify()
method, you get this error:
Uncaught TypeError: Converting circular structure to JSON
--> starting at object with constructor 'Object'
--- property 'comment' closes the circle
at JSON.stringify (<anonymous>)
at <anonymous>:5:6
The solution to fix this error is simple: You need to avoid referencing the object itself into one of its properties as shown above, and the JSON.stringify()
method should work.
let user = { name: 'Nathan' };
user.comment = ''; // pass an empty string
JSON.stringify(user); // ✅
If you really need to refer to the object itself in your code, then you can install the flatted package that can convert a circular structure without any issue.
Install the package, then import it into your code as follows:
const {parse, stringify} = require('flatted');
let user = { name: 'Nathan' };
user.comment = user;
// convert to JSON
let myJson = stringify(user);
// convert back to object
let myObject = parse(myJson);
You can use the stringify()
method from flatted
to convert an object into a JSON string, then use the parse()
method to convert the JSON string back to an object.
And that’s how you solved the TypeError: Converting circular structure to JSON in JavaScript.
I also have more articles related to JSON manipulations in JavaScript as follows:
These articles should help you in working with JSON data in JavaScript. Happy coding!