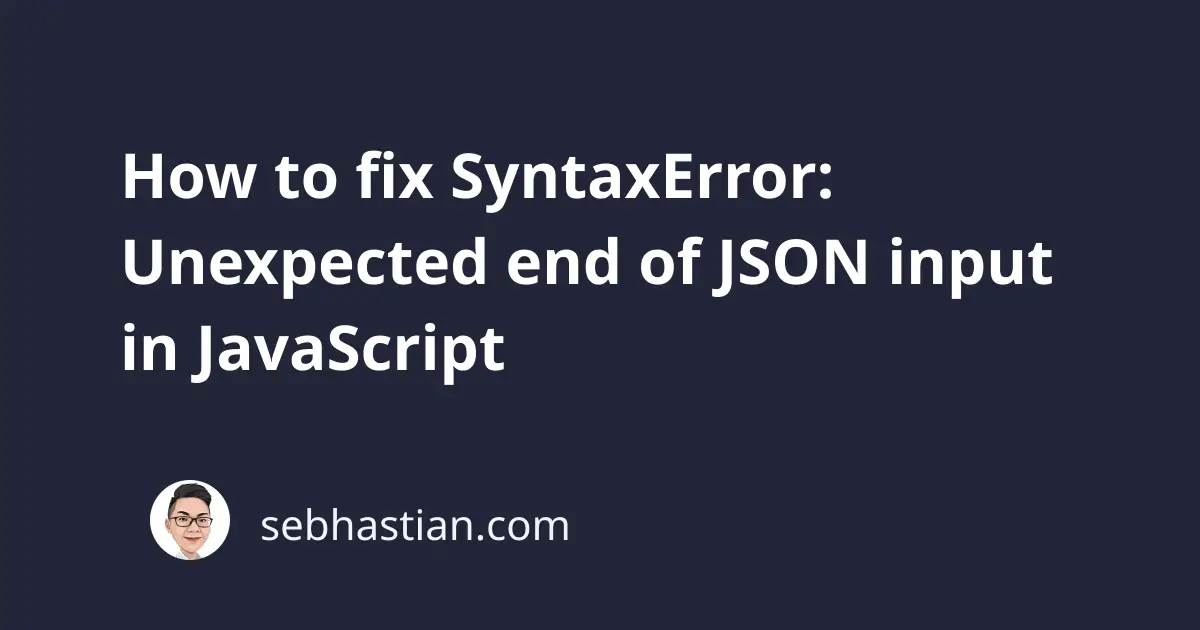
The “Unexpected end of JSON input” error occurs when you call the JSON.parse()
method and pass incomplete or invalid JSON data as its argument.
For example, suppose you call the JSON.parse()
method with an empty string as follows:
JSON.parse("");
Here, there’s no value to parse as an object, so JavaScript reached the end of the string before parsing a valid JSON text.
The output will be:
Uncaught SyntaxError: Unexpected end of JSON input
at JSON.parse (<anonymous>)
at <anonymous>:1:6
To resolve this error, you need to make sure that you’re passing an argument that can be converted into a JavaScript object.
Let’s see some examples which cause this error. One example is when you pass a JSON string that’s missing a closing curly bracket as follows:
let jsonObj = JSON.parse('{ "key": "value"'); // ❌
Here there’s no closing curly bracket }
symbol after the “value” entry, so the error occurs.
Another example is when you parse an empty array:
let jsonObj = JSON.parse([]); // ❌
Because an empty array doesn’t contain any valid JSON data at all, the same error occurs.
You might also see this error when trying to fetch data from local storage as follows:
let user = JSON.parse(localStorage.getItem('user') || '');
Here, the logical OR ||
operator is used to provide a fallback value should the getItem()
method returns a falsy value.
But because the fallback value is an empty string, the error is triggered. To resolve the error, make sure your fallback value is a valid JSON string.
The following example can work:
let fallbackJson = '{ "user": "null" }';
let user = JSON.parse(localStorage.getItem('user') || fallbackJson);
console.log(user) // {user: 'null'}
Or you can also remove the fallback value because JSON.parse()
can handle a null
value just fine.
The getItem()
method returns null when the key you passed doesn’t exist:
let user = JSON.parse(localStorage.getItem('user')); // ✅
There may be more possible scenarios that can cause this error. The key to resolving this error is to check if you have a valid JSON string input.
You can use an online JSON formatter to help you check on the input. Pass the JSON string to the provided input area without the quotation mark.
For example, pass the data '{ "key": "value"'
as { "key": "value"
and the formatter will help you fix the error.
I hope this tutorial helps. Happy coding!