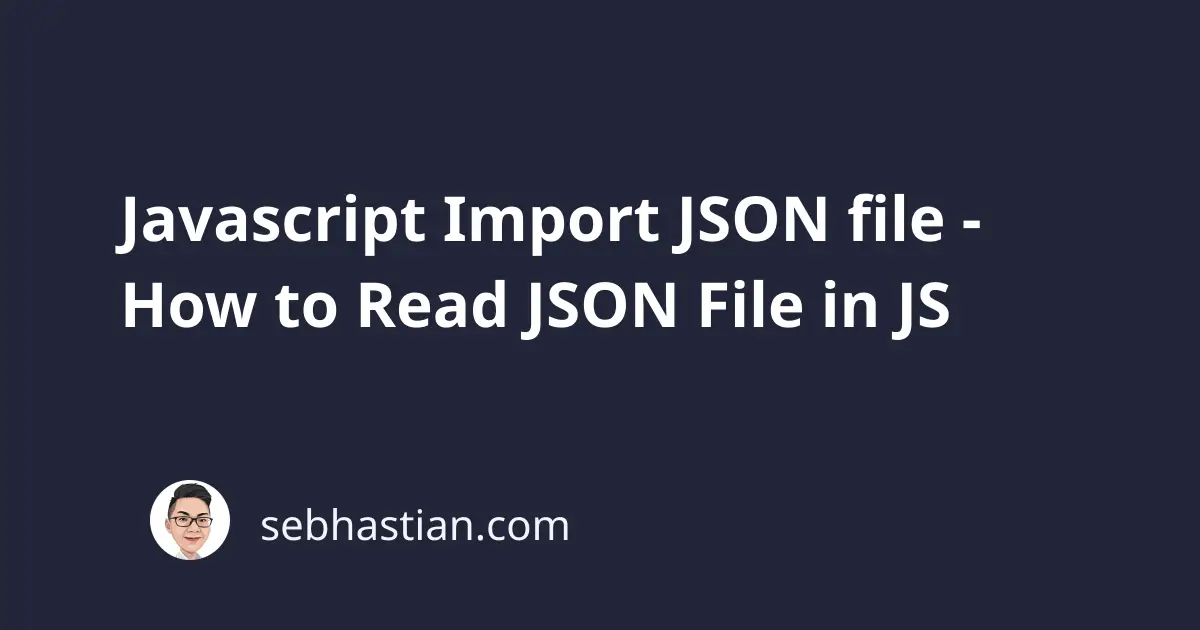
When developing web applications, there are times when you need to import and read a JSON file in your application.
Depending on where you store the JSON file, there are several provided solutions you can use to import and read a JSON file:
- Using the fetch API
- Using the import statement
- Using the require statement
This tutorial will show you how to use the three solutions above in your project.
1. Read JSON File Using the Fetch API
When you have a JSON file uploaded in the same or remote server, you can use the fetch API to retrieve that file.
Suppose you have a JSON file named data.json
with the following contents:
{
"id": 1,
"name": "Nathan Sebhastian",
"isActive": true
}
To fetch the data.json
file, you can use the Fetch API to send an HTTP request. Call the fetch()
method as shown below:
fetch('https://resource.com/data.json')
.then(response => response.json())
.then(json => console.log(json.name)); // Nathan Sebhastian
You need to pass the full URL of the location as an argument to the fetch()
method, then use the then()
method to accept the response from the HTTP request.
From that response, call the json()
method to read the response stream and convert it to a JSON object.
Use the then()
method again to accept the response from json() method
, and then do what you need with the JSON data. Here, we just call the console.log()
method to print the result.
This solution should work when you put the JSON file in a server, whether remote or local. But it will fail if you fetch the data from the filesystem as follows:
fetch('./data.json')
.then(response => response.json())
.then(json => console.log(json.name));
If you run the code above without having a server, then Fetch will use your computer’s filesystem, causing a CORS error as follows:
Also note if you use Fetch API in Node.js, you also get the following error:
TypeError: Failed to parse URL from ./data.json
To fetch a JSON file using Node.js, you need to use the require()
method.
2. Import JSON File Using require()
in Node.js
The require()
method in Node.js can be used to read a JSON file and store the result directly in a variable as follows:
let json = require('./data.json');
console.log(json.name); // Nathan Sebhastian
And that’s it. The require()
method will try to find the JSON file using the filesystem path.
You can perform further manipulation after assigning the result to a variable as shown above.
3. Import JSON File Using import
Another way you can import a JSON file is by using the import
statement, which is supported in both browser and Node.js environment.
In the browser, you need to set the type
attribute of your <script>
tag as module
in the script that’s importing the JSON file:
This is how the script should look like in your <body>
tag:
<body>
<div>Importing JSON</div>
<script type="module" src="index.js"></script>
</body>
Inside the index.js
file, here’s how you use the import
syntax:
import json from './data.json' assert {type: 'json'};
console.log(json.name); // Nathan Sebhastian
console.log(json.isActive); // true
The assert
statement is used to tell the browser that you’re expecting a MIME type of “application/json” from the import result.
If you don’t add the assert
statement, then you might have an error as follows:
If you got an error when using the assert
statement, try to use the with
statement instead:
import json from './data.json' with {type: 'json'};
This is because there’s a proposal from JavaScript maintainers to change the assert
keyword back to with
.
Right now, the assert
statement would still work, but it might be deprecated in the future.
Now if you use the import
statement in Node.js, you need to add the type
property to the package.json
file:
{
"name": "n-app",
"version": "1.0.0",
"type": "module"
}
And that’s how you import a JSON file using JavaScript, both in the browser and Node.js environment.
Here are some JavaScript related articles that I’ve written previously:
- SyntaxError: Cannot use import statement outside a module
- How to fix error:0308010C:digital envelope routines::unsupported on NodeJS
- How do disable a button using JavaScript
I hope these articles help. Happy coding!