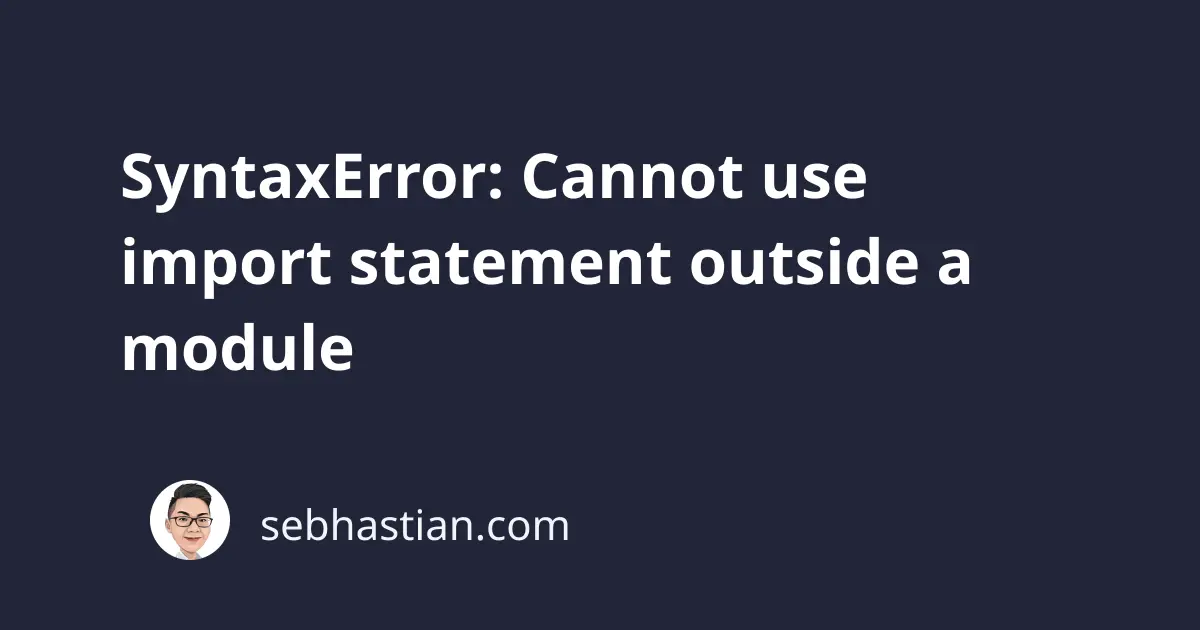
One error that you might encounter when working with JavaScript is:
SyntaxError: Cannot use import statement outside a module
This error occurs when you use the import
keyword in an environment that doesn’t support the ES modules syntax.
This tutorial shows an example that causes this error and how to fix it.
How to reproduce this error
Suppose you’re developing a JavaScript web application using the Express framework.
You used the import
statement to get the express
package and use it in your source code as follows:
import express from 'express'
const app = express()
const port = 3000
app.listen(3000, () => {
console.log(`Example app listening on port ${port}`)
})
You saved the code as index.js
and then run it using the node index.js
command.
The terminal output shows an error:
/n-app/index.js:1
import express from "express"
^^^^^^
SyntaxError: Cannot use import statement outside a module
This error occurs because Node.js doesn’t support the ES modules import/export syntax by default.
Instead, Node.js used the CommonJS syntax as follows:
const express = require('express')
If you replaced the import
statement with require
, the error should disappear and your Express application should run.
How to fix this error
To resolve this error and keep using the import
statement in your code, you need to modify your package.json
file and define the type
property as module
like this:
{
"name": "n-app",
"version": "1.0.0",
"main": "index.js",
"type": "module",
// ...
}
The type: module
tells Node.js that the JavaScript modules are loaded using the ES modules syntax. With that, you can use the import
statement in your source code.
But keep in mind that this prevents you from using the CommonJS modules syntax. Node.js only allows you to run one type of the modules syntax to prevent confusion and inconsistencies.
This error can also occur when you’re running JavaScript code in the browser.
To enable the ES modules syntax on the browser, you need to add the type="module"
attribute to your <script>
tag as follows:
<script type="module" src="helper.js"></script>
By adding the attribute, the import
statement should work from the browser.
I hope this tutorial is helpful. See you in other tutorials! 🙌