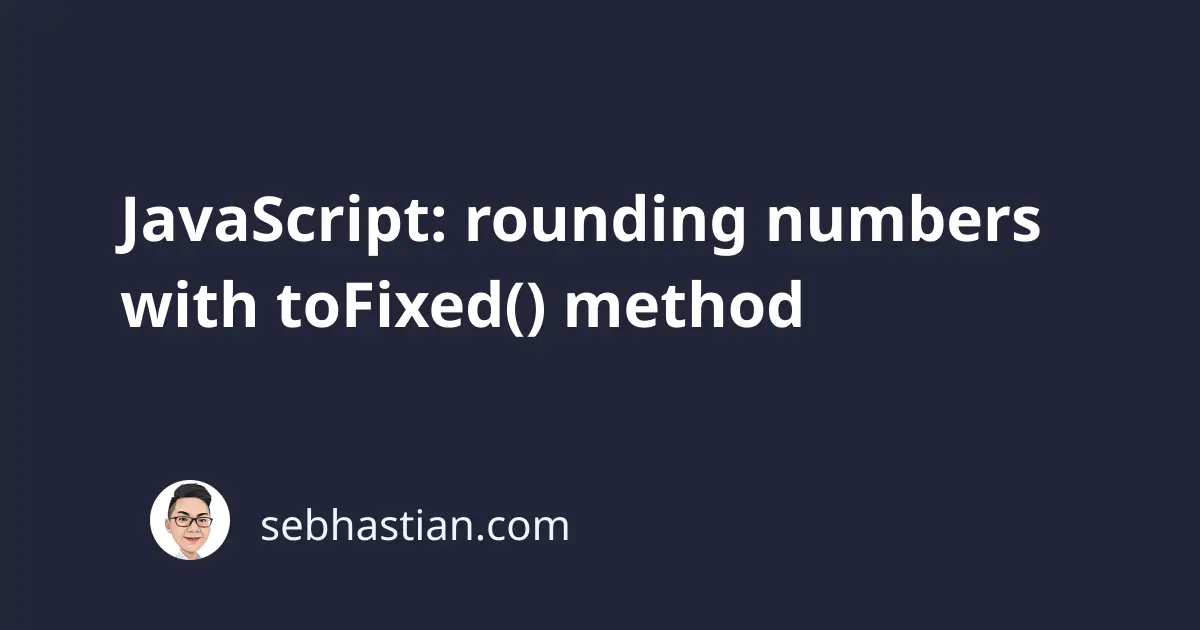
The JavaScript toFixed()
method is a built-in method for Number
objects that allows you to round a number
value that has floating or decimal numbers.
The method accepts one optional parameter, which is the length of decimal values you want to keep for the currently processed number.
Here’s an example:
let num = 34.7698;
let numFixed = num.toFixed();
let numFixedTwo = num.toFixed(2);
console.log(numFixed); // "35"
console.log(numFixedTwo); // "34.77"
Now one weird behavior of this method is that although it can only be called on a number
type value, it will return a string
instead of a number
back to you:
let num = 34.7698;
let numFixed = num.toFixed();
console.log(typeof num); // "number"
console.log(typeof numFixed); // "string"
If you need to have the value returned as a number
, you need to convert it back using the parseFloat()
function.
Here’s an example of that:
let num = 34.7698;
let numFixedTwo = parseFloat(num.toFixed(2));
console.log(numFixedTwo); // 34.77
console.log(typeof numFixedTwo); // "number"
You only need to use the toFixed()
method when you want to keep the decimal length to a certain desired length. If you want to round the number to zero decimal length, it’s better to use the built-in Math
object methods for rounding:
Feel free to choose which method works best for your situation.