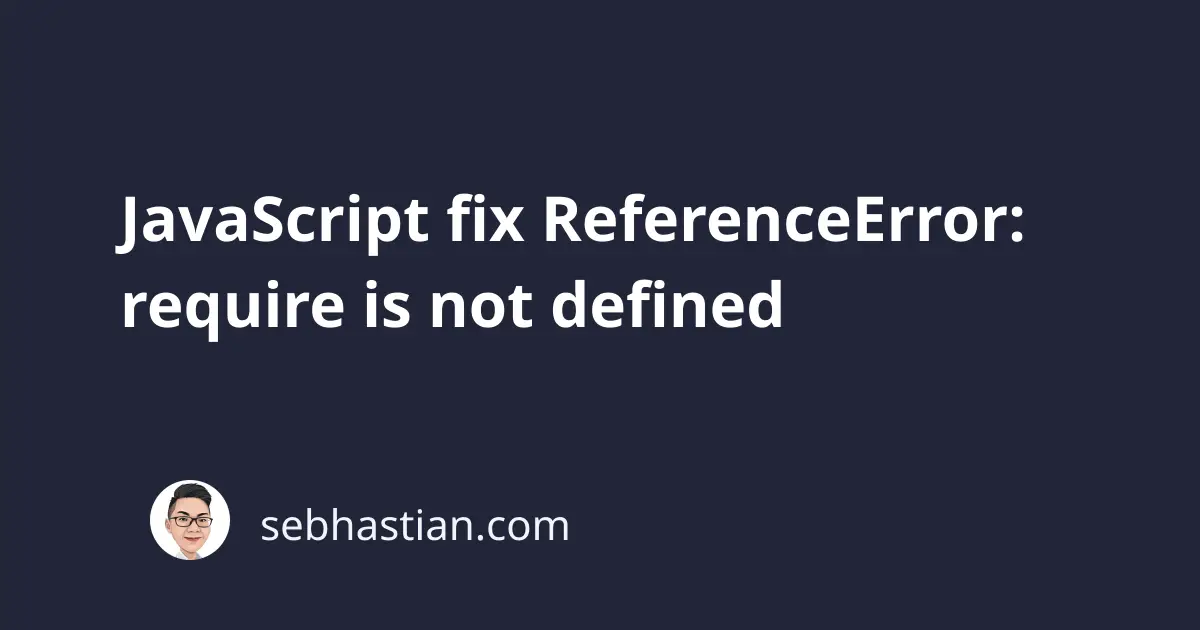
The ReferenceError: require is not defined
error means the require
function is not available under the current JavaScript environment.
The JavaScript environment is the platform or runtime in which JavaScript code is executed.
This can be a web browser, a Node.js server, or other environments such as embedded systems and mobile devices.
Here’s an example code that triggers this error:
const axios = require("axios");
// ReferenceError: require is not defined
This error occurs because JavaScript doesn’t understand how to handle the call to the require
function.
To fix this error, you need to make sure that the require
function is available under your JavaScript environment.
The availability of the require
function depends on whether you run JavaScript from the browser or Node.js.
Consequently, there are several solutions you can use to fix this error:
The following article shows how you can fix the error.
Fix require is not defined in the browser
The JavaScript require()
function is only available by default in Node.js environment.
This means the browser doesn’t know what to do with the require()
call in your code.
But require()
is actually not needed because browsers automatically load all the <script>
files you added to your HTML file.
For example, suppose you have a lib.js
file with the following code:
function hello() {
alert("Hello World!");
}
Then you add it to your HTML file as follows:
<body>
<!-- 👇 add a script -->
<script src="lib.js"></script>
</body>
The function hello()
is already loaded just like that. You can call the hello()
function anytime after the <script>
tag above.
Here’s an example:
<body>
<script src="lib.js"></script>
<!-- 👇 call the lib.js function -->
<script>
hello();
</script>
</body>
A browser load all your <script>
tags from top to bottom.
After a <script>
tag has been loaded, you can call its code from anywhere outside of it.
Server-side environments like Node.js doesn’t have the <script>
tag, so it needs the require()
function.
Use ESM import/ export syntax
If you need a require-like syntax in the browser, then you can use the ESM import/export syntax.
Modern browsers like Chrome, Safari, and Firefox support import/export syntax when you load a script with module
type.
First, you need to create exports in your script. Suppose you have a helper.js
file with an exported function as follows:
function greetings() {
alert("Using ESM import/export syntax");
}
export { greetings };
The exported function can then be imported into another script.
Create an HTML file and load the script, then add the type
attribute as shown below:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
</head>
<body>
<!-- 👇 don't forget the type="module" attribute -->
<script type="module" src="helper.js"></script>
<script type="module" src="process.js"></script>
</body>
</html>
In the process.js
file, you can import the helper.js
file:
import { greetings } from "./helper.js";
greetings();
You should see an alert box called from the process.js
file.
You can also use the import
statement right in the HTML file like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
</head>
<body>
<script type="module" src="helper.js"></script>
<!-- 👇 import here -->
<script type="module">
import { greetings } from "./helper.js";
greetings();
</script>
</body>
</html>
Keep in mind that old browsers like Internet Explorer might respond with an error if you have ESM import/export syntax as shown above.
Using RequireJS on the browser.
If you want to use the require()
function in a browser, then you need to add RequireJS to your script.
RequireJS is a module loader library for in-browser use. To add it to your project, you need to download the latest RequireJS release and put it in your scripts/
folder.
Next, you need to call the script on your main HTML header as follows:
<!DOCTYPE html>
<html>
<head>
<title>RequireJS Tutorial</title>
<script data-main="scripts/app" src="scripts/require.js"></script>
</head>
<body>
<h1 id="header">My Sample Project</h1>
</body>
</html>
The data-main
attribute is a special attribute that’s used by RequireJS to load a specific script right after RequireJS
is loaded. In case of the above, scripts/app.js
file will be loaded.
You can load any script you need to use inside the app.js
file.
Suppose you need to include the Lodash library in your file. You first need to download the script from the website, then include the lodash.js
script in the scripts/
folder.
Your project structure should look as follows:
├── index.html
└── scripts
├── app.js
├── lodash.js
└── require.js
Now all you need to do is use requirejs
function to load lodash
, then pass it to the callback function.
Take a look at the following example:
requirejs(["lodash"], function (lodash) {
const headerEl = document.getElementById("header");
headerEl.textContent = lodash.upperCase("hello world");
});
The code above shows how to use RequireJS to load the lodash
library.
Once it’s loaded, the <h1>
element will be selected, and the textContent
will be assigned as “hello world” text that is transformed to uppercase by lodash.uppercase()
call.
You can wait until the whole DOM is loaded before loading scripts by listening to DOMContentLoaded
event as follows:
document.addEventListener("DOMContentLoaded", function () {
requirejs(["lodash"], function (lodash) {
const headerEl = document.getElementById("header");
headerEl.textContent = lodash.upperCase("hello world");
});
});
Finally, you may also remove the data-main
attribute and add the <script>
tag right at the end of the <body>
tag as follows:
<!DOCTYPE html>
<html>
<head>
<title>RequireJS Tutorial</title>
<script src="scripts/require.js"></script>
</head>
<body>
<h1 id="header">My Sample Project</h1>
<script>
document.addEventListener("DOMContentLoaded", function () {
requirejs(["scripts/lodash"], function (lodash) {
const headerEl = document.getElementById("header");
headerEl.textContent = lodash.upperCase("hello world");
});
});
</script>
</body>
</html>
Feel free to restructure your script to meet your project requirements.
You can download an example code on this requirejs-starter repository at GitHub.
Now you’ve learned how to use RequireJS in a browser. Personally, I think it’s far easier to use ESM import/export syntax because popular browsers already support it by default.
Next, let’s see how to fix the error on the server side.
Node application type is set to module in package.json file
The require()
function is available in Node.js by default, so you only need to specify the library you want to load as its argument.
For example, here’s how to load the lodash
library from Node:
// 👇 load library from node_modules
const lodash = require("lodash");
// 👇 load your local exported modules
const { greetings } = require("./helper");
But even when you are running the code using Node, the require is not defined
error may occur because of your configurations.
Here’s the error logged on the console:
$ node index.js
file:///DEV/n-app/index.js:1
const { greetings } = require("./helper");
^
ReferenceError: require is not defined
If this happens to you, then the first thing to do is check your package.json
file.
See if you have a type: module defined in your JSON file as shown below:
{
"name": "n-app",
"version": "1.0.0",
"type": "module"
}
The module
type is used to make Node treat .js
files as ES modules. Instead of require()
, you need to use the import/export syntax.
To fix the error, remove the "type": "module"
from your package.json
file.
Using .mjs extension when running Node application
If you still see the error, then make sure that you are using .js
extension for your JavaScript files.
Node supports two JavaScript extensions: .mjs
and .cjs
extensions.
These extensions cause Node to run a file as either ES Module or CommonJS Module.
When using the .mjs
extension, Node will not be able to load the module using require()
. This is why you need to make sure you are using .js
extension.
Conclusion
By default, the require
function is not available in the browser environment.
But even when you run JavaScript code from Node.js, you can still see this error if you don’t set the proper configuration.
The solutions provided in this article should help you fix ReferenceError: require is not defined
in both server and browser environments.
I’ve also written several other common JavaScript errors and how to fix them:
- How to fix JavaScript unexpected token error
- How to fix JavaScript function is not defined error
- Fixing JavaScript runtime error: $ is undefined
These articles should help you become better at debugging JavaScript errors.
That’s all for this article. Happy coding! 🙏