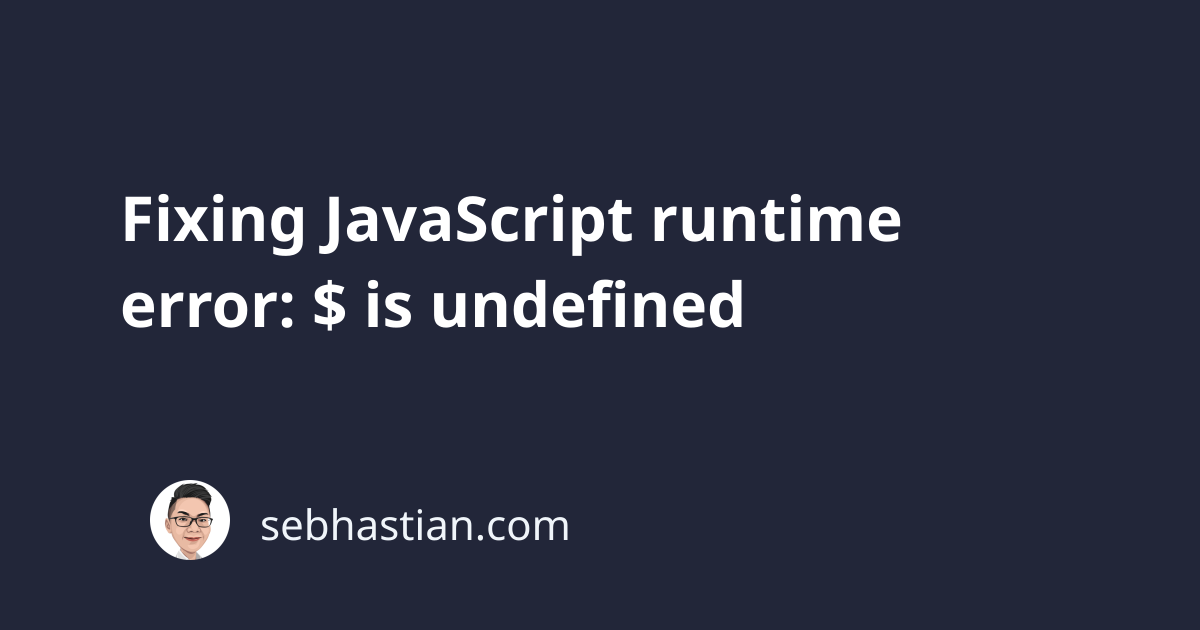
Sometimes, you may saw a JavaScript error that says $ is undefined
:
JavaScript runtime error: '$' is undefined
This error happens when your code tries to run a jQuery script, but the script is not yet downloaded. The $
symbol is used by jQuery for selecting elements. You probably have code in your project that looks as follows:
$(document).ready(function () {
$(".btn-hidden").hide();
alert("Button is hidden!");
});
To fix the error, you need to add jQuery script to your application. The easiest way to add jQuery is to add the <script>
tag for jQuery library in your HTML <head>
tag:
<head>
<meta charset="utf-8">
<title>jQuery download</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
You can download any version of jQuery you need at code.jquery.com or just copy the <script>
tag above.
If you already have jQuery in your application, make sure that the <script>
tag is downloaded before your code is executed.
The following example still causes the error because jQuery is downloaded after the $
symbol is executed:
<script>
$(document).ready(function () { // $ is undefined
$(".btn-hidden").hide();
});
</script>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
You just need to reverse the <script>
order as follows:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function () {
$(".btn-hidden").hide();
});
</script>
And that’s how you can fix $ is undefined
error.