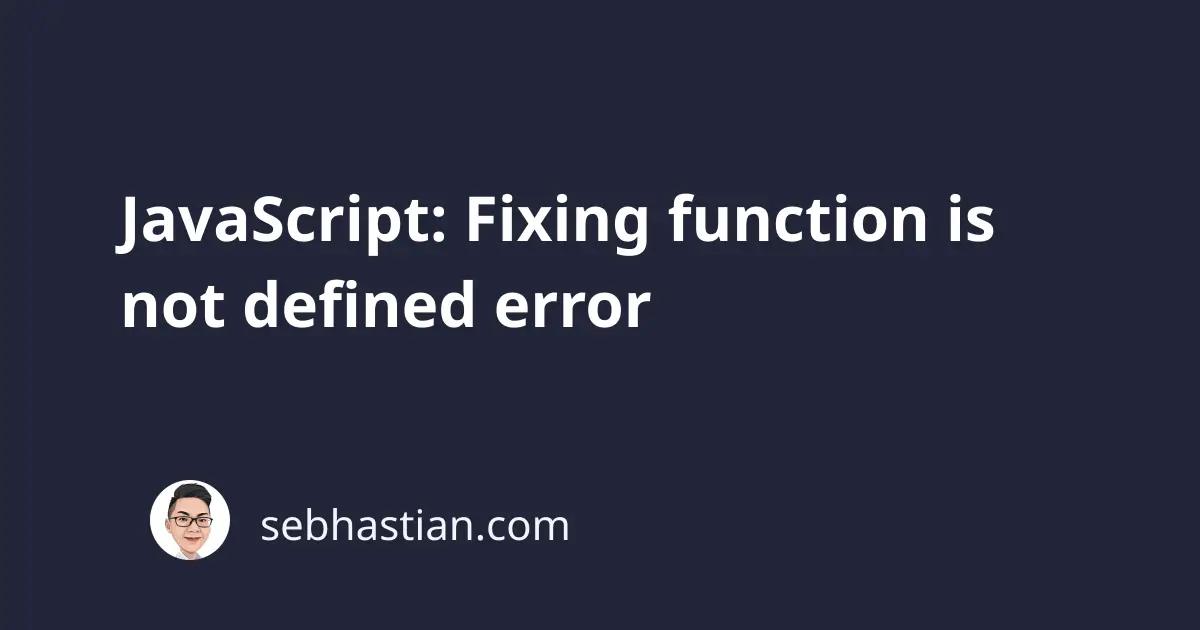
Sometimes, you may come across JavaScript function is not defined error that looks like the following:
doAction();
Uncaught ReferenceError: doAction is not defined
The ReferenceError
as in the case above is caused when you call something that’s not defined in JavaScript. Let me show you several things you can do to fix the error.
Make sure the function is defined inside your script
One of the small mistakes that could cause the error is that you haven’t defined the function properly. You need to define the function using either the function
keyword:
function doAction() {
// your function code here
}
Or using the arrow function syntax as follows:
const doAction = () => {
// your function code here
};
Please keep in mind that functions defined through function expressions must be defined before the call. Function expressions are functions that you defined through a variable keyword as follows:
var fnAction = function () {};
// or
let fnAction = () => {};
From the example code above, the variable fnAction
will be hoisted, but the function declaration is not, so it will be undefined as shown below:
fnAction(); // fnAction is not defined
let fnAction = () => {
console.log("Executing action");
};
See also: JavaScript hoisting behavior
That’s why it’s always better to define the function before calling it.
When you have defined the function, try calling it immediately below the declaration to see if it works:
function doAction() {
// your function code here
}
doAction(); // test the function call
If it’s running without any error, then you may have several lines of code after the declaration that causes the script to malfunction.
Make sure the entire script has no error
If you’re putting the function into a script and calling it from an HTML tag, you need to make sure that the entire script has no error or the function won’t be loaded.
For example, notice how there is an extra )
right next to getElementById
call:
<!DOCTYPE html>
<html>
<head>
<title>Function not defined</title>
</head>
<body>
<button id="action" onclick="fnAction()">Click me</button>
<script type="text/javascript">
document.getElementById("action")); // extra ')' here
function fnAction(){
console.log("Executing action");
}
</script>
</body>
</html>
Although there’s no error on the fnAction()
code, an error in any part of the script will cause the browser to ignore the rest of that script. You need to fix the error first so the rest of the code can be executed by the browser.
One way to see if you have any error is to run the HTML page and check on the console as follows:
You may find the ReferenceError
fixed itself as you fix JavaScript errors from your scripts.
Make sure the script is loaded before the call.
Finally, the function is not defined error can also be caused by calling the function before the script is loaded to the browser. Suppose you have a JavaScript file separated from your HTML file as follows:
// script.js
function fnAction() {
console.log("executing action");
}
Then you load the script into your HTML file, but you call the fnAction
function before you load the script as follows:
<body>
<h1>Load script demo</h1>
<script type="text/javascript">
fnAction();
// Uncaught ReferenceError: fnAction is not defined
</script>
<script src="script.js"></script>
</body>
The same also happens when you call it on the <head>
tag:
<head>
<title>Function not defined</title>
<script type="text/javascript">
fnAction();
</script>
<script src="script.js"></script>
</head>
To fix this, you need to call the function below the <script src="script.js"></script>
call:
<head>
<title>Function not defined</title>
<script src="script.js"></script>
<script type="text/javascript">
fnAction();
</script>
</head>
Those are the three ways you can try to fix function is not defined error in JavaScript.