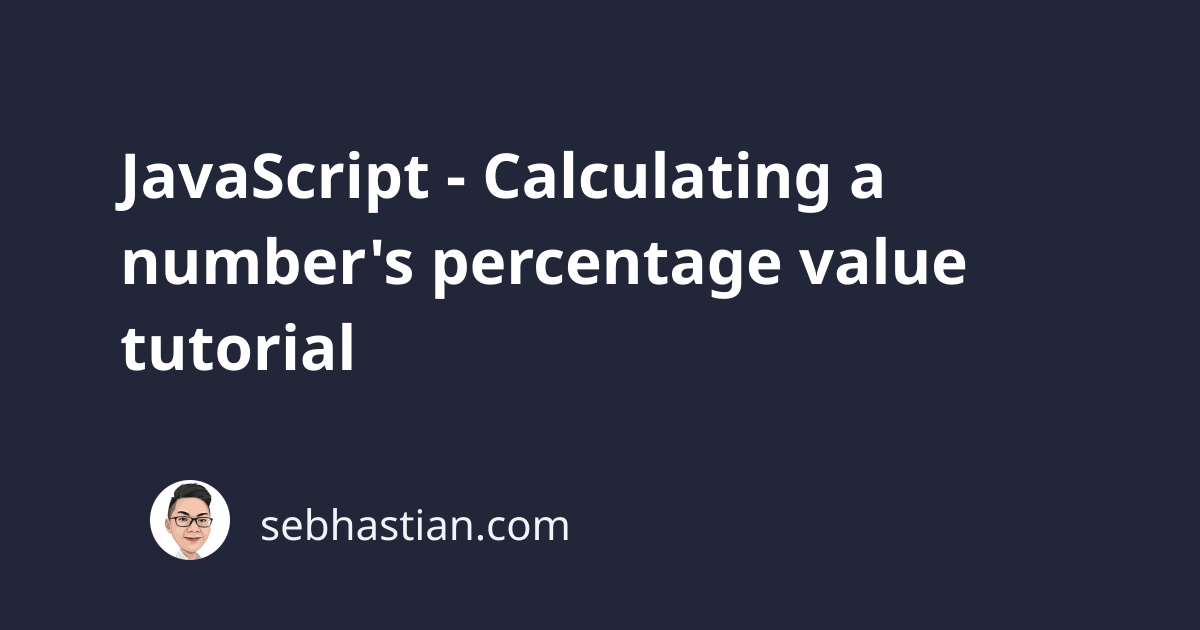
The JavaScript Math
object doesn’t have any built-in method to calculate the percentage of a number, so if you want to find out the percentage of a specific number, you need to create your own custom function.
For example, the code below will find what 20% of 250 is using the calcPercent()
function:
const percentVal = calcPercent(250, 20);
console.log(percentVal); // 50
To write the calcPercent()
code, we need to first understand the formula to calculate the percentage number.
To find 20% of 250, you need to divide 20
by 100 and then multiply it by 250
:
const num = 250;
const percentage = 20;
const percentVal = 250 * (20 / 100);
console.log(percentVal); // 50
Now that you know the formula for calculating percentages, you can extract the code as a helper function:
function calcPercent(num, percentage){
return num * (percentage / 100);
}
Now you can call the helper function calcPercent()
anytime you need to calculate a number’s percentage value:
function calcPercent(num, percentage){
return num * (percentage / 100);
}
let percentVal = calcPercent(250, 20);
console.log(percentVal); // 50
percentVal = calcPercent(210, 10);
console.log(percentVal); // 21
Rounding the result to 2 decimals
Sometimes, a percentage calculation may return a floating number as follows:
const percentVal = calcPercent(20, 3.33);
console.log(percentVal); // 0.666
If you need to round the number to 2 decimals, then you can use a combination of toFixed()
and parseFloat()
methods to return the number in 2-decimal format.
See also: Rounding numbers with toFixed() method
Here’s the full function code:
function calcPercent(num, percentage){
const result = num * (percentage / 100);
return parseFloat(result.toFixed(2));
}
const percentVal = calcPercent(20, 3.33);
console.log(percentVal); // 0.67
Instead of returning 0.666
, the function will return 0.67
because it rounds the decimal numbers to a maximum length of two.