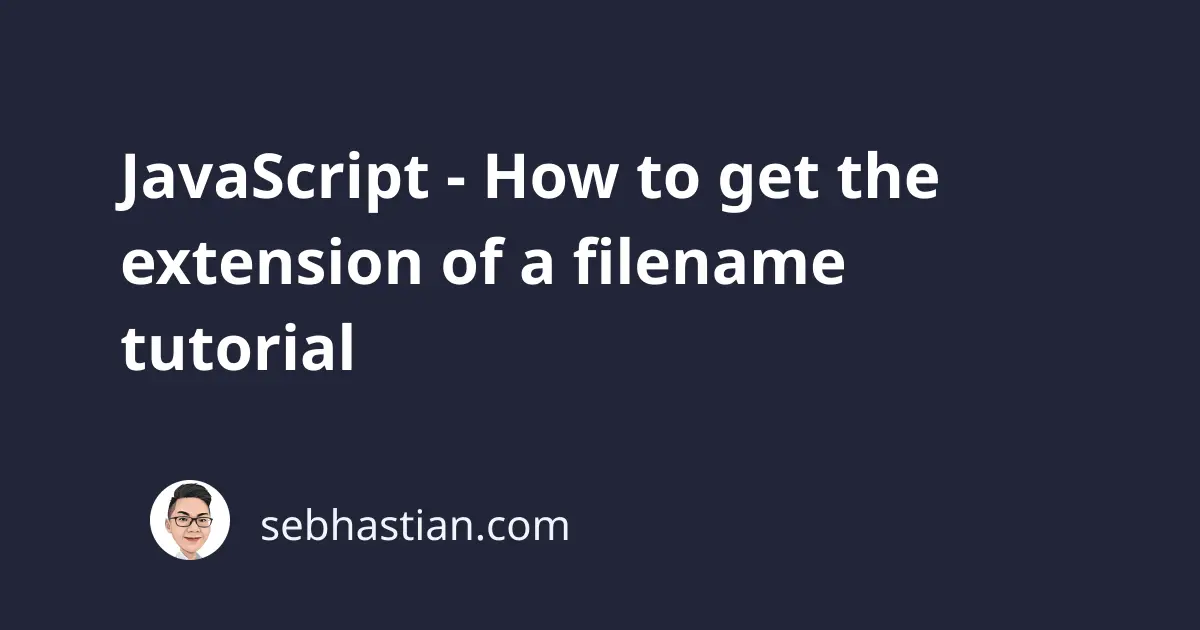
Sometimes, you need to find a file’s extension so you can properly handle the file in your code.
There are two ways you can get a filename’s extension:
- Using a combination of the
split()
andpop()
methods - Using a combination of
substring()
andlastIndexOf()
methods.
This tutorial will explain both ways, starting from using split()
and pop()
methods.
Get filename extension using split() and pop() methods
To get a filename extension, you can use a combination of split()
and pop()
methods.
The split()
method will convert a string
into an array
of substrings, separated by the character you passed as the method’s parameter.
Here’s an example of the split()
method in action:
let filename = "index.js";
let arr = filename.split(".");
console.log(arr); // ["index", "js"]
Next, the pop()
method will remove the last element of an array and return that as a value. You can assign the result of calling the pop()
method into a variable as follows:
let arr = ["index", "js"];
let extension = arr.pop();
console.log(arr); // ["index"]
console.log(extension); // "js"
Now that you know how the two methods work, you can chain call the methods to get the extension of a filename as follows:
let filename = "index.js";
let extension = filename.split(".").pop();
console.log(extension); // "js"
And that’s how you can get the file extension from a filename.
To avoid repeating the code each time you need to extract the extension, you can create a small function called getExtension()
that receives one filename
parameter as string
and return
the only the extension as follows:
function getExtension(filename) {
return filename.split(".").pop();
}
let filename = "main.config.js";
let extension = getExtension(filename);
console.log(extension); // "js"
let photoname = "photo.jpg";
let photoExtension = getExtension(photoname);
console.log(photoExtension); // "jpg"
The method also works well when receiving a file from <input>
HTML element as shown below:
<body>
<h1>Get file extension</h1>
<p>Get the extension of a file from user upload</p>
<form>
<input type="file" id="myFile" />
<button id="checkExt">Check extension</button>
</form>
<script>
let button = document.getElementById("checkExt");
function checkExtension() {
let filename = document.querySelector("#myFile").value;
let extension = filename.split(".").pop();
document.write(`The file extension is: ${extension}`);
}
button.addEventListener("click", checkExtension);
</script>
</body>
There’s one more way to extract a file extension, let’s learn how to do that next.
Get filename extension using substring() and lastIndexOf() methods
The substring()
method is a built-in method of the String
object that grabs a part of a string from the start
and end
indices that you specified as its arguments.
Keep in mind that the end
index is excluded from the result, so you need to select the next index of the character you want to include in the substring.
For example, you can extract "ors"
from "Horse"
with the following code:
let animal = "Horse";
let extract = animal.substring(1, 4);
console.log(extract); // ors
A string index starts at 0
so the letter "o"
from "Horse"
will have an index of 1
.
The letter "s"
from "Horse"
has an index value of 3
, so you need to put 4
as the second parameter of the substring()
method.
When you omit the second parameter, the method will return the rest of the string without cutting it:
let animal = "Horse";
let extract = animal.substring(1);
console.log(extract); // orse
Next, the lastIndexOf()
returns the index value of a specified string’s last occurrence. You need to specify what you want to look for as an argument to the method.
For example, here’s how to return the last index of "a"
from "Banana"
:
let fruit = "Banana";
let lastIndex = fruit.lastIndexOf("a");
console.log(lastIndex); // 5
The letter "a"
from the word "Banana"
above has the index value of 1
, 3
, and 5
, so the lastIndexOf()
method returns only the last one, which is 5
.
Knowing how the two methods work, you can call the lastIndexOf()
method to get the index position of the last dot .
symbol in a file name, then call the substring()
method to extract the extension.
Here’s an example:
let filename = "presentation.pptx";
let lastIndex = filename.lastIndexOf(".");
let extension = filename.substring(lastIndex + 1);
console.log(extension); // "pptx"
To make your code more concise, you can call both methods in one line as shown below:
let filename = "presentation.pptx";
let extension = filename.substring(filename.lastIndexOf(".") + 1);
console.log(extension); // "pptx"
And thats how you can extract a file extension using substring()
and lastIndexOf()
methods.
Just like with split()
and pop()
methods, you can write a small helper function to get the extension so you don’t have to repeat yourself each time you need it:
function getExtension(filename) {
return filename.substring(filename.lastIndexOf(".") + 1);
}
let filename = "main.config.js";
let extension = getExtension(filename);
console.log(extension); // "js"
let photoname = "photo.jpg";
let photoExtension = getExtension(photoname);
console.log(photoExtension); // "jpg"
Any time you need to grab the extension, just call on the getExtension()
method as shown above 😉