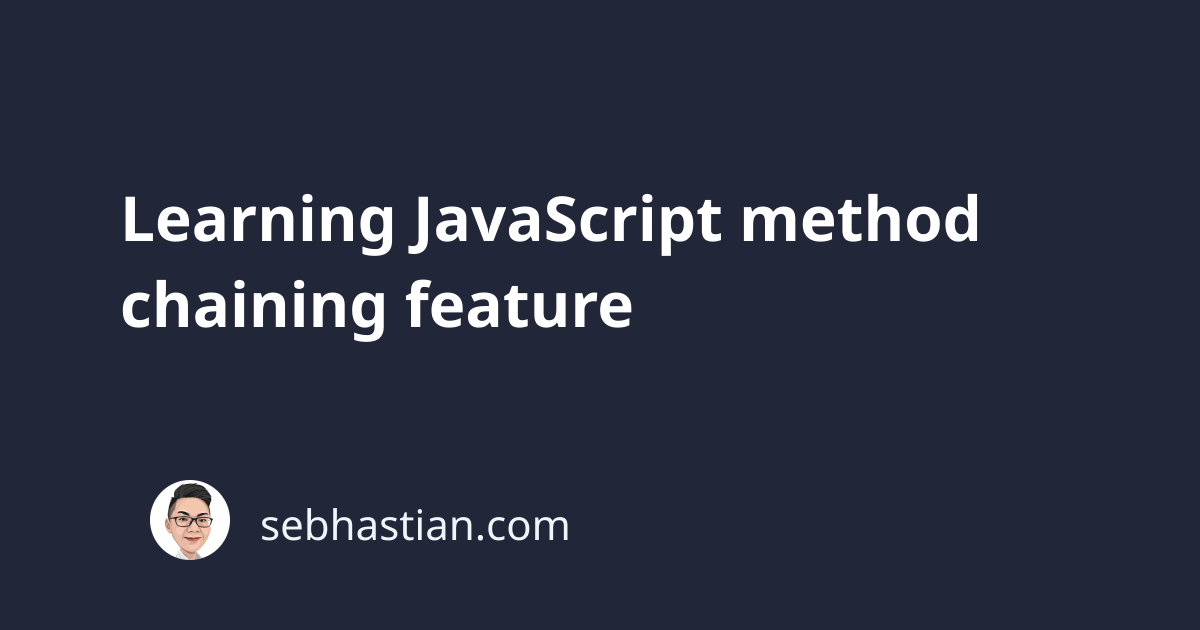
Method chaining allows you to sequentially call functions on the same object or value so that you can achieve your desired result with a cleaner code.
For example, the following JavaScript code transforms the string into lower case and then split the words into an array:
let myString = "HELLO WORLD";
let lcString = myString.toLowerCase(); // hello world
let words = lcString.split(" "); // ["hello", "world"]
Although the code above is working fine, you can write less code using the method chaining technique as follows:
let myString = "HELLO WORLD";
let words = myString.toLowerCase().split(" ");
The above code is an example of method chaining. Although myString
variable is actually a primitive string, JavaScript automatically converts primitives to String
objects, so you can call String
object methods on it.
You can use the chaining method technique when you create either an object or a class. The trick is that you need to return this
for each of your functions because this
refers to the object in which you call the function.
Here’s an example of a custom cat
object with method chaining enabled:
const cat = {
activity: null,
walk: function () {
this.activity = "walking";
console.log("Cat is walking");
return this;
},
eat: function () {
this.activity = "eating";
console.log("Cat is eating");
return this;
},
};
You can then chain the method call of cat
object as follows:
cat.walk().eat();
Because cat.walk()
returns this
, the next call will be this.eat()
with this
referring to the cat
object. The same thing happens when you call methods from the String
or Array
object. The this
keyword will refer to the string or array value that has the methods available.