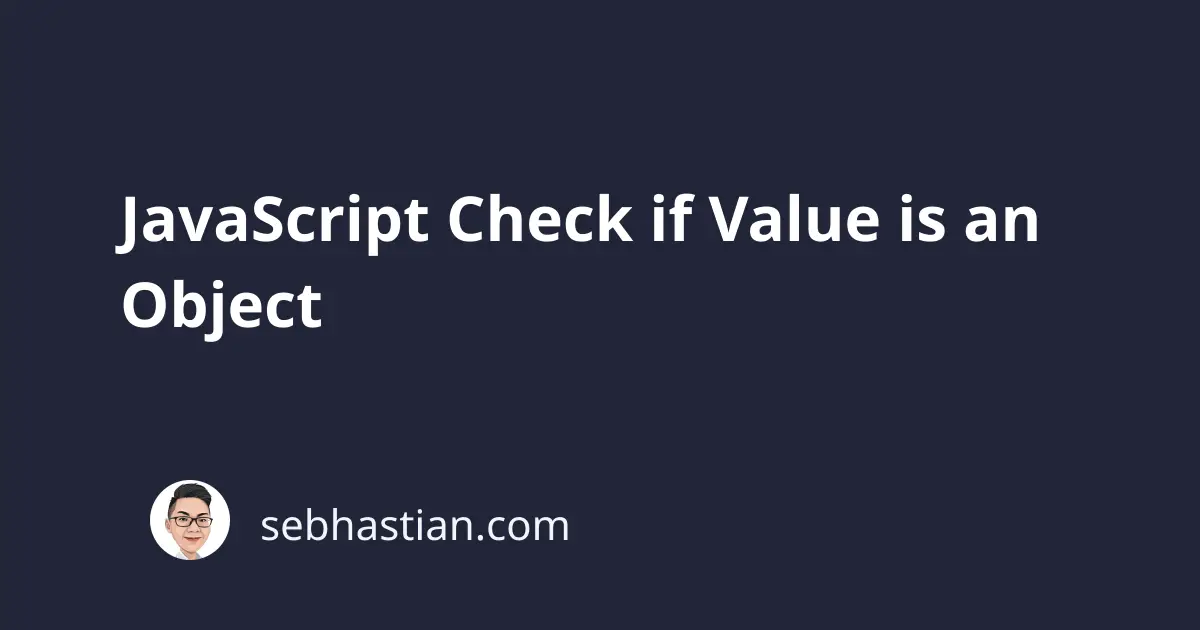
Hi friends! Today I’m going to show you how to check if the value of a variable is an Object using JavaScript.
At first glance, it might look easy as we can just use the typeof
operator and see if the returned value is equal to ‘object’ as follows:
const myObj = {};
console.log(typeof myObj === 'object'); // true
But the problem here is that array and null
values are also an object to JavaScript, so you don’t get an accurate result.
If you check the type of these values and compare them to the ‘object’ string, both returns true
:
console.log(typeof [] === 'object'); // true
console.log(typeof null === 'object'); // true
To handle this problem, we need to use the logical AND &&
operator and check 3 conditions together.
Use the Array.isArray()
method to check if the value is not an array by adding the logical NOT !
operator in front of the method, and check that the value is not a null
as well.
You can create a custom function called isObject()
to make things easier. Anytime you want to check a value, just call the function:
function isObject(val) {
return typeof val === 'object' && !Array.isArray(val) && val !== null;
}
console.log(isObject({})); // true
console.log(isObject([])); // false
console.log(isObject(null)); // false
But note that if you pass a Date
object, then the isObject
function will return true
because Date
is also an object in JavaScript.
If you don’t want to include anything but a literal object, then you need to use the Object.prototype.toString.call()
method instead.
Here’s how it works: The Object.prototype.toString.call()
method returns a string containing the type of the value. If you pass an object, it will return ‘[object Object]’, if you pass a null it will be ‘[object Date]’, an array would be ‘[object Array]’ and so on.
Replace the isObject()
function content as follows:
function isObject(val) {
return Object.prototype.toString.call(val) === '[object Object]';
}
console.log(isObject({})); // true
console.log(isObject([])); // false
console.log(isObject(null)); // false
console.log(isObject(new Date())); // false
Now anything but a literal object would result in a false
.
And that’s how you check if a JavaScript value is an object or not. You can edit the code to fit your specific requirements, but the example should be enough for most common cases.
I have more articles related to JavaScript object here:
How to Find a JavaScript Object Length
Check if an Object is Empty in JavaScript
Printing JavaScript Object
I’m sure these tutorials will help you in your daily work with JavaScript object.
That will be all for today, see you in other tutorials! 👋