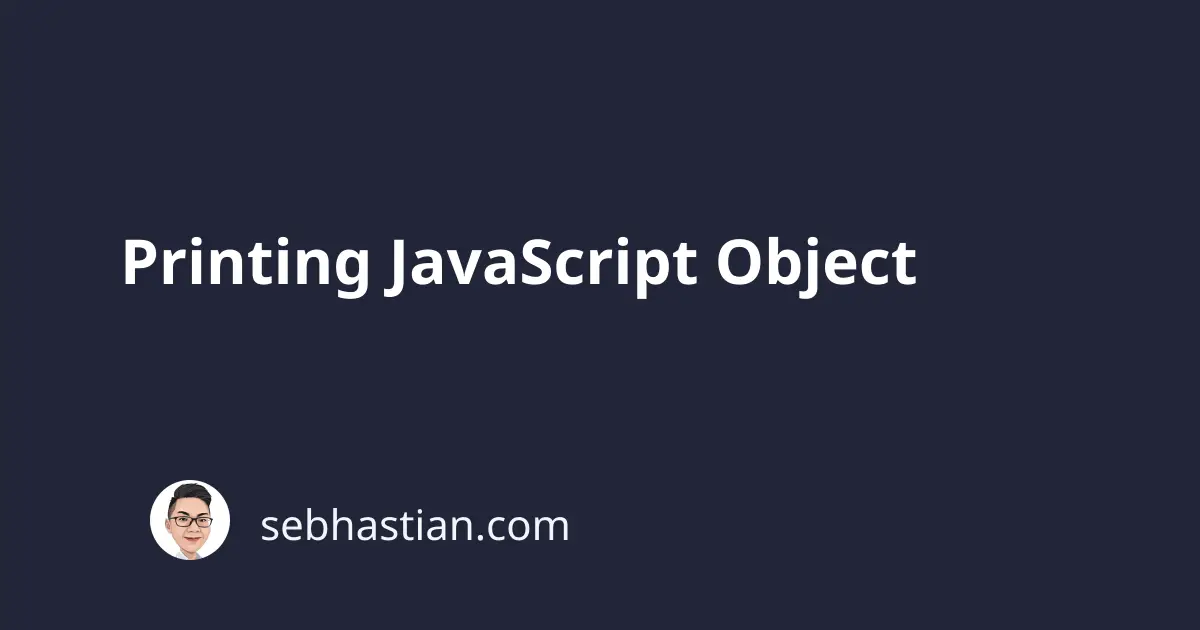
Sometimes, JavaScript’s console.log()
output for an Object
variable may return the "[object Object]"
instead of displaying the actual properties and values of the object. It usually occurs when you log an HTTP request-response object or using the alert()
method to print an object:
let myObj = { name: "Nathan", age: 29 };
alert(myObj); // [object Object]
This is because myObj
is transformed into a string
by the alert()
method. To actually print the properties and values of myObj
, you need to use the JSON.stringify()
method so that myObj
is transformed into its JSON string representation:
let myObj = { name: "Nathan", age: 29 };
alert(JSON.stringify(myObj)); // {"name":"Nathan","age":29}
The same works for a response from HTTP requests. All you need to remember is [object Object]
is a string
representation of your object, so to print the output, you need to call JSON.stringify()
method on the object.