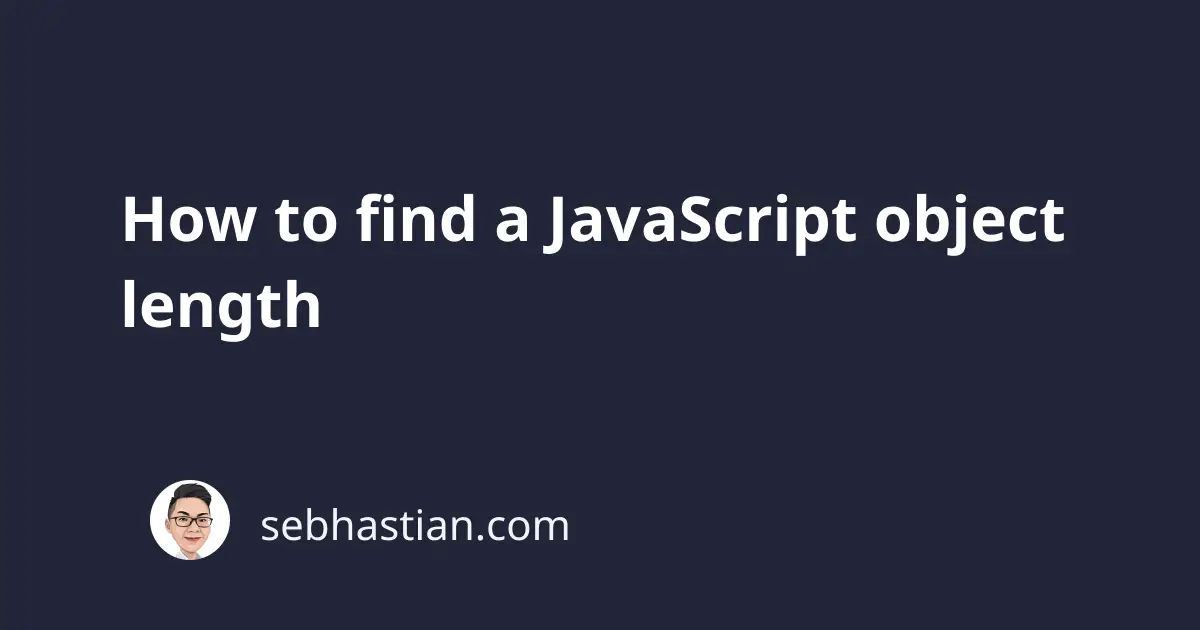
The JavaScript object
type doesn’t have the length
property like in the array
type.
If you need to find out how many properties are defined in one of your objects, you need to use the Object.keys()
method.
The Object.keys()
method returns an array
containing the property name of your object.
You need to pass the object as an argument to the Object.keys()
method as shown below:
const myObj = {
username: "Nathan",
role: "Software developer",
age: 29
}
const keys = Object.keys(myObj);
console.log(keys); // ["username", "role", "age"]
Because the Object.keys()
method returns an array, you can chain the method call with the .length
property to find out how many defined properties your object has:
const myObj = {
username: "Nathan",
role: "Software developer",
age: 29
}
console.log(Object.keys(myObj).length); // 3
However, keep in mind that the Object.keys()
method only works for regular object properties and not Symbol
object properties.
A Symbol
property as in the example below will be excluded from the Object.keys()
method:
const myObj = {
[Symbol("id")]: 7889,
username: "Nathan",
role: "Software developer",
}
console.log(Object.keys(myObj).length); // 2
But this is as it should be since Symbol
type properties are used for adding properties to objects used by other functions or libraries.
By hiding it from functions like Object.keys()
and for..in
statement the Symbol
properties won’t be accidentally processed.
To fetch Symbol
properties, you need to use the Object.getOwnPropertySymbols()
method with returns an array of Symbol
keys:
const myObj = {
[Symbol("id")]: 7889,
[Symbol("username")]: "Nathan",
role: "Software developer",
}
console.log(Object.getOwnPropertySymbols(myObj));
// [Symbol(id), Symbol(username)]
If you want to include all Symbol
properties in your length calculation, you need to add the Object.keys().length
value and the Object.getOwnPropertySymbols().length
value
Here’s an example:
const myObj = {
[Symbol("id")]: 7889,
[Symbol("username")]: "Nathan",
role: "Software developer",
}
const symbolKeysLength = Object.getOwnPropertySymbols(myObj).length; // 2
const keysLength = Object.keys(myObj).length; // 1
const myObjLength = symbolKeysLength + keysLength;
console.log(myObjLength); // 3
And that’s how you can find objects length in JavaScript 😉