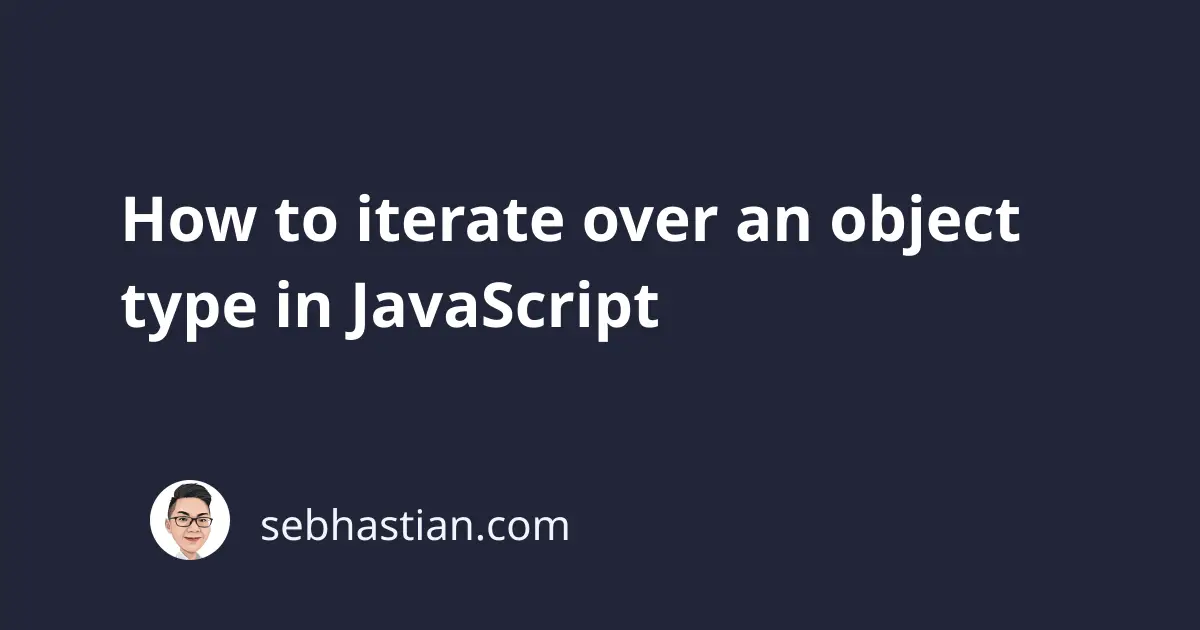
Sometimes, you may need to iterate over an object
type variable in JavaScript. Even though object
and array
type data are considered an object
in JavaScript, you can’t use the methods of JavaScript array
type like map()
and forEach()
to loop over an object
type data.
There are three ways you can iterate over an object in JavaScript:
- Using
for...in
statement - Using
Object.entries()
method andfor...of
statement - Using
Object.keys()
method andfor...of
statement
This tutorial will explain all three ways, starting from using the for...in
statement.
Iterate over object using for…in statement
To iterate over an object
type variable in JavaScript, you need to use the for..in
statement.
The for...in
statement allows you to iterate over the object
property keys, so you can log both the property keys and values.
Here’s an example of for...in
statement in action:
const obj = {
name: "Nathan",
age: 29,
job: "Web developer",
};
for (const key in obj) {
console.log(`${key}: ${obj[key]}`);
}
The output of the code above will be as follows:
name: Nathan
age: 29
job: Web developer
Now that you know how the for...in
statement works, let’s learn how to combine it with Object.entries()
method next.
Iterate over object using Object.entries() method
The Object.entries()
method is a built-in method of the JavaScript Object
class that transforms an object
value into a two-dimensional array.
Here’s how the method works:
const obj = {
name: "Nathan",
age: 29,
job: "Web developer",
};
const objArray = Object.entries(obj);
console.log(objArray);
// [
// [name, Nathan],
// [age, 29],
// [job, Web developer]
// ]
Knowing this, you can use a combination of the for..of
statement with the destructuring assignment to loop over an object as follows:
const obj = {
name: "Nathan",
age: 29,
job: "Web developer",
};
for (const [key, value] of Object.entries(obj)) {
console.log(`${key}: ${value}`);
}
// Output:
// name: Nathan
// age: 29
// job: Web developer
And that’s how you can iterate over an object using the Object.entries()
method.
Iterate over object using Object.keys() method
Aside from Object.entries()
, you can also use the Object.keys()
method, which returns your object
property keys as an array. You can then use the for...of
statement to iterate over the array and log each object
property:
const obj = {
name: "Nathan",
age: 29,
job: "Web developer",
};
const keys = Object.keys(obj);
for (const key of keys) {
console.log(`${key}: ${obj[key]}`);
}
And those are the three methods you can use to iterate over an object
in JavaScript. Feel free to use the method that you liked best 😉