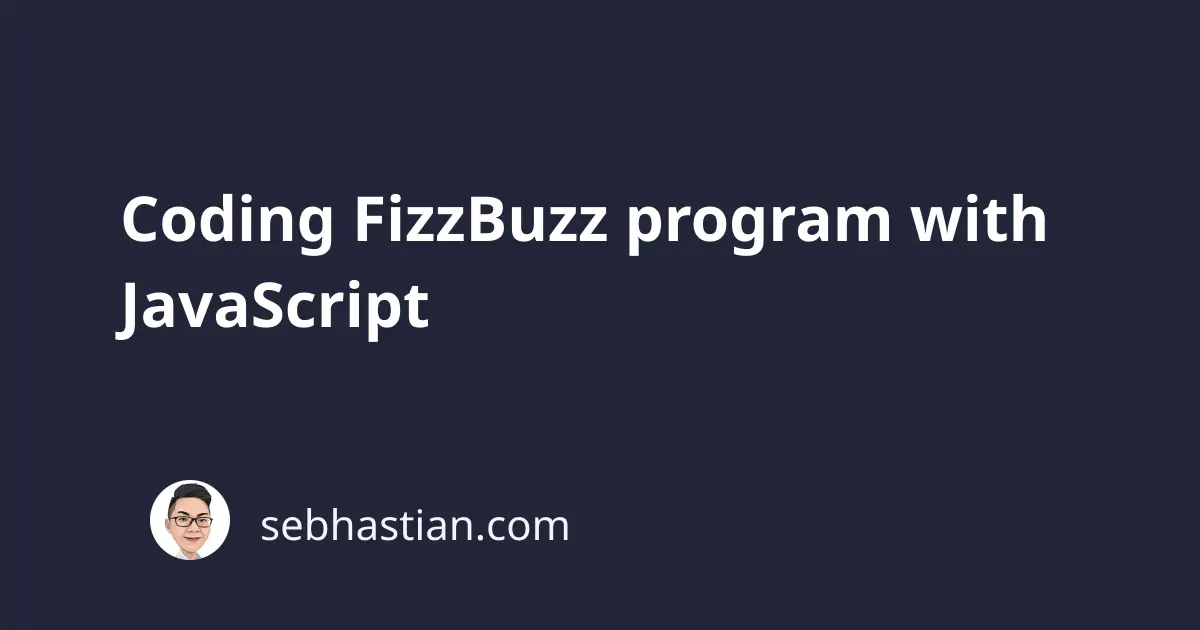
The so called FizzBuzz program is a basic programming exercise commonly used as a job interview question. The program usually comprises of printing a certain set of numbers from 1 to 100, but with a certain twist:
- When the number is a multiples of three, you print
Fizz
instead of the number - When the number is a multiples of five, you print
Buzz
instead of the number - When the number are the multiples of both three and five, you print
FizzBuzz
instead of the number
For example, the result of printing 10 to 15 should look like this:
Buzz # 10
11
Fizz # 12
13
14
FizzBuzz # 15
The easiest way to solve this programming challenge is by using a combination of a for
loop and the conditional if..else
statement.
First, you loop over a certain times as required by the instruction. If you need to loop 100 times, then write the code as follows:
for (var i = 1; i <= 100; i++) {
console.log(i);
}
With the code above, you have printed the numbers 1
to 100
. You just need to create the conditionals where you print "Fizz"
, "Buzz"
, or "FizzBuzz"
.
To look for numbers that are multiples of three, you can check if the current number left no remainder when divided by three. You can do so by using the modulo operator (%
) as follows:
for (var i = 1; i <= 100; i++) {
if (i % 3 === 0) {
console.log("Fizz");
} else {
console.log(i);
}
}
The modulo operator (also known as the remainder operator) will divide the number on the left with the number on the right and return the remaining number left after the division.
For example, the number 5
divided by 3
will left 2
, so 5 % 3 === 2
Now that you’ve figured out the basic pattern of a FizzBuzz program, you just need to add more conditionals to the for
loop. Add the code to check if the number can be divided by 5 by writing i % 5 === 0
:
for (var i = 1; i <= 100; i++) {
if (i % 3 === 0) {
console.log("Fizz");
} else if (i % 5 === 0) {
console.log("Buzz");
} else {
console.log(i);
}
}
Finally, you need to add the code that will check if the number can be divided by both five and three. You can do so by writing the following if
code:
for (var i = 1; i <= 100; i++) {
if (i % 3 == 0 && i % 5 == 0) {
console.log("FizzBuzz");
} else if (i % 3 === 0) {
console.log("Fizz");
} else if (i % 5 === 0) {
console.log("Buzz");
} else {
console.log(i);
}
}
And now you have completed the FizzBuzz program. Try to run the code and you will see the “FizzBuzz” word replacing all the numbers that fulfill the conditional statements.