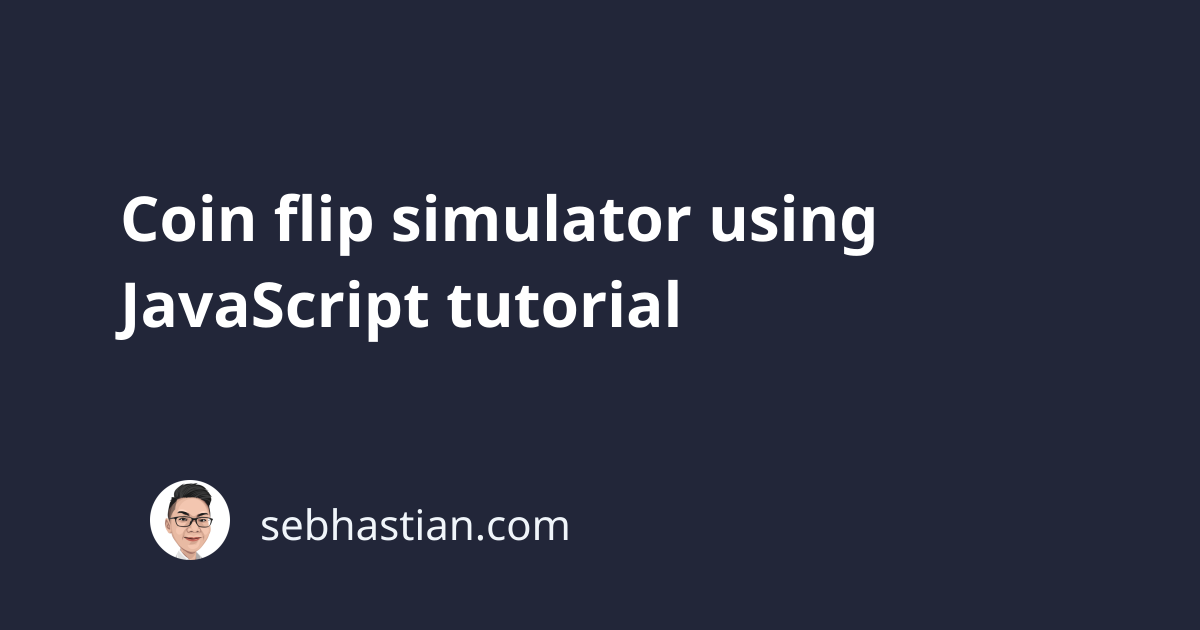
This tutorial will help you to create a coin flip simulator using JavaScript, and HTML. A visual coin flip using CSS is also included.
Simulating a coin flip using JavaScript can be done by using the built-in Math.random()
method.
JavaScript’s Math.random()
method generates a random number between 0
and 0.99999999999999999
so you can use the generated number to decide if the result of the coin flip is a head or a tail.
The breakpoint of the flip is at 0.50
as shown below:
let num = Math.random();
if (num < 0.5) {
console.log("HEAD");
} else {
console.log("TAIL");
}
This means the result will be tail when it’s between 0
and 0.49999999999999999
.
Using the code above, you can create a flip coin simulator that uses HTML <button>
to trigger the JavaScript code and display the result in a <div>
element.
First, create a <button>
and a <div>
with id
attributes inside your HTML element:
<body>
<button id="flip" type="button">Flip the coin</button>
<div id="result"></div>
</body>
Next, add a <script>
tag and attach an event listener to the <button>
element to listen for click
events. When the button is clicked, the fnClick()
function will be executed by JavaScript as follows:
<body>
<button id="flip" type="button">Flip the coin</button>
<div id="result"></div>
<script>
let button = document.getElementById("flip");
function fnClick() {
// TODO: write the function code later
}
button.addEventListener("click", fnClick);
</script>
</body>
The fnClick()
function will run the code to simulate a coin flip by using the Math.random()
method you’ve seen above.
The result will be set as the content of the <div>
element you have in the HTML body:
<body>
<button id="flip" type="button">Flip the coin</button>
<div id="result"></div>
<script>
let button = document.getElementById("flip");
let result = document.getElementById("result");
function fnClick(event) {
let num = Math.random();
if (num < 0.5) {
result.innerHTML = "You got HEAD";
} else {
result.innerHTML = "You got TAIL";
}
}
button.addEventListener("click", fnClick);
</script>
</body>
Here’s a demo of the code above:
See the Pen Coin Flip Simulator by Nathan Sebhastian (@nathansebhastian) on CodePen.
Alternatively, you can also create a simulator that flips as many as x times following the user’s input. Let’s do that in the next section.
Create a coin flip simulator that flips x times
To create a coin flip simulator that flips as many times as the user wants, you need to create an HTML <input>
element to accept the user’s input as follows:
<body>
<div>
<label for="quantity">How many times to flip?</label>
<input type="number" id="quantity" min="1" value="1" />
</div>
<button id="flip" type="button">Flip the coin</button>
<div id="result"></div>
</body>
Next, revise the fnClick()
function to grab the value of the <input>
tag and use a for
statement to loop the coin flip code.
The result of the flip needs to be wrapped with a <p>
tag and stored in a variable (named score
in the example below)
Once you have all the results from the simulator, set it as the content of the <div>
element
Take a look at the fnClick()
function below:
<script>
let button = document.getElementById("flip");
let result = document.getElementById("result");
function fnClick(event) {
let qty = document.getElementById("quantity").value;
let score = "";
for (let i = 0; i < qty; i++) {
let num = Math.random();
if (num < 0.5) {
score += "<p>You got HEAD</p>";
} else {
score += "<p>You got TAIL</p>";
}
}
result.innerHTML = score;
}
button.addEventListener("click", fnClick);
</script>
Now you will have as many results as the number you put inside the <input>
element.
Check out the following demo:
See the Pen Coin flip with input JavaScript by Nathan Sebhastian (@nathansebhastian) on CodePen.
Finally, you can also create a coin flip simulator that shows a virtual coin using CSS. Let’s do that next.
Create a coin flip simulator with CSS
Note: the following coin flip simulator is adapted from a Codepen by Le Liu. I adjusted the code to remove redundant styling and give a fresh look
To create a coin flip simulator using CSS, you need to create three <div>
elements styled and animated using CSS.
First, create a new HTML <body>
tag with the following code:
<body>
<h1>Click on the coin below to flip</h1>
<div id="coin">
<div class="side head"></div>
<div class="side tail"></div>
</div>
</body>
The three <div>
elements represent a coin in the browser, with the parent <div>
coin
acts as the container of the head
and tail
side of the coin.
Next, write the CSS code that will style the coin as follows:
h1 {
text-align: center;
}
#coin {
position: relative;
margin: 0 auto;
width: 100px;
height: 100px;
cursor: pointer;
}
.side {
width: 100%;
height: 100%;
border-radius: 50%;
position: absolute;
backface-visibility: hidden;
}
.head {
background-color: yellow;
z-index: 10;
}
.tail {
background-color: red;
transform: rotateX(-180deg);
}
The #coin
style will put your visual coin at the center of the HTML page and set the width and height size to 100px
. You will also have the cursor transformed into a pointer to tell the user that it can be clicked.
The .side
style will transform the two <div>
elements that represent the sides of a coin to a circle by setting the border-radius
to 50%
on all sides. The backface-visibility
is set to hidden
to hide the back face while rotating.
The .head
and .tail
style each colors the side of the coin. The head side will have yellow
color while the tail side will have red
color.
The .head
class z-index
property is set to 10
to put it above the .tail
class.
The .tail
class will have the transform: rotateX()
property set to -180deg
so that it will synergize with
the rotation animation you will create next.
Now that the <div>
elements are styled as a visual coin, let’s create a CSS animation using @keyframes
Coin flip CSS animation with @keyframes
The @keyframes
rule is a CSS rule used to specify the animation code.
A coin flip animation can be achieved using the following rule:
@keyframes resultHead {
from {
transform: rotateX(0);
}
to {
transform: rotateX(1800deg);
}
}
@keyframes resultTail {
from {
transform: rotateX(0);
}
to {
transform: rotateX(1980deg);
}
}
the from
rule will set the initial condition of the animation. The code above sets the property transform
to rotateX(0)
so that the flip always initialized from the head side visible.
if the result of the flip is head, the coin will be flipped 1800deg
. This will create a flip animation five times because one flip is 360deg
.
When the flip result is tail, the coin will have rotating animation five times plus a 180 degree rotation to show the tail side to the user. This is why the .tail
CSS class sets the rotateX
value to -180
degree.
With the CSS animation rule specified, you can call them from CSS classes using the animation
property as follows:
.flipHead {
animation: resultHead 2s ease-out forwards;
}
.flipTail {
animation: resultTail 2s ease-out forwards;
}
The animation will be triggered when you add the flipHead
or flipTail
class to the <div id='coin'>
element. The animation will run with the following rules:
- The duration will be two seconds (
2s
) - with the transition getting slower when ending (
ease-out
) - and the style values when the animation ends will be saved (
forwards
)
With that, you have completed the CSS code for the simulator. Only the script to process the flip remains.
Coin flip simulator JavaScript code
To flip the coin on click, you need to add an event listener to <div id='coin'>
that will run the fnClick()
function.
Just like before, fnClick()
function will call Math.random()
method to determine the flip result. But instead of writing the result to an HTML element, it will set the className
property of the coin
<div>
:
let coin = document.getElementById("coin");
coin.addEventListener("click", fnClick);
function fnClick() {
var flipResult = Math.random();
if (flipResult < 0.5) {
coin.className = "flipHead";
} else {
coin.className = "flipTail";
}
}
And with that, your coin flip simulator is ready.
Here’s a demo of the simulator:
See the Pen Coin Flip simulator by Nathan Sebhastian (@nathansebhastian) on CodePen.
Feel free to use the code above and improve it.
Conclusion
You’ve just learned how to create a coin flip simulator using plain JavaScript and HTML. To add a visual coin flip animation, you need to learn how to style and animate elements using CSS.
Thanks for reading and I hope you’ve learned something new from this tutorial 😉