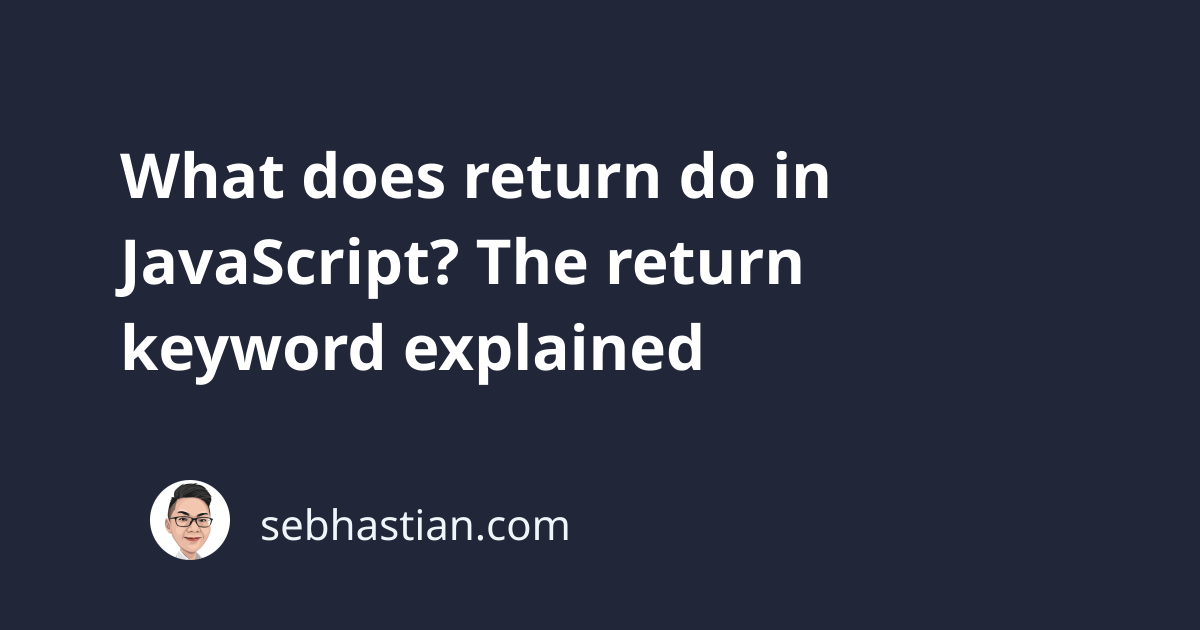
The return
keyword in JavaScript is a keyword to build a statement that ends JavaScript code execution. For example, suppose you have the following code that runs two console logs:
console.log("Hello");
return;
console.log("How are you?");
You’ll see that only “Hello” is printed to the console, while “How are you?” is not printed because of the return
keyword. In short, the return
statement will always stop any execution in the current JavaScript context, and return control to the higher context. When you use return
inside of a function
, the function
will stop running and give back control to the caller.
The following function won’t print “Hi from function” to the console because the return
statement is executed before the call to log the string. JavaScript will then give back control to the caller and continue code execution from there:
function fnExample() {
return;
console.log("Hi from function");
}
fnExample(); // nothing will be printed
console.log("JavaScript");
console.log("Continue to execute after return");
You can add an expression to the return
statement so that instead of returning undefined
, the value that you specify will be returned.
Take a look at the following code example, where the fnOne()
function just return
without any value, while fnTwo()
returns a number 7
:
function fnOne() {
return;
}
function fnTwo() {
return 7;
}
let one = fnOne();
let two = fnTwo();
console.log(one); // undefined
console.log(two); // 7
In the code above, the values returned from the return
statement by each function are assigned to variables one
and two
. When return
statement will give back undefined
by default.
You can also assign a variable to the return
statement as follows:
function fnExample() {
let str = "Hello";
return str;
}
let response = fnExample();
console.log(response); // Hello
And that’s all about the return
keyword in JavaScript. You can combine the return
statement and conditional if..else
block to control what value returned from your function
.
In the following example, the function will return “Banana” when number === 1
else it will return “Melon”:
function fnFruit(number) {
if (number === 1) {
return "Banana";
} else {
return "Melon";
}
}
let fruit = fnFruit(1);
console.log(fruit); // "Banana"