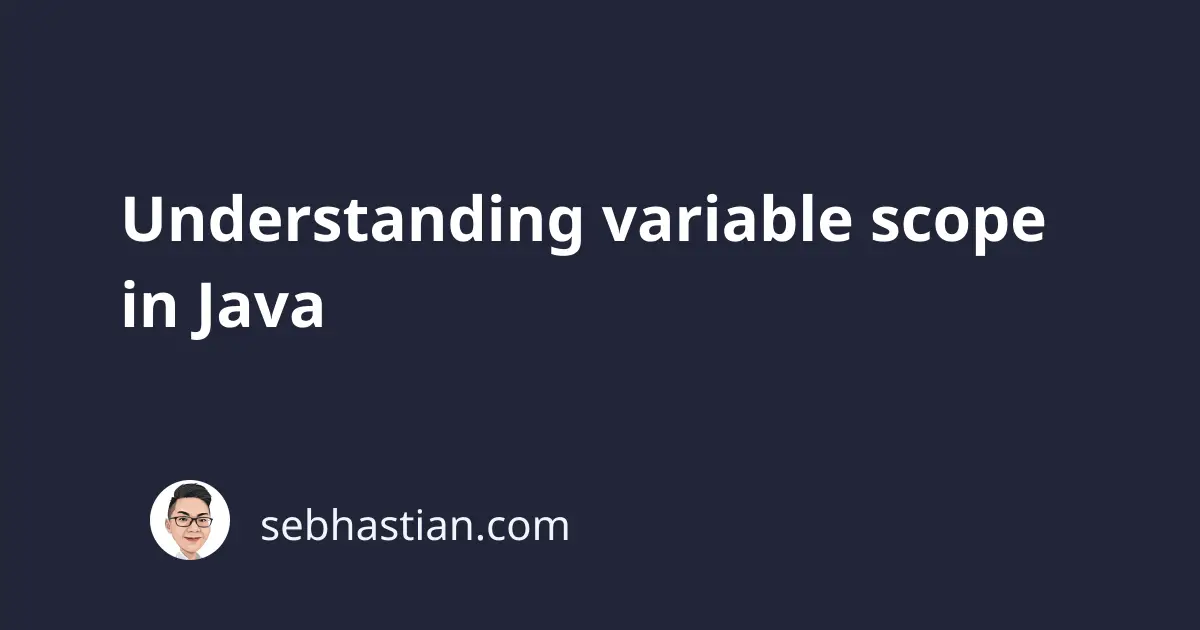
Each variable you used in your Java application will have a different variable scope depending on where you created that variable.
The variable scope then determines from where you can access your variable.
This tutorial will help you to understand the three different variable scopes in Java:
- class-level scope
- method-level scope
- block-level scope
Let’s start with learning the class-level scope.
Class level variable scope
The variables that you declared inside a class but outside of its methods are called instance variables. These variables the class-level scope and can be accessed by methods of the class.
For example, suppose you have a Cat
class with name
variable initialized inside:
public class Cat {
String name = "cat";
}
Then the methods inside the class can call on the name
variable and assign a new value to it.
Take a look at the following example:
public class Cat {
String name = "cat";
public void hello(){
System.out.println(name + " says hello!");
}
public void setName(String newName){
name = newName;
}
}
Both methods above are valid and will use the name
variable you initialized below the class
declaration in their execution.
Variables in Java must also be declared or initialized before subsequent calls to use or re-assign the variable value:
public class Cat {
System.out.println(name + " says hello!");
// or
name = "Tom";
// both cause error: Can't resolve symbol name
String name = "cat";
}
Now that you know about class-level variable scope, let’s continue to learn about method-level variable scope.
Method level variable scope
The variables you declared or initialized inside a method are called local variables, and they will have method-level scope.
It means these variables will be accessible only inside the method you created them.
Suppose you have the following size()
method inside the Cat
class:
public class Cat {
String name = "cat";
public void size(){
double length = 1.5;
System.out.println("Body length is "+ length);
}
}
Then the length
variable, which is declared inside the size()
method won’t be accessible anywhere outside the method.
Suppose you have a second method called sleep()
that will increase the cat’s length to 2
as follows:
public class Cat {
String name = "cat";
public void size(){
double length = 1.5;
System.out.println("Body length is "+ length);
}
public void sleep(){
length = 2;
// ^ error: Can't resolve symbol length
}
}
The length
variable assignment inside sleep()
method above will cause an error. You need to either declare the variable again inside the sleep()
method:
public class Cat {
String name = "cat";
public void size(){
double length = 1.5;
System.out.println("Body length is "+ length);
}
public void sleep(){
double length = 1.5;
length = 2;
}
}
Or declare the length
variable inside the class instead of the methods:
public class Cat {
String name = "cat";
double length = 1.5;
public void size(){
System.out.println("Body length is "+ length);
}
public void sleep(){
length = 2;
}
}
Block level variable scope
The variables you declared inside a certain bracket {}
will have the block-level scope and can only be accessed inside that brackets.
For example, you may see a block-level variable when you write a for
loop statement:
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
The Integer i
above can’t be accessed outside of the for
loop.
In Java, you can declare an additional block anytime by creating another bracket inside a method or a class. The variables created inside the bracket will then have block level scope.
Here’s an example of creating a block inside a method:
public class Cat {
public void changeName(){
{
String name = "cat";
}
name = "Tom";
// ^ error: Can't resolve symbol length
}
}
If you consider the previous scopes carefully, you may start to notice that Java variables declared in one block scope are accessible from the inner scope but not from the outer scope.
For example, a variable created in the class block can be accessed by the inner method block:
public class Cat {
String name = "cat";
public void hello(){
System.out.println(name + " says hello!");
}
}
But a method variable can’t be accessed by the outer class block:
public class Cat {
public void hello(){
String name = "cat";
}
name = "Tom"
}
When you remove the class and method keywords from the screen, you’ll see that both class and method level scopes just use brackets {}
to change the variable scope:
{ // public class Cat
{ // public void hello()
String name = "cat";
}
name = "Tom"
}
This means both class level and method level scopes are actually block scopes too.
Conclusion
Now that you’ve learned about variable scopes in Java, it’s time to learn about Java access modifier which determines whether you can access certain variables from outside of its package or not.