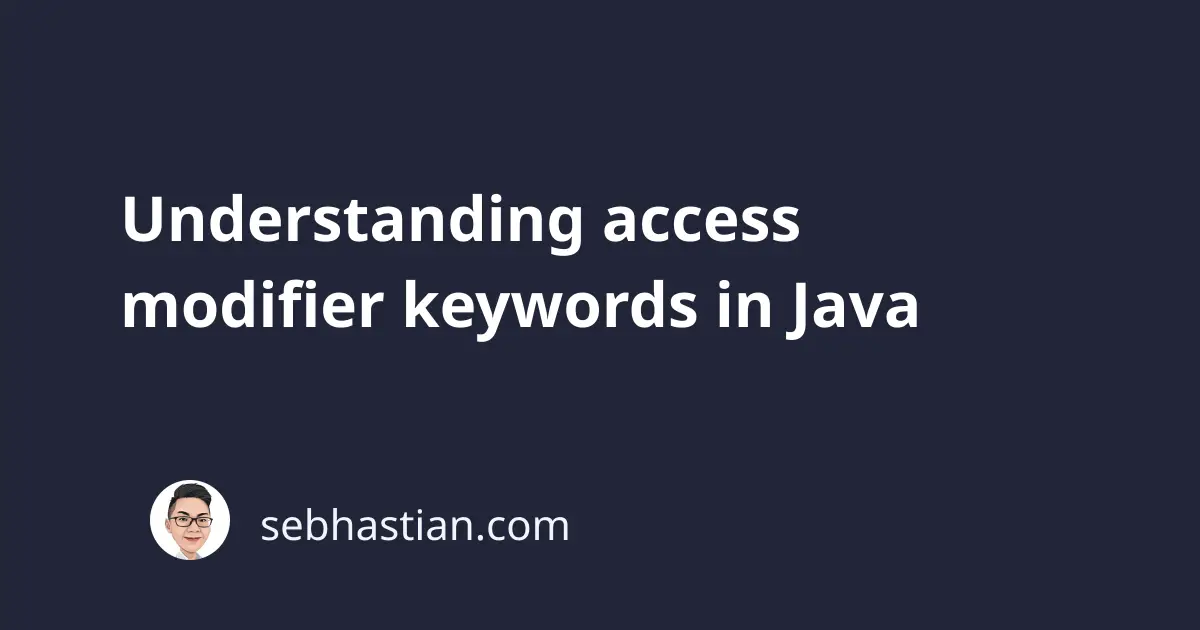
Access modifiers are keywords that determine the accessibility of Java language members like classes, constructors, methods, or variables.
There are four types of access modifier keywords in Java:
- Default (no keyword required)
private
protected
public
Except for the default modifier, all other modifiers are usually declared before the class, method, or variable itself.
Declaring access modifiers
The code below shows how to declare a class as public
level access:
public class Cat {}
And the following shows how to declare a protected
method:
public class Cat {
protected void hello(){
System.out.println("Meow!");
}
}
The same goes for variables:
protected String name = "Cat";
// or
private int age = 5;
Finally, here’s how to declare a constructor as protected
:
public class Cat {
String name;
protected Cat(){
name = "Cat";
}
}
Without the access modifier type declared, your classes, methods, and variables will have the default access:
String name = "Cat";
int age = 5;
The access modifier keywords determine the accessibility of Java language members.
Here’s a table showing the differences between these keywords:
Modifier | Same Class | Same Package | Subclasses/ instances | World |
---|---|---|---|---|
Default/ no modifier | Y | Y | N | N |
private | Y | N | N | N |
protected | Y | Y | Y | N |
public | Y | Y | Y | Y |
Let’s see some explanations about these keywords so you can understand them better, starting from the default access level
Java default access modifier
The default level access allows Java class and its members to be accessed from the same class and package, but inaccessible from a different package.
For example, suppose you have a Cat.java
file located in the animal
package as follows:
package animal;
class Cat {
void hello() {
System.out.println("Mew!");
}
}
You can access the Cat
class from other classes in the same package without importing as shown below:
package animal;
public class SiameseCat extends Cat {}
But you won’t be able to access the Cat
class from package main
even after you import
the class as follows:
package main;
import animal.Cat;
// ^ Error animal.Cat is not public
public class Main {
public static void main(String[] args) {
Cat myCat = new Cat();
}
}
To make the Cat
class available outside of the animal
package, you need to make the class public
like this:
public class Cat {}
Java private access modifier
The private
keyword in Java is used to make variables and methods of a Java
class to be inaccessible outside of the class itself.
The private
modifier is not commonly used for a class, since a private
class would be useless as nothing can access it.
For example, the following name
variable and hello()
method inside the Cat
class are declared as private:
public class Cat {
private String name = "Tom";
private void hello() {
System.out.println("Meow!");
}
}
Both members won’t be accessible in a new object instance as shown below:
public static void main(String[] args) {
Cat myCat = new Cat();
myCat.name = "The Cat";
// Error: name has private access
myCat.hello();
// Error: hello() has private access
}
To make the variables and methods of a class accessible from its instances, then you need to use the protected
modifier.
Java protected access modifier
Java protected
access modifier allows you to access class members from the class itself or another class of the same package.
The following example shows how the Cat
class members are accessible from the Main
class in the same package:
package com.company;
class Cat {
protected String name = "Tom";
protected void hello() {
System.out.println("Meow!");
}
}
public class Main {
public static void main(String[] args) {
Cat myCat = new Cat();
myCat.name = "Thomas";
myCat.hello();
}
}
The protected
members of a class can also be accessed by its subclass even from a different package.
The code below shows how the SiameseCat
object instance can access variables and methods from the Cat
class:
import animal.Cat;
public class SiameseCat extends Cat {
public static void main(String[] args) {
SiameseCat myCat = new SiameseCat();
myCat.name = "Siam";
myCat.hello();
}
}
Java public access modifier
The Java public
access modifier removes all restrictions for accessing classes, constructors, methods, and keywords. It allows you to access the Java class and its members from anywhere inside the same program.
The public
keyword is usually reserved for constant variables in Java:
public static final score = 10;
You may see the public static final
modifier applied to many of the Math
class members.
Now that you’ve learned about access modifier keywords in Java, it’s time for you to learn about the non-access modifier keywords next.