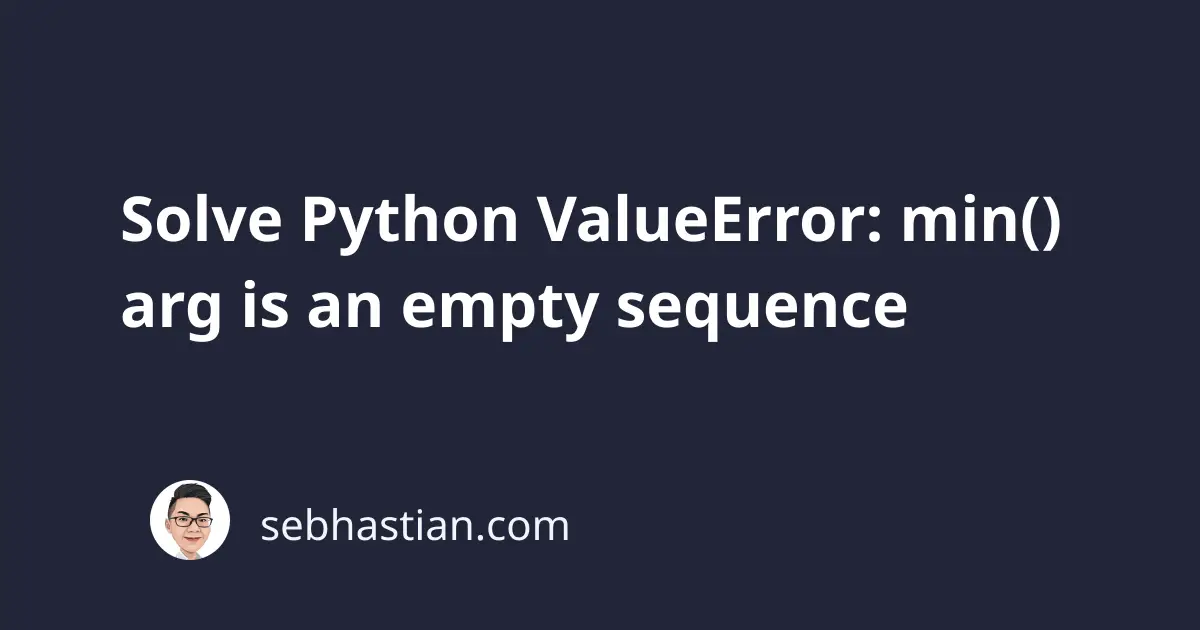
The “ValueError: min() arg is an empty sequence” error in Python occurs when you try to call the min()
function on an empty sequence, such as a list or tuple.
To solve this error, you need to make sure that you pass a non-empty sequence or a default value. This article shows you how it works.
The min()
function is a built-in function in Python that returns the smallest element in a sequence as shown below:
list = [8, 4, 10, 2]
result = min(list)
print(result) # 2
However, if you try to call min()
on an empty sequence, the function has nothing to compare, and Python will raise a “ValueError: min() arg is an empty sequence” to notify you of this issue.
The following code:
list = []
# ❗️ValueError: min() arg is an empty sequence
result = min(list)
Will produce the output below:
To fix this error, you need to make sure that the sequence passed to min()
is not empty.
You can do this by checking the length of the sequence before calling min()
, or by adding the default
argument for the function when the sequence is empty.
See the example below:
# example 1: pass empty list with default value
list = []
result = min(list, default=0)
print(result) # 0
# example 2: pass a list with default value
list = [7, 9, 10, 5]
result = min(list, default=0)
print(result) # 5
When you pass a default
argument, Python will assign the default
value when the list is empty.
You can pass any type of data as the default
argument, like a string or another list:
list = []
# pass a list as the default value
result = min(list, default=[1, 2, 3])
print(result) # [1, 2, 3]
Alternatively, you can also use an if .. else
conditional and call the min()
function only when the list
is not empty.
When the len()
of the list is greater than 0
, call the min()
function as shown below:
list = []
if len(list) > 0:
result = min(list)
else:
result = 9
The code above will assign the number 9
as the value of the result
variable when the list
is empty.
Finally, you can also add the try .. except
block like this:
list = []
try:
result = min(list)
except ValueError:
print("An error occurred")
result = 9
print(result) # 9
The except
block above will be run whenever the code in the try
block raises a ValueError
. This keeps the code running since Python knows what to do when the error happens.
You are free to continue the code above to fit your project.
Conclusion
The Python “ValueError: min() arg is an empty sequence” error is raised when you pass an empty list as an argument to the min()
function.
To solve this error, you need to make sure that the list argument has at least one valid element. You can also pass a default
argument to the function that will be used as the default value should the sequence is empty.
Now you’ve seen how to fix this error like a real Python pro! 👍
Keep in mind that the min()
function may raise other errors if the elements of the sequence are not comparable.
For example, if the sequence contains elements of different types, such as strings and integers, the function will raise a TypeError: < not supported between instances of int and str error.
Besides the min()
function, the max()
function also has the ValueError exception with the same solution.