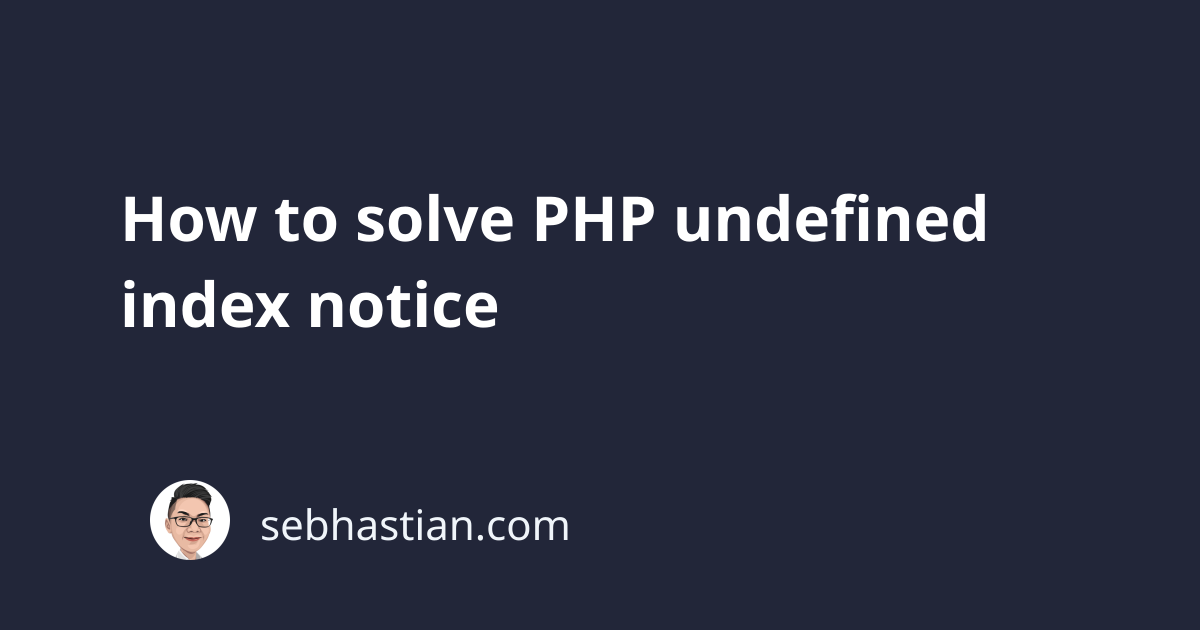
When accessing an array in PHP, you may see a notice message that says “undefined index” as follows:
<?php
$my_arr = [
"name" => "Nathan",
];
echo $my_arr["age"];
// Notice: Undefined index: age on line 7
When accessing the PHP page from the browser, the following notice message appears:
The Notice: Undefined index
message means that the index key you use to access an array’s value doesn’t exist.
In the above example, the array $my_arr
only has the name
index key. The age
index is undefined.
To solve this notice message, you need to make sure that the index is defined inside the array.
The easiest way to do so is by calling the isset()
function on the array index before accessing it.
Consider the example below:
<?php
$my_arr = [
"name" => "Nathan",
];
// 👇 call isset to check for the age value
if (isset($my_arr["age"])) {
echo $my_arr["age"];
} else {
echo "age is not defined in the array!";
}
The isset()
function in PHP will make sure that the age
index is defined in $my_arr
.
Combine it with an if
condition to create a conditional block that runs when the array index is defined.
Alternatively, you can also use the null coalescing operator to provide a fallback value when the index is undefined like this:
<?php
$my_arr = [
"name" => "Nathan",
];
echo $my_arr["age"] ?? 29;
// 👆 prints 29
Because the age
index is undefined in the example above, the integer 29
is will be printed by the echo
function.
The null coalescing operator is useful when you have a fallback value in case the first value is undefined.
Both isset
and null coalescing operator also works when you are accessing form values with $_GET
and $_POST
global variable.
Suppose you’re accessing a PHP page with the following GET
parameters:
index.php?name=nathan&age=22
The following code checks if you have a GET
parameter named age
:
if (isset($_GET["age"])) {
echo "I am {$_GET["age"]} years old.";
} else {
echo "age is not defined in the array!";
}
Or if you want to use the null coalescing operator:
// 👇 place the value in a variable
$age = $_GET["age"] ?? 27;
// 👇 call the variable inside echo
echo "I am $age years old.";
You can use the same methods with the $_POST
variable.
Remove the undefined index notice
While the notice message is useful, you may want to turn it off in production environment so your visitors won’t see it.
When you don’t want the undefined index notice to appear, you can remove it by setting the error_reporting()
function at the top of your PHP page.
Set it as follows:
<?php
error_reporting(E_ALL ^ E_NOTICE);
Now your PHP compiler will not show any notice messages, but other error messages will still be shown.
The error_reporting()
function works only for the page where you call the function.
To make it work for all of your PHP pages, you need to set the configuration in your php.ini
file as follows:
error_reporting = E_ALL & ~E_NOTICE
By setting the configuration in php.ini
file, all pages will not show notice messages.
Conclusion
Now you’ve learned what is an undefined index notice in PHP and how to resolve the message.
You can use the isset()
function or null coalescing operator to deal with an undefined index in PHP.
You can also remove the notice message by setting the error_reporting
parameter in php.ini
file, or calling the error_reporting()
function.