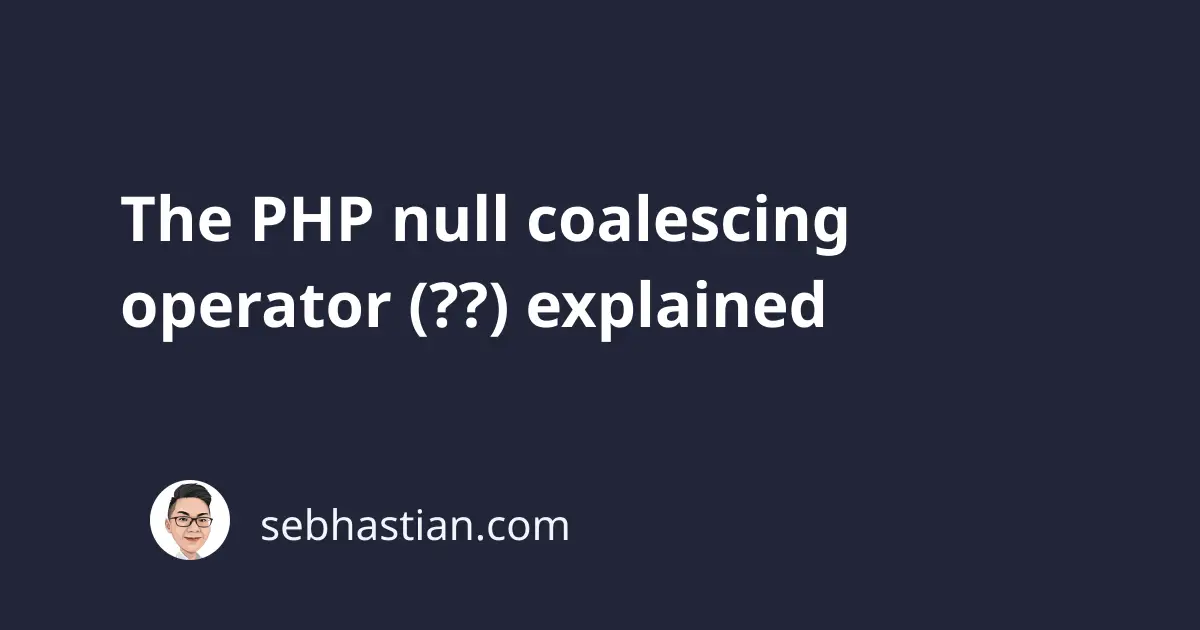
The PHP null coalescing operator (also known as the double question mark ??
operator) is a new feature introduced in PHP 7.
The operator has two operands as follows:
first operand ?? second operand;
The operator returns the first operand when the value is not null. Otherwise, it returns the second operand.
The operator allows you to set an if condition as shown below:
// 👇 PHP null coalescing operator common case
$username = $_GET['user'] ?? 'nathan';
If the $_GET['user']
value is null
in the example above, then assign the value nathan
into the $user
variable.
The null coalescing operator allows you to shorten a null
check syntax that you need to do with the isset()
function.
Before null coalescing operator, you may check for a null
value using the ternary operator in conjunction with the isset()
function like this:
// 👇 example of checking null using isset()
// and ternary operator
$user = isset($_POST['user']) ? $_POST['user']: 'nathan';
When the user
value is “unset” or null
, assign nathan
as the value of $user
variable. Otherwise, it will assign $_POST['user']
as its value.
Stacking the null coalescing operands in PHP
The null coalescing operator allows you to stack as many operands as you need.
The operator will go from left to right looking for a non-null value:
$user = $username ?? $first_name ?? $last_name ?? 'nathan';
echo $user; // 'nathan';
When the value of $username
is null
or not set, PHP will look for the value of $first_name
.
When $first_name
is null
or not set, PHP will look for the value of $last_name
variable.
When $last_name
variable is also null
or not set, PHP will assign nathan
as the value of $user
variable.
Null coalescing assignment operator in PHP
The null coalescing operator can also be used to check a variable if it’s null.
You can then assign a value to the variable itself like this:
$user = null;
$user ??= 'nathan';
echo $user; // 'nathan';
The syntax above means that PHP will check for the value of $user
.
When $user
is null or not set, then the value nathan
will be assigned to it.
The code above is equivalent to the following isset()
check:
$user = null;
if (!isset($user)) $user = 'nathan';
echo $user; // 'nathan';
And that’s how you assign a variable value using the null coalescing operator.
Conclusion
The PHP null coalescing operator is a new syntax introduced in PHP 7.
The operator returns the first operand if it exists and not null
. Otherwise, it returns the second operand.
It’s a nice syntactic sugar that allows you to shorten the code for checking variable values using the isset()
function.
Now you’ve learned how the null coalescing operator works in PHP. Nice work!